How to Declare a Char Array in Java
-
Declare a
char
Array Using thetoCharArray
Function in Java -
Declare a
char
Array Using thenew
Keyword in Java -
Declare and Initialize a
char
Array Using thenew
Keyword in Java
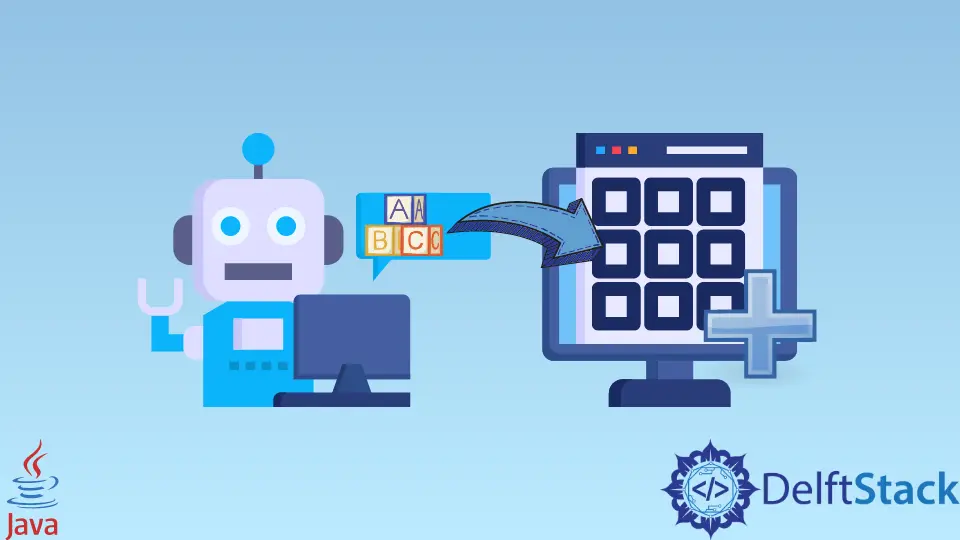
The term Character Array in Java represents the sequence of characters that binds together to form a string. The array has various functionalities that help in character operations.
Declare a char
Array Using the toCharArray
Function in Java
package character_manipulation;
public class DeclareCharArray {
public static void main(String[] args) {
String s1 = "First String";
char[] charArray = s1.toCharArray();
for (char c : charArray) {
System.out.print(" " + c);
}
}
}
In the code block above, a string s1
gets declared as the first step. Next to it, the string gets utilized to create a character array. The toCharArray
function gets used to convert the string to a char
array. The function returns the character array with the length of the s1
string. It stores the characters at the same place as those present in the defined s1
string.
To iterate over the character array, you use the for each
loop. In this type of loop, no initialization of counter value or condition is to be explicitly specified. Instead, it declares a datatype variable and the collection over which the data variable is to iterate. Finally, in the loop block, the data variable gets printed.
Below is the output string for the code block above with a space added in between.
Output:
F i r s t S t r i n g
Declare a char
Array Using the new
Keyword in Java
package character_manipulation;
public class DeclareCharArray {
public static void main(String[] args) {
String s1 = "First String";
char[] charArray = new char[s1.length()];
for (int i = 0; i < s1.length(); i++) {
charArray[i] = s1.charAt(i);
System.out.print(charArray[i]);
}
}
}
In the code block above, the string gets initialized first. Next to it, a character array is declared using the new
keyword. The size of the char
array is the same as that of the length of the s1
string initialized. The size of the defined string gets evaluated using the length
method of the String
class. The method returns the length of the character sequence in the int
type.
A for
loop gets defined to iterate over the array. The first line in the loop is to initialize the char
array. It uses the charAt()
method to copy the content of the string into the array, and it returns the character at a particular index. The function throws an IndexOutOfBoundException
when the index ranges out of the length of the given string. At last, the sequence gets printed in the loop.
Below, you’ll see the output of the above code without any spaces in between.
Output:
First String
Declare and Initialize a char
Array Using the new
Keyword in Java
package character_manipulation;
public class DeclareCharArray {
public static void main(String[] args) {
char[] charArray2 = new char[] {'h', 'i', ' ', 't', 'h', 'e', 'r', 'e'};
String stringFromCharArray = new String(charArray2);
System.out.println(stringFromCharArray);
}
}