Javaで画像をトリミング
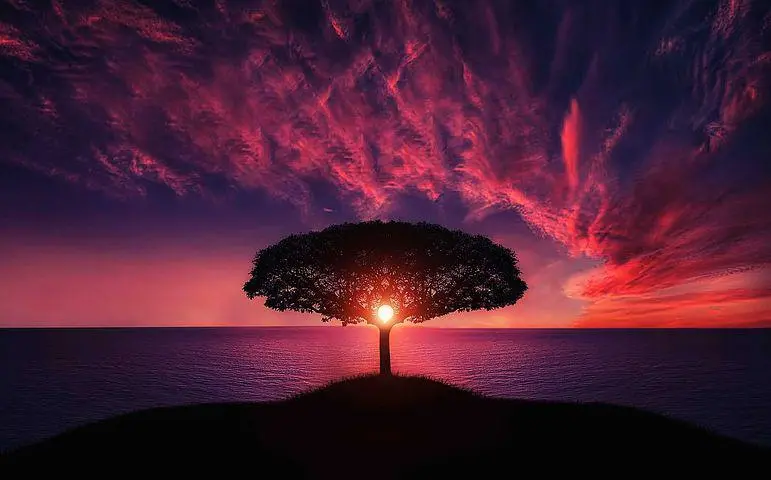
画像のトリミングは、画像から目障りな部分を削除するためによく使用されます。 画像をトリミングして、フレーム内の特定のオブジェクトに注目を集めることができます。この記事では、Java でプログラムを使用して写真をトリミングする方法を説明します。
有料ツールには多くの用途があり、時間を節約できますが、数日で作成できる場合は、常に試してみる価値があります. この投稿は主に、サードパーティの高価なツールを避けることでお金を節約できる初心者向けです。 BufferedImage
クラスの subimage
関数を使用して、Java で画像をトリミングします。
BufferedImage
クラスを使用して Java で画像をトリミングする
これらの例を作成するとき、BufferedImage
クラスが考慮されました。
BufferedImage
クラスの getSubimage()
メソッドの構文:
public BufferedImage getSubimage(int X, int Y, int width, int height)
このコードは、過去の入力によって決定される矩形領域を持つサブイメージを返します。 以下は、4つのパラメーターの説明です。
パラメーター | 説明 |
---|---|
X |
選択した長方形領域の左上隅の x 座標を提供します。 |
Y |
左上隅の指定された長方形領域の y 座標を提供します。 |
Height |
長方形領域の高さを指定します。 |
Width |
長方形領域の幅を定義します。 |
以下は、トリミングする画像です。
コード例:
import java.awt.Image;
import java.awt.image.BufferedImage;
import java.io.File;
import java.io.IOException;
import javax.imageio.ImageIO;
public class Main {
public static void main(String[] args) {
File imageFile = new File("/D:/test/image.jpg");
BufferedImage bufferedImage = null;
try {
bufferedImage = ImageIO.read(imageFile);
BufferedImage image = bufferedImage.getSubimage(0, 0, 50, 50);
File pathFile = new File("/D:/test/image-crop.jpg");
ImageIO.write(image, "jpg", pathFile);
} catch (IOException e) {
System.out.println(e);
}
}
}
出力:
私は 10 行目と 16 行目で私のものを提供しましたが、ファイルの場所を提供する必要があることに注意してください。 さらに、実行時にユーザーが X
、Y
、width
、および height
の値を指定できるようにして、コードの柔軟性を高めることができます。
ここでは、別の例を探しています。画像をトリミングするには、Java でいくつかのクラスが必要です。 以下は、Java で画像をトリミングする前にインポートする必要があるクラスです。
画像ファイルを読み書きするには、File
クラスをインポートする必要があります。 このクラスは通常、ファイルとディレクトリのパス名を表します。
Import java.io.File
IOException
クラスは、エラーを処理するために使用されます。
Import java.io.IOException
BufferedImage
クラスを使用して、画像を保持する BufferedImage
オブジェクトを作成します。
import java.awt.image.BufferedImage
ImageIO
クラスをインポートして、画像に対して 読み書き
操作を実行します。 このクラスでは、静止画像の読み取りと書き込みのテクニックを利用できます。
import javax.imageio.ImageIO
BufferedImage
クラスの組み込みメソッドのいくつかを使用します。
// importing the necessary packages
import java.awt.image.BufferedImage;
import java.io.File;
import java.io.IOException;
import javax.imageio.ImageIO;
// Main class
public class GFG {
// Main driver method
public static void main(String[] args) {
// Using a try block, look for exceptions
try {
// Reading the original image from the local path by
// creating an object of BufferedImage class
BufferedImage originalImg = ImageIO.read(new File("D:/test/Image.jpeg"));
// Fetching and printing alongside the
// dimensions of original image using getWidth()
// and getHeight() methods
System.out.println(
"Original Image Dimension: " + originalImg.getWidth() + "x" + originalImg.getHeight());
// Creating a subimage of given dimensions
BufferedImage SubImg = originalImg.getSubimage(50, 50, 50, 50);
// Printing Dimensions of the new image created
System.out.println(
"Cropped Image Dimension: " + SubImg.getWidth() + "x" + SubImg.getHeight());
// Creating a new file for the cropped image by
// creating an object of the File class
File outputfile = new File("D:/test/ImageCropped.jpeg");
// Writing image in the new file created
ImageIO.write(SubImg, "png", outputfile);
// Display message on console representing
// proper execution of program
System.out.println("Cropped Image created successfully");
}
// Catch block to handle the exceptions
catch (IOException e) {
// Print the exception along with the line number
// using printStackTrace() method
e.printStackTrace();
}
}
}
さらに、プログラムの実行後、コンソールには、実行されたメッセージと、指定されたパスに新しく作成されたトリミングされた画像が表示されます。
まとめ
Java で画像をトリミングする方法を明確に理解しました。 長方形の領域が過去の入力によって決定される画像をいくつかの異なるメソッドでトリミングする方法と、BufferedImage
クラスとその他の組み込みメソッドを使用して Java で画像をトリミングする方法を示しました。
さらに、ドキュメンテーションを使用して、Java 画像のクロッピングについて詳しく知ることができます。 このページを読んだあなたは、おそらく画像のトリミングが何であるかを知っているでしょう。
これは、フレームを改善したり、縦横比を変更したりするために、画像の外側部分を単純に削除することです。これで、指定された Java コードを使用して非常に迅速に行うことができます。
Zeeshan is a detail oriented software engineer that helps companies and individuals make their lives and easier with software solutions.
LinkedIn