How to Create PPTP Connection in Java
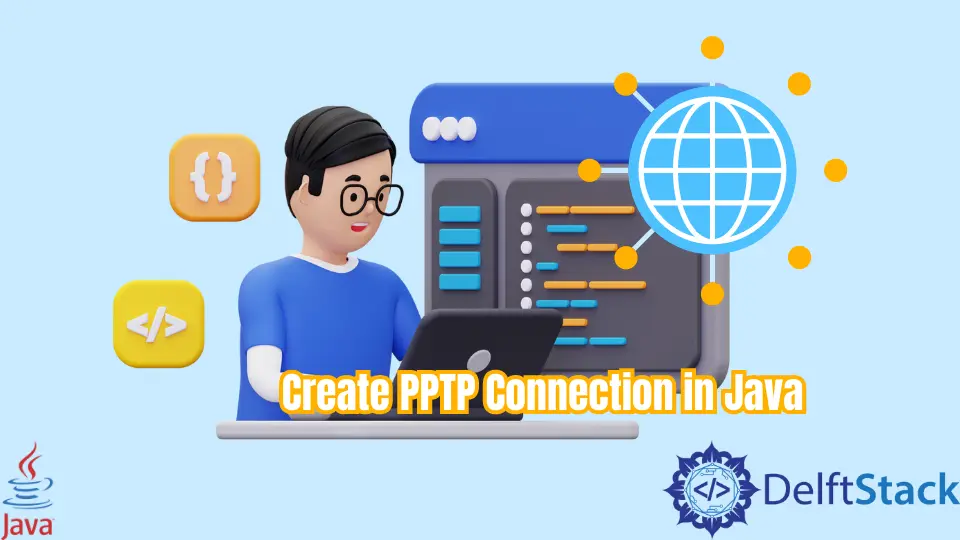
In this article, we’ll learn how to create Point-to-Point Tunneling Protocol (PPTP) connection using Java.
Why We Need a PPTP Connection
The Point-to-Point Tunneling Protocol is used to encapsulate the compressed and encrypted Point-to-Point Protocol (PPP) packet into IP datagrams for sending over the Internet.
The IP datagrams keep routing over the network until they reach the Point-to-Point Tunneling Protocol Server, connected to the private network and Internet.
The PPTP server deconstructs (disassembles) the IP datagram into Point-to-Point Protocol (PPP) packet decrypts the PPP packet via the private network’s network protocol. Remember, the network protocol on a private network supported by PPTP is TCP/IP
, NetBEUI
, IPX
.
This tutorial will use a TCP/IP
connection. So, let’s start with a practical example.
Create PPTP Connection in Java
Example Code (client.java
):
import java.io.*;
import java.net.*;
public class client {
public static void main(String[] args) throws IOException {
Socket socket = new Socket("localhost", 5000);
PrintWriter printWriter = new PrintWriter(socket.getOutputStream());
printWriter.println("Is it working?");
printWriter.flush();
InputStreamReader in = new InputStreamReader(socket.getInputStream());
BufferedReader bufferReader = new BufferedReader(in);
String str = bufferReader.readLine();
System.out.println("server : " + str);
}
}
Example Code (server.java
):
import java.io.*;
import java.net.*;
public class server {
public static void main(String[] args) throws IOException {
ServerSocket serverSocket = new Socket(5000);
Socket socket = serverSocket.accept();
System.out.println("client connected");
InputStreamReader in = new InputStreamReader(socket.getInputStream());
BufferedReader bufferReader = new BufferedReader(in);
String str = bufferReader.readLine();
System.out.println("client : " + str);
PrintWriter printWriter = new PrintWriter(socket.getOutputStream());
printWriter.println("Yes");
printWriter.flush();
}
}
Output:
Here, the client sends the message as Is it working?
and the server responds with Yes
.
How? Let’s understand what happens in the client.java
and server.java
classes.
In the client.java
class, we use a class named Socket
to connect with the server. The Socket
class takes two parameters, the IP address and a TCP Port number.
IP address means the address of the server. We are running both programs (client.java
& server.java
) on the same machine; that’s why we wrote localhost
.
The TCP port number displays the application executed on the server.
We can use any port number ranging from 0
to 64535
. We are using 5000
in this tutorial.
For communication purposes over the socket connection, we are using streams for input and output both. Why? We are implementing two-way communication (client to server & server to client).
In the server.java
class, we need two sockets. One is a simple socket of the Socket
class that will be used for communication with the client, and the other is ServerSocket
that waits for the client’s requests.
These requests are received on the server-side when a client makes a new object of the Socket
class by using new Socket(IP address, port number)
.
Before using getInputStream()
function to accept input from socket, the accept()
function blocks and sits until the client connects with the server. Here, we are using input and output streams to get the data from the client and send data to the client.
You may have a question: Why do we need streams for client and server? It is because the raw data can’t be sent over the network.
The data needs to be converted into streams to communicate from client-to-server and server-to-client.