How to Convert C# Codes to Java Codes
-
Convert Whole C# Project to Java Using
C# to Java Converter Tool
- Convert C# Code Snippet to Java Using C# to Java Converter Tool
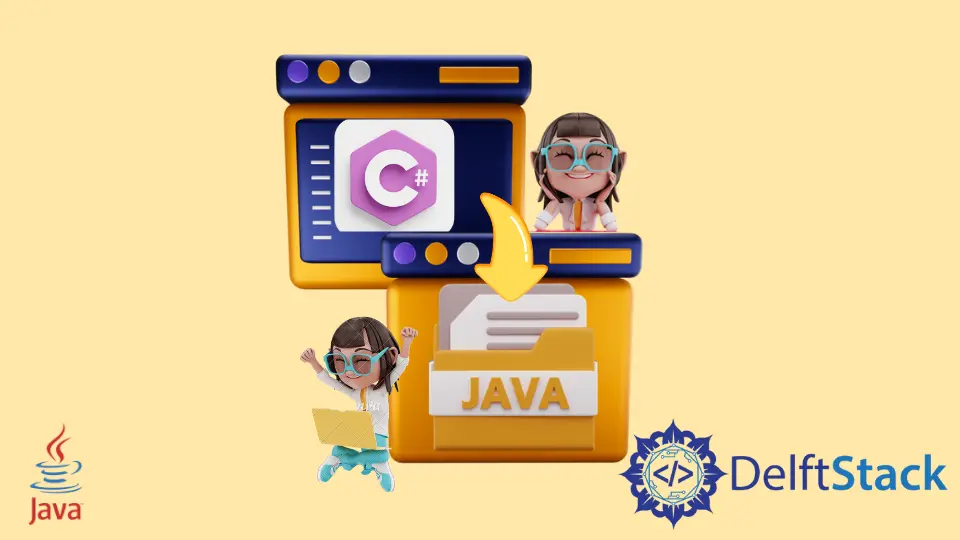
This article will discuss using a C# to Java converter tool to convert C# code to Java code. You can download this tool here.
Convert Whole C# Project to Java Using C# to Java Converter Tool
There are two ways to convert C# code to Java using the C# to Java Converter tool
. The first way is to select the whole C# project for conversion using the Project & Folder Converter
tab.
In the following image, we can see three options to browse through the file explorer. We can select a C# project by clicking the Browse button in the C# source project section and selecting the project file.
We can select a folder instead of a C# project file using the C# Source Folder
option, and the last field is to specify the Java Target Folder where the converted code will be stored.
At the bottom of the tool, we can see that it shows the conversion history and conversion date.
The C# project contains the below C# program.
using System;
namespace Example {
internal class Program {
private static void Main(string[] args) {
Console.Write("Enter the principle amount: ");
var principle = Console.ReadLine();
Console.Write("\n Enter the interest rate: ");
var rateOfInterest = Console.ReadLine();
Console.Write("\n Enter the duration: ");
var duration = Console.ReadLine();
var total = Convert.ToInt32(principle) *
Math.Pow(1 + Convert.ToInt32(rateOfInterest), Convert.ToInt32(duration));
Console.WriteLine("\n The total value of your $" + initial + " investement after " + years +
" years @ " + rate + "% is $" + total + ".\n\n.");
System.Threading.Thread.Sleep(10000);
}
}
}
After conversion, we can see that the tool converted the whole program into a Java program inside the project.
import java.util.*;
public class Program {
public static void main(String[] args) {
System.out.print("Enter the principle amount: ");
var principle = new Scanner(System.in).nextLine();
System.out.print("\n Enter the interest rate: ");
var rateOfInterest = new Scanner(System.in).nextLine();
System.out.print("\n Enter the duration: ");
var duration = new Scanner(System.in).nextLine();
var total = Integer.parseInt(principle)
* Math.pow(1 + Integer.parseInt(rateOfInterest), Integer.parseInt(duration));
System.out.println("\n The total value of your $" + principle + " investement after " + duration
+ " years @ " + rateOfInterest + "% is $" + total + ".\n\n.");
Thread.sleep(10000);
}
}
Output:
Enter the principle amount: 150000
Enter the interest rate: 12
Enter the duration: 10
The total value of your $150000 investement after 10 years @ 12% is $2.067877377735E16.
Convert C# Code Snippet to Java Using C# to Java Converter Tool
Another way to convert a C# code to Java code is using the File & Snippet Converter
of the converter tool that allows us to convert a single file containing the C# code or a C# snippet to Java.
In the image below, we select the second tab of the tool called File & Snippet Converter
, which shows two editors and buttons Clear, Insert File and Convert on the left side, while on the right bottom side, we have Clear, Copy All, and Save buttons.
To convert a C# snippet, we paste the code into the left editor and click on the Convert button.
After clicking the Convert button, the tool converts the C# code to Java and shows it on the right editor. We can save this in a file using the Save button or copy it.
Below is the C# code that we used in this example to convert to Java.
using System;
public class ReverseNumExample {
public static void Main(string[] args) {
int num, reverse = 0, rem;
Console.WriteLine("Enter a number you want to reverse");
num = int.Parse(Console.ReadLine());
while (num != 0) {
rem = num % 10;
reverse = reverse * 10 + rem;
num /= 10;
}
Console.WriteLine("Reversed number: {0}", reverse);
Console.ReadLine();
}
}
Output:
Enter a number you want to reverse
278
Reversed number: 872
Here is the converted code that runs successfully.
import java.util.*;
public class ReverseNumExample {
public static void main(String[] args) {
int num, reverse = 0, rem;
System.out.println("Enter a number you want to reverse");
num = Integer.parseInt(new Scanner(System.in).nextLine());
while (num != 0) {
rem = num % 10;
reverse = reverse * 10 + rem;
num /= 10;
}
System.out.printf("Reversed number: %1$s"
+ "\r\n",
reverse);
new Scanner(System.in).nextLine();
}
}
Output:
Enter a number you want to reverse
567
Reversed number: 765
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedIn