How to Create GUID in Java
- What Is GUID
-
Example Code to Generate UUID Using
randomUUID()
Method -
Example Code to Generate UUID Using
UUID constructor
andfromString()
Method
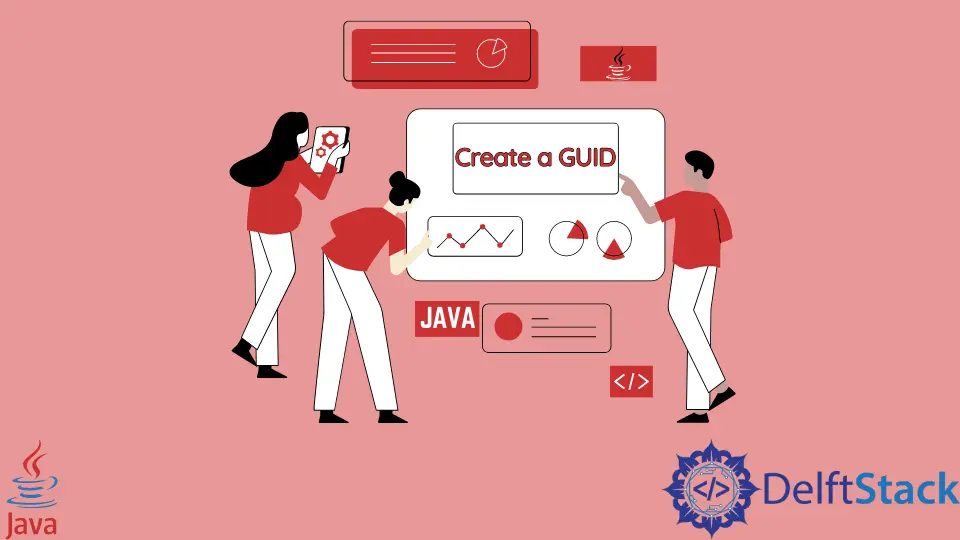
What Is GUID
GUID is an acronym for Globally Unique Identifier
. It is often also referred to Universally Unique Identifiers
or UUIDs
. There is no genuine difference between the two terms. Technically, these are 128-bit immutable, unique, cryptographically-strong, random numbers. These random numbers are eventually in use for computing purposes. The algorithm to generate such numbers is so complex that it could generate 1,0000,000,000 random numbers per second. These numbers are unlikely to repeat.
We use GUID or UUIDs in software development practices and methodologies, where we would like huge transactions to happen with a unique ID as a primary key that is database keys, component identifiers, and varied transactions.
Example Code to Generate UUID Using randomUUID()
Method
package guid;
import java.util.UUID;
public class CreateGuid {
public static void main(String[] args) {
UUID uuid = UUID.randomUUID();
System.out.println("UUID = " + uuid.toString());
}
}
In Java language, the UUID
class is available after version 1.5. The class is present in the java.util.UUID
package. The randomUUID()
method is a static factory method to retrieve a type 4 (pseudo-randomly generated) UUID. As the method is a static factory method of the UUID class hence the class name is required to call the method.
The output from the above program seems to be a uniquely generated UUID.
UUID = 70aba303-60d8-4cb5-b3e7-4170c4be5642
Example Code to Generate UUID Using UUID constructor
and fromString()
Method
In the below program, the UUID
class constructor is used to generate a UUID. The constructor takes two parameters, mostSignificantBits
and leastSignificantBits
.
The mostSignificantBits
is for the most significant 64 bits of the UUID, and the leastSignificantBits
is for the least significant 64 bits.
The fromString()
method is a static factory method that creates a UUID from the string standard representation. The above method takes String
as a parameter. So over the uuid
object, the toString()
method is called in the inline function.
The fromString
method throws IllegalArgumentException
if the name does not conform to the string representation described in the toString
method.
package guid;
import java.util.UUID;
public class GenerateGuidUsingConstructor {
public static void main(String[] args) {
UUID uuid = new UUID(24, 02);
System.out.println(UUID.fromString(uuid.toString()));
}
}