How to Crop Image in Java
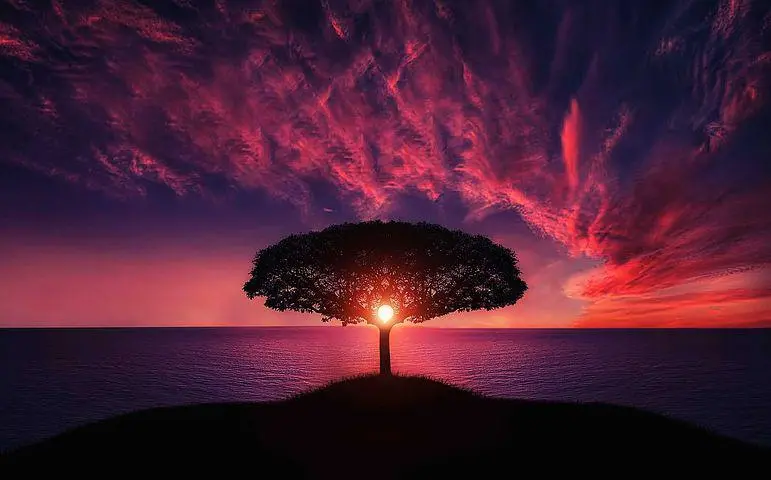
Image cropping is frequently used to remove obtrusive parts from images. You can crop an image to draw attention to a particular object in the frame, and this article will teach you how to crop photos in Java programmatically.
Even while paid tools have many uses and can save time, it is always worthwhile to try when we can make it in a matter of days. This post is primarily for beginners who can save money by avoiding third-party, expensive tools. We will use the subimage
function from the BufferedImage
class to crop an image in Java.
Crop an Image in Java Using BufferedImage
Class
The BufferedImage
class was considered when creating these examples.
Syntax of the BufferedImage
class’s getSubimage()
Method:
public BufferedImage getSubimage(int X, int Y, int width, int height)
This code will return a sub-image whose rectangular region is determined by the past inputs. Below is a description of the four parameters.
Parameters | Description |
---|---|
X |
provides the x coordinate of the upper-left corner of the chosen rectangular area. |
Y |
provides the y coordinate of the upper-left corner’s designated rectangle region. |
Height |
the height of the rectangular area is specified. |
Width |
defines how wide the rectangular area is. |
Following is the image we are going to crop.
Code Example:
import java.awt.Image;
import java.awt.image.BufferedImage;
import java.io.File;
import java.io.IOException;
import javax.imageio.ImageIO;
public class Main {
public static void main(String[] args) {
File imageFile = new File("/D:/test/image.jpg");
BufferedImage bufferedImage = null;
try {
bufferedImage = ImageIO.read(imageFile);
BufferedImage image = bufferedImage.getSubimage(0, 0, 50, 50);
File pathFile = new File("/D:/test/image-crop.jpg");
ImageIO.write(image, "jpg", pathFile);
} catch (IOException e) {
System.out.println(e);
}
}
}
Output:
Note that I have provided mine in lines 10 and 16, but you must provide your file location. Additionally, you can allow the user to provide X
, Y
, width
, and height
values at runtime to give your additional code flexibility.
Here we are looking for another example, to crop an image, we need some classes in Java. The following are the required classes to import before cropping an image in Java.
You must import the File
class to read and write to an image file. This class generally represents path names for files and directories.
Import java.io.File
The IOException
class is used to handle errors.
Import java.io.IOException
We use the BufferedImage
class to build the BufferedImage
object, which holds the image.
import java.awt.image.BufferedImage
We will import the ImageIO
class to carry out the read-write
operation on an image. Static image reading and writing techniques are available in this class.
import javax.imageio.ImageIO
Employing several of the BufferedImage
class’s built-in methods.
// importing the necessary packages
import java.awt.image.BufferedImage;
import java.io.File;
import java.io.IOException;
import javax.imageio.ImageIO;
// Main class
public class GFG {
// Main driver method
public static void main(String[] args) {
// Using a try block, look for exceptions
try {
// Reading the original image from the local path by
// creating an object of BufferedImage class
BufferedImage originalImg = ImageIO.read(new File("D:/test/Image.jpeg"));
// Fetching and printing alongside the
// dimensions of original image using getWidth()
// and getHeight() methods
System.out.println(
"Original Image Dimension: " + originalImg.getWidth() + "x" + originalImg.getHeight());
// Creating a subimage of given dimensions
BufferedImage SubImg = originalImg.getSubimage(50, 50, 50, 50);
// Printing Dimensions of the new image created
System.out.println(
"Cropped Image Dimension: " + SubImg.getWidth() + "x" + SubImg.getHeight());
// Creating a new file for the cropped image by
// creating an object of the File class
File outputfile = new File("D:/test/ImageCropped.jpeg");
// Writing image in the new file created
ImageIO.write(SubImg, "png", outputfile);
// Display message on console representing
// proper execution of program
System.out.println("Cropped Image created successfully");
}
// Catch block to handle the exceptions
catch (IOException e) {
// Print the exception along with the line number
// using printStackTrace() method
e.printStackTrace();
}
}
}
Additionally, after the program execution, the console will display an executed message and a newly created cropped image at the path specified.
Conclusion
You have clearly understood how to crop an image in Java. We have shown how to crop a picture whose rectangular region is determined by past inputs in a few different methods and how the BufferedImage
class and its other built-in methods are used to crop an image in Java.
Additionally, you can use the documentation to learn more about Java image cropping. Since you have read this page, you probably know what image cropping is.
It is simply the removal of the exterior portions of an image to improve the frame, alter the aspect ratio, etc. Now you can do it very quickly using the given Java codes.
Zeeshan is a detail oriented software engineer that helps companies and individuals make their lives and easier with software solutions.
LinkedIn