How to Create SSH Connection in Java
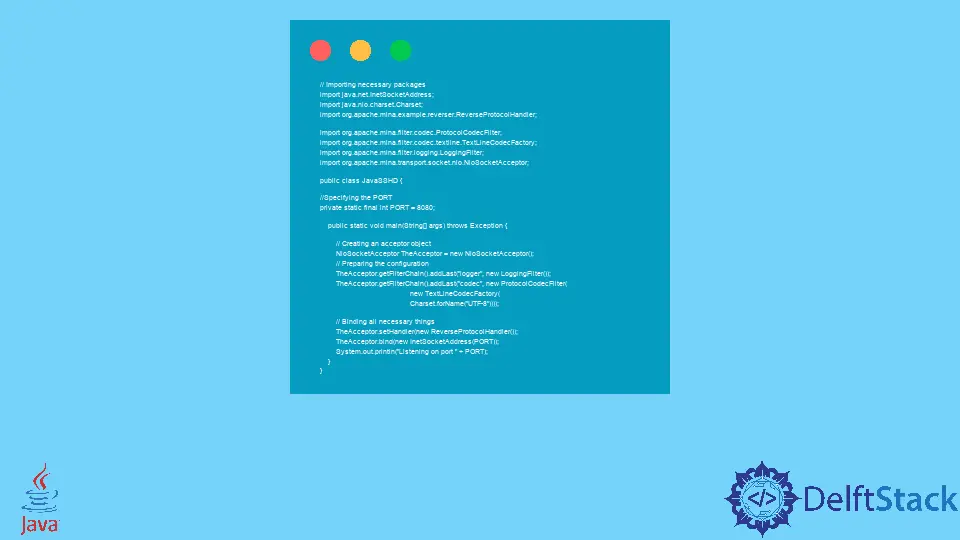
The SSH, also known as the Secure Shell or Secure Socket Shell, is a network protocol that allows us to connect over a secured connection to another computer.
Today’s tutorial discusses how to open an SSH connection and demonstrates the use of JSch
and Apache Mina SSHD
to create an SSH connection.
Create SSH Connection in Java
There are various ways but using JSch
and Apache Mina SSHD
are the most common, so let’s learn them one by one.
Method 1: Use JSch
to Create an SSH Connection in Java
JSch
is an implementation of SSH2
written in pure Java. This library allows you to connect to an SSHD server. It also allows you to use X11 forwarding, port
forwarding, file transfer, and more.
Our example below will create a simple SSH connection using the JSch
.
Example Code:
// Importing necessary packages
import com.jcraft.jsch.*;
import java.io.InputStream;
public class JavaJSCH {
public static void main(String[] args) {
// Organizing all necessary elements for authenticating
String MyHost = "test.rebex.net";
String User = "demo";
String Password = "password";
try {
// Configuring
java.util.Properties Config = new java.util.Properties();
// Disable the 'StrictHostKeyChecking'
Config.put("StrictHostKeyChecking", "no");
// Declaring a JSch object
JSch Jsch = new JSch();
// Creating a session
Session MySession = Jsch.getSession(User, MyHost, 22);
// Setting the session password
MySession.setPassword(Password);
// Configuring the session
MySession.setConfig(Config);
// Connecting the session
MySession.connect();
System.out.println("Successfully Connected !!!");
} catch (Exception e) {
e.printStackTrace();
}
}
}
Output:
Successfully Connected !!!
Method 2: Use Apache Mina SSHD
to Create an SSH Connection in Java
The Apache Mina SSHD
is another library to create a connection with a server using the SSH protocols. This library is also written in pure Java, and the library is mainly based on Apache MINA
.
Our example below will create a simple SSH connection using the Apache Mina SSHD
.
Example Code:
// Importing necessary packages
import java.net.InetSocketAddress;
import java.nio.charset.Charset;
import org.apache.mina.example.reverser.ReverseProtocolHandler;
import org.apache.mina.filter.codec.ProtocolCodecFilter;
import org.apache.mina.filter.codec.textline.TextLineCodecFactory;
import org.apache.mina.filter.logging.LoggingFilter;
import org.apache.mina.transport.socket.nio.NioSocketAcceptor;
public class JavaSSHD {
// Specifying the PORT
private static final int PORT = 8080;
public static void main(String[] args) throws Exception {
// Creating an acceptor object
NioSocketAcceptor TheAcceptor = new NioSocketAcceptor();
// Preparing the configuration
TheAcceptor.getFilterChain().addLast("logger", new LoggingFilter());
TheAcceptor.getFilterChain().addLast(
"codec", new ProtocolCodecFilter(new TextLineCodecFactory(Charset.forName("UTF-8"))));
// Binding all necessary things
TheAcceptor.setHandler(new ReverseProtocolHandler());
TheAcceptor.bind(new InetSocketAddress(PORT));
System.out.println("Listening on port " + PORT);
}
}
The example shared above illustrates a basic level of a server program that provides a list of the port. When you run the example code shared above, you will get the below output in your console.
Output:
Listening on port 8080
Aminul Is an Expert Technical Writer and Full-Stack Developer. He has hands-on working experience on numerous Developer Platforms and SAAS startups. He is highly skilled in numerous Programming languages and Frameworks. He can write professional technical articles like Reviews, Programming, Documentation, SOP, User manual, Whitepaper, etc.
LinkedIn