How to Convert Decimal to Binary in Java
- Convert Decimal to Binary Using Bitwise Operators in Java
- Convert Decimal to Binary Using Arrays in Java
-
Convert Decimal to Binary Using
Math.pow()
in Java
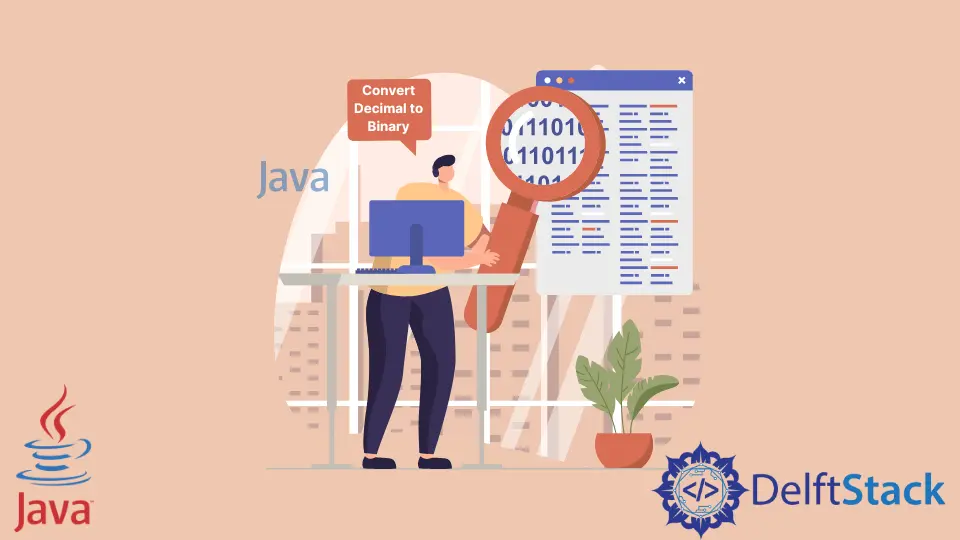
There are different approaches to converting decimal to binary in Java.
This tutorial demonstrates different ways how to convert decimal to binary in Java.
We can convert the decimal numbers to binary using the bitwise operators, arrays, and Math.pow()
method in Java.
Convert Decimal to Binary Using Bitwise Operators in Java
The bitwise operators usually work faster than the arithmetic operators; they can also be used to convert decimal to binary in Java. See example:
package delftstack;
import java.util.Scanner;
class Delftstack {
public void DecimalToBinary(int number) {
// Size of the integer is 32 bits
for (int x = number - 1; x >= 0; x--) {
int k = number >> x;
if ((k & 1) > 0) {
System.out.print("1");
} else {
System.out.print("0");
}
}
}
}
public class Decimal_to_Binary {
public static void main(String[] args) {
Delftstack Demo = new Delftstack();
System.out.println("Please enter a number");
Scanner New_Number = new Scanner(System.in);
int number = New_Number.nextInt();
System.out.println("The Input Decimal Number : " + number);
System.out.print("The Output Binary Number : ");
Demo.DecimalToBinary(number);
}
}
The code above will take an input of a decimal number and convert that number into binary. See the output:
Please enter a number
50
The Input Decimal Number : 50
The Output Binary Number : 00000000000011001000000000000000000000000000110010
Convert Decimal to Binary Using Arrays in Java
Follow the steps below to convert the decimal number to binary using arrays in Java.
-
First, store the remainder when the number is divided by two in the array.
-
Next, divide the number by two.
-
Then, we will repeat the above steps until the number is greater than 0.
-
Finally, print the array in the reverse order.
Let’s try an example based on the steps above.
package delftstack;
import java.io.*;
import java.util.Scanner;
class Decimal_to_Binary {
static void DecimalToBinary(int number) {
// an array which will store the binary number
int[] Binary_Number = new int[1000];
int x = 0;
while (number > 0) {
// store the remainder in the array
Binary_Number[x] = number % 2;
number = number / 2;
x++;
}
// print the final array in reverse order
for (int y = x - 1; y >= 0; y--) System.out.print(Binary_Number[y]);
}
public static void main(String[] args) {
System.out.println("Please enter a number");
Scanner New_Number = new Scanner(System.in);
int number = New_Number.nextInt();
System.out.println("The Input Decimal Number :" + number);
System.out.print("The Output Binary Number : ");
DecimalToBinary(number);
}
}
The code above will take an input of a decimal number and convert that number into binary. See output:
Please enter a number
33
The Input Decimal Number :33
The Output Binary Number : 100001
Convert Decimal to Binary Using Math.pow()
in Java
Math.pow()
method can also convert the decimal number to binary. Let’s try an example.
package delftstack;
import java.io.*;
import java.util.Scanner;
public class Decimal_to_Binary {
static int DecimalToBinary(int number) {
int Binary_number = 0;
int count = 0;
while (number != 0) {
int remainder = number % 2;
double temp = Math.pow(10, count);
Binary_number += remainder * temp;
number /= 2;
count++;
}
return Binary_number;
}
public static void main(String[] args) {
System.out.println("Please enter a number");
Scanner New_Number = new Scanner(System.in);
int number = New_Number.nextInt();
System.out.println("The Input Decimal Number : " + number);
System.out.print("The Output Binary Number : ");
System.out.println(DecimalToBinary(number));
}
}
The code above will take an input of a decimal number and convert that number into binary. See output:
Please enter a number
65
The Input Decimal Number : 65
The Output Binary Number : 1000001
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook