How to Detect EOF in Java
-
Detecting
EOF
in Java Using theScanner.hasNext()
Method -
Detecting
EOF
in Java Using theInputStream.read()
Method -
Detecting
EOF
in Java Using theBufferedReader.readLine()
Method -
Detecting
EOF
in Java UsingFileChannel
andByteBuffer
- Conclusion
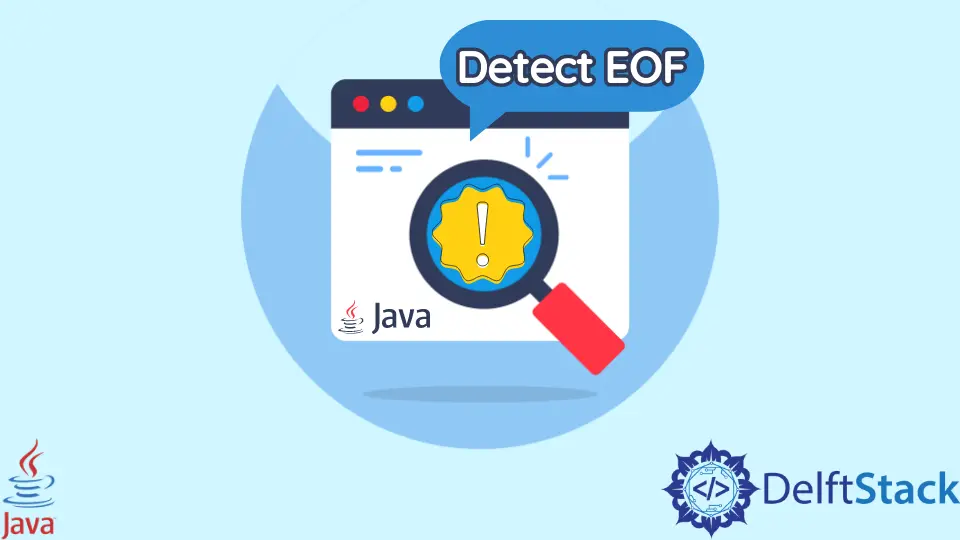
In this tutorial, we’ll introduce how to detect EOF
(End OF File) using different methods in Java. We’ll also discuss developing a program that continues reading content until the end of the file is reached.
From the programming standpoint, the EOF
is a specialized type that developers use to continually read input, whether from the file or keyboard console. This process continues until there is no more data to retrieve.
Detecting EOF
in Java Using the Scanner.hasNext()
Method
One of the methods that provide an elegant and convenient solution is the Scanner.hasNext()
method. This method belongs to the Scanner
class, a powerful tool for parsing input.
Detecting EOF
is essential because it allows a program to gracefully exit a loop or terminate file reading operations when there is no more data to process. In this section, we will explore how to leverage the Scanner.hasNext()
method for EOF
detection in Java.
Let’s consider a simple scenario where we have a text file named example.txt
containing a list of words. Our goal is to read each word from the file until the end is reached, using the Scanner.hasNext()
method.
Below is the complete Java code demonstrating this concept:
import java.io.File;
import java.io.FileNotFoundException;
import java.util.Scanner;
public class EOFDetectionExample {
public static void main(String[] args) {
// Specify the file path
String filePath = "example.txt";
try {
// Create a Scanner object for the file
Scanner scanner = new Scanner(new File(filePath));
// Loop until the end of the file
while (scanner.hasNext()) {
// Read the next word from the file
String word = scanner.next();
// Process the word (In this example, we simply print it)
System.out.println("Word: " + word);
}
// Close the scanner to release resources
scanner.close();
} catch (FileNotFoundException e) {
System.err.println("File not found: " + filePath);
e.printStackTrace();
}
}
}
We start by importing the necessary classes, including File
and Scanner
, to handle file operations and input parsing.
The main
method is the entry point of our program. Here, we specify the path of the file we want to read (example.txt
).
We encapsulate file-related operations in a try-catch
block to handle the possibility of a FileNotFoundException
.
We create a Scanner
object and associate it with the specified file. This Scanner
will be our tool for reading from the file.
Using a while
loop, we continuously check for the presence of the next token (word
) in the file using scanner.hasNext()
.
Within the loop, we use scanner.next()
to retrieve the next word from the file. In this example, we simply print each word, but you can customize this part to suit your specific processing needs.
It’s crucial to close the Scanner
using the close()
method to release system resources when we are done reading the file.
Assuming the example.txt
file contains the words Java
, Scanner
, and EOF
, the output of the program would be as follows:
Word: Java,
Word: Scanner,
Word: EOF
This output demonstrates that the program successfully detects and processes each word in the file until reaching the end of the file.
Detecting EOF
in Java Using the InputStream.read()
Method
Reading from files or streams in Java often involves the need to detect the end of the file (EOF
) to gracefully terminate the reading process. One fundamental method for achieving this is by using the InputStream.read()
method.
This method is part of the InputStream
class, providing a way to read bytes from an input stream.
In this example, we’ll create a simple Java program that reads a text file named example.txt
and prints its content using the InputStream.read()
method.
import java.io.FileInputStream;
import java.io.IOException;
import java.io.InputStream;
public class EOFDetectionExample {
public static void main(String[] args) {
// Specify the file path
String filePath = "example.txt";
try {
// Create an InputStream for the file
InputStream inputStream = new FileInputStream(filePath);
// Read and print each byte until the end of the file is reached
int data;
while ((data = inputStream.read()) != -1) {
// Convert byte to character and print
System.out.print((char) data);
}
// Close the InputStream to release resources
inputStream.close();
} catch (IOException e) {
System.err.println("Error reading file: " + e.getMessage());
e.printStackTrace();
}
}
}
The code begins by importing the necessary classes, including FileInputStream
and InputStream
, to handle file input operations.
The main
method serves as the entry point for our program. Here, we specify the path of the file we want to read (example.txt
).
File-related operations are enclosed within a try-catch
block to handle potential IOExceptions
. We create an InputStream
and associate it with the specified file using FileInputStream
.
The while
loop continuously reads bytes from the file using inputStream.read()
. The loop continues until read()
returns -1
, indicating the end of the file.
Within the loop, each byte read is cast to a character using (char) data
and then printed. This conversion is necessary to interpret the bytes as characters.
It’s crucial to close the InputStream
using close()
to release system resources when done reading the file.
Assuming the example.txt
file contains the text Hello, World!
, the output of the program would be:
Hello, World!
This output demonstrates the successful detection of EOF
using the InputStream.read()
method. The program reads each byte from the file and prints it until the end of the file is reached, providing an effective way to handle EOF
scenarios during file reading in Java.
Detecting EOF
in Java Using the BufferedReader.readLine()
Method
When working with file input operations in Java, it’s crucial to efficiently handle the end of a file (EOF
). One of the powerful tools for this task is the BufferedReader.readLine()
method.
This method, part of the BufferedReader
class, enables reading lines of text from a file until the end is reached.
Let’s explore a practical example that demonstrates the use of BufferedReader.readLine()
to read a text file named example.txt
and print its content.
import java.io.BufferedReader;
import java.io.FileReader;
import java.io.IOException;
public class EOFDetectionExample {
public static void main(String[] args) {
// Specify the file path
String filePath = "example.txt";
try {
// Create a BufferedReader for the file
BufferedReader reader = new BufferedReader(new FileReader(filePath));
// Read and print each line until the end of the file is reached
String line;
while ((line = reader.readLine()) != null) {
// Process each line (In this example, we simply print it)
System.out.println("Line: " + line);
}
// Close the BufferedReader to release resources
reader.close();
} catch (IOException e) {
System.err.println("Error reading file: " + e.getMessage());
e.printStackTrace();
}
}
}
The code begins by importing the necessary classes, including BufferedReader
, FileReader
, and IOException
, to handle file input operations and exceptions.
The main
method is the starting point for our program. Here, we specify the path of the file we want to read (example.txt
).
File-related operations are encapsulated within a try-catch
block to handle potential IOExceptions
that may occur during file processing.
We create a BufferedReader
and associate it with the specified file using FileReader
. This buffered reader allows us to efficiently read lines of text from the file.
The while
loop continuously reads lines from the file using reader.readLine()
. The loop continues until readLine()
returns null
, indicating the end of the file.
Within the loop, each line read is processed. In this example, we simply print each line, but you can customize this part to suit your specific processing needs.
It’s essential to close the BufferedReader
using close()
to release system resources when we are done reading the file.
Assuming the example.txt
file contains the lines Hello, World!
and Java is powerful
, the output of the program would be:
Line: Hello, World!
Line: Java is powerful
This output demonstrates the successful detection of EOF
using the BufferedReader.readLine()
method. The program reads each line from the file and processes it until the end of the file is reached, showcasing an effective approach to handle EOF
scenarios during file reading in Java.
Detecting EOF
in Java Using FileChannel
and ByteBuffer
While there are various methods available, leveraging the FileChannel
and ByteBuffer
classes provides a more low-level but powerful approach. The FileChannel
class, part of the java.nio
package, allows for more direct interaction with a file, and when combined with ByteBuffer
, it becomes a versatile tool for reading data efficiently.
In this section, we’ll explore how to use FileChannel
and ByteBuffer
to detect EOF
in Java.
Let’s delve into a comprehensive example that showcases the use of FileChannel
and ByteBuffer
to read a file named example.txt
and print its content.
import java.io.RandomAccessFile;
import java.nio.ByteBuffer;
import java.nio.channels.FileChannel;
public class EOFDetectionExample {
public static void main(String[] args) {
// Specify the file path
String filePath = "example.txt";
try {
// Create a RandomAccessFile with read-only mode
RandomAccessFile file = new RandomAccessFile(filePath, "r");
// Get the FileChannel from the RandomAccessFile
FileChannel channel = file.getChannel();
// Create a ByteBuffer with a specified capacity
ByteBuffer buffer = ByteBuffer.allocate(1024);
// Read and print data until the end of the file is reached
while (channel.read(buffer) != -1) {
// Flip the buffer to read its contents
buffer.flip();
// Process the data (In this example, we print it)
while (buffer.hasRemaining()) {
System.out.print((char) buffer.get());
}
// Clear the buffer for the next read
buffer.clear();
}
// Close the FileChannel and RandomAccessFile to release resources
channel.close();
file.close();
} catch (Exception e) {
System.err.println("Error reading file: " + e.getMessage());
e.printStackTrace();
}
}
}
We begin by importing the necessary classes, including RandomAccessFile
, FileChannel
, and ByteBuffer
, to handle file input operations and buffer management.
The main
method serves as the entry point for our program. Here, we specify the path of the file we want to read (example.txt
).
File-related operations are encapsulated within a try-catch
block to handle potential exceptions. We create a RandomAccessFile
with read-only mode, providing us with a flexible file access mechanism.
The getChannel()
method is used to obtain the FileChannel
associated with the RandomAccessFile
. This channel allows for low-level operations on the file.
We create a ByteBuffer
with a specified capacity. This buffer serves as a temporary storage for the data read from the file.
The while
loop reads data from the file into the ByteBuffer
until channel.read(buffer)
returns -1
, indicating the end of the file.
We flip the buffer using buffer.flip()
to prepare it for reading. The inner loop processes the data, printing each character in this example.
After processing the data, we clear the buffer using buffer.clear()
to make it ready for the next read operation. Finally, we close the FileChannel
and RandomAccessFile
to release system resources.
Assuming the example.txt
file contains the text Hello, FileChannel and ByteBuffer!
, the output of the program would be:
Hello, FileChannel and ByteBuffer!
This output demonstrates the successful detection of EOF
using the FileChannel
and the ByteBuffer
method. The program efficiently reads and processes data from the file until the end is reached, highlighting the effectiveness of this low-level approach for EOF
detection in Java file handling.
Conclusion
Detecting EOF
is vital for effective file handling. The Scanner.hasNext()
method offers simplicity, ideal for tokenized data.
Alternatively, InputStream.read()
provides low-level byte control, suitable for binary data. BufferedReader.readLine()
strikes a balance, allowing line-by-line processing.
The FileChannel
and ByteBuffer
combination offers an efficient, low-level approach for handling large datasets. Each method caters to distinct needs, providing developers with versatile tools for EOF
detection in Java file operations.
The choice depends on the task’s specific requirements, whether simplicity, granular control, or optimal performance is paramount. Java’s flexibility accommodates a variety of file-handling scenarios.