Java에서 EOF 감지
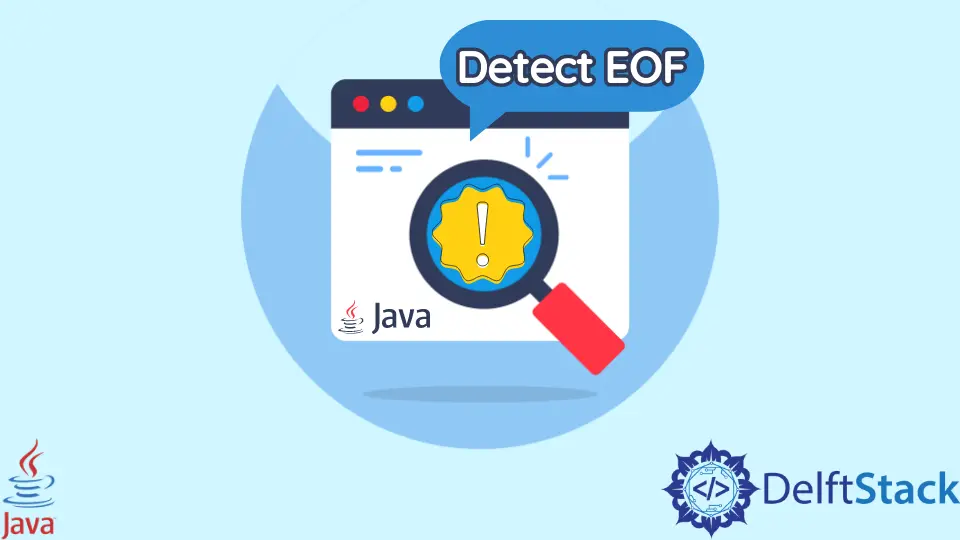
이 튜토리얼에서는 Java에서 while
루프를 사용하여 EOF (End OF File
)를 감지하는 방법을 소개합니다. 또한 파일 끝에 도달할 때까지 콘텐츠를 계속 읽는 프로그램 개발에 대해 논의할 것입니다.
프로그래밍 관점에서 EOF는 개발자가 파일 또는 키보드 콘솔에서 입력을 지속적으로 읽는 데 사용하는 특수 유형입니다. 이 프로세스는 검색할 데이터가 더 이상 없을 때까지 계속됩니다.
여기서 파일의 데이터는 언제든지 수정되거나 변경될 수 있다는 점을 언급할 가치가 있습니다. 따라서 해당 파일에 감시 제어 루프를 적용하는 것은 사실상 불가능합니다.
이러한 이유로 위에서 언급한 문제의 잠재적 위협을 완화하기 위해 항상 파일 끝 루프를 사용하는 것이 좋습니다.
아래 코드 예제에서는 키보드를 입력 소스로 사용합니다.
package sampleProject;
import java.util.*;
import java.util.Scanner;
public class Codesample {
static Scanner console = new Scanner(System.in);
public static void main(String[] args) {
int total_ages = 0;
int age;
while (console.hasNext()) {
age = console.nextInt();
total_ages = total_ages + age;
}
System.out.println("Total ages are:" + total\_ages);
}
}
출력:
99
90
88
Ko
Exception in thread "main" java.util.InputMismatchException
at java.base/java.util.Scanner.throwFor(Scanner.java:939)
at java.base/java.util.Scanner.next(Scanner.java:1594)
at java.base/java.util.Scanner.nextInt(Scanner.java:2258)
at java.base/java.util.Scanner.nextInt(Scanner.java:2212)
at sampleProject/sampleProject.Codesample.main(Codesample.java:15)
스캐너 클래스의 객체를 선언하고 초기화하는 것으로 시작합니다. 그런 다음 코드에서 사용할 다른 변수를 선언했습니다.
루프 반복 조건으로 console.hasNext()
를 사용하는 것을 보셨을 것입니다. 콘솔이 입력 클래스 스캐너의 객체라는 것을 이미 알고 있습니다.
반면 hasNext()
는 입력 클래스 Scanner에 미리 정의된 메서드입니다. 이 표현식은 정수 입력이 있는 경우에만 true를 반환합니다. 그렇지 않으면 false를 반환합니다.
이 코드를 직접 컴파일하고 출력을 확인하십시오. 잘못된 입력 유형을 제공할 때까지 프로그램이 계속 데이터를 읽는다는 것은 출력에서 매우 분명합니다. 이 경우 이 프로그램은 입력 불일치 예외를 발생시킵니다.
입력 소스로 파일
다른 예에서 파일 끝에서 제어되는 while 루프를 사용하여 파일에서 데이터를 지속적으로 읽습니다.
Students\_Result.txt
파일에서 모든 데이터를 읽고 싶다고 가정해 보겠습니다. 파일에는 학생 이름과 시험 점수가 포함되어 있습니다.
이 프로그램의 목표는 학생의 이름, 시험 점수 및 성적을 표시하는 파일을 출력하는 것입니다.
package sampleProject;
import java.io.FileNotFoundException;
import java.io.FileReader;
import java.io.PrintWriter;
import java.util.*;
import java.util.Scanner;
public class Codesample {
static Scanner console = new Scanner(System.in);
public static void main(String[] args) throws FileNotFoundException {
// declare file name as an input object
Scanner inputFile = **new** Scanner(**new** FileReader("Students\_Result.txt"));
// declare the output object that stores data we want to display
PrintWriter outputFile = **new** PrintWriter("Students\_Record.txt");
// Declare variable to store first name and last name
String firstName, lastName;
// declare variable to store score
double score;
// declare variable to store student's grad
char grade = ' ';
// declare and initialize counter variable
int counter = 0;
// continue reading data from the file until the loop reaches end of file
while (inputFile.hasNext()) {
// read first name from the provided file
firstName = inputFile.next();
// read last name from the file
lastName = inputFile.next();
// read score of the students from the filee
score = inputFile.nextDouble();
// increment counter variable
counter++;
// To evaluate grade of the students, we are using the switch statemet
switch ((int) score / 10) {
case 0:
case 1:
case 2:
case 3:
case 4:
case 5:
grade = 'F';
break;
case 6:
grade = 'D';
break;
case 7:
grade = 'C';
break;
case 8:
grade = 'B';
break;
case 9:
case 10:
grade = 'A';
break;
default:
outputFile.println("Your score does not meet our criteria");
}
// Display retrieved data using the outputFile object we have declared earlier
outputFile.println(firstName + " " + lastName + " " + score + " " + grade);
}
// If no data is found in the output file
if (counter == 0)
outputFile.println("There is not data in your input file");
// close output file upon reading all the data from it.
outputFile.close();
}
}
출력:
Waleed Ahmed 89.5 B
Bob Alice 90.0 A
Khan Arshad 100.0 A
Waqas Jameed 65.5 D
Danish Taimoor 70.5 C
Muaz Junaid 80.5 B
John Harris 88.0 B
시스템에서 이 프로그램을 컴파일하고 실행하여 무슨 일이 일어나는지 확인하십시오.