ConcurrentHashMap vs Hashtable in Java
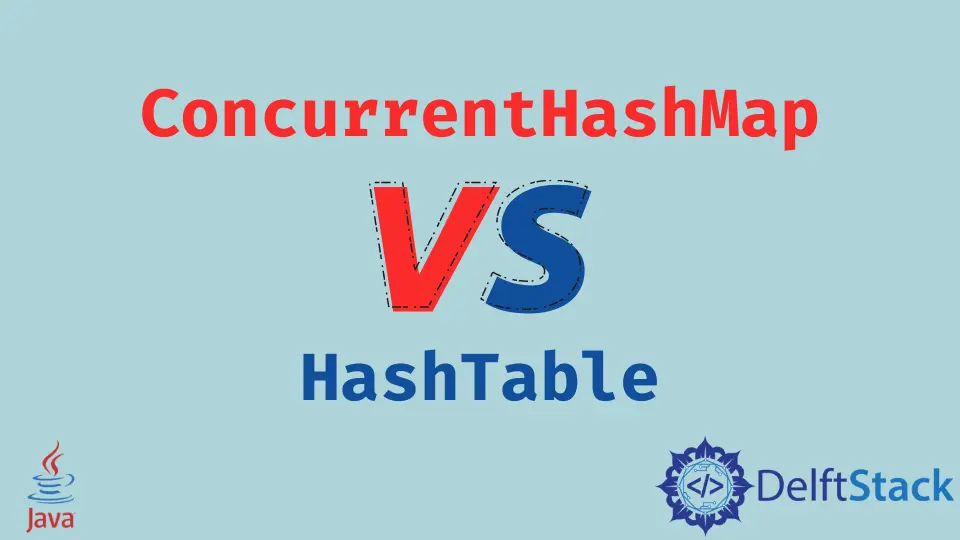
This tutorial introduces the difference between ConcurrentHashMap and Hashtable in Java.
ConcurrentHashMap is a class that belongs to java.util.concurrent
framework. It implements ConcurrentMap and a Serializable interface. It is used to store data that is thread-safe. It uses multiple buckets to store data. The declaration syntax of this is:
public class ConcurrentHashMap<K, V>
extends AbstractMap<K, V> implements ConcurrentMap<K, V>, Serializable
ConcurrentHashMap Keypoints
- It is thread-safe.
- It uses multiple locks on segment level instead of the whole map.
- It uses 16 locks by default.
- It applies to lock only for updates. For reading, it allows multiple threads to access the data.
Let’s see an example of ConcurrentHashMap.
Creating ConcurrentHashMap in Java
In this example, we created a ConcurrentHashMap that holds data of String and Integer type. We used the put()
method to add elements and, getKey()
and getValue()
methods to access key and value respectively. See the example below.
import java.util.Map;
import java.util.concurrent.ConcurrentHashMap;
public class SimpleTesting {
ConcurrentHashMap<String, Integer> hm = new ConcurrentHashMap<String, Integer>();
public void AddScore(String name, int score) {
hm.put(name, score);
}
public static void main(String[] args) {
SimpleTesting simpleTesting = new SimpleTesting();
simpleTesting.AddScore("Rohan", 95);
simpleTesting.AddScore("Sohan", 85);
simpleTesting.AddScore("Mohan", 93);
simpleTesting.AddScore("Lohan", 91);
System.out.println("Students Scores: ");
for (Map.Entry<String, Integer> entry : simpleTesting.hm.entrySet()) {
System.out.println(entry.getKey() + " - " + entry.getValue());
}
}
}
Output:
Students Scores:
Lohan - 91
Mohan - 93
Sohan - 85
Rohan - 95
HashTable is a class that belongs to the Java collection framework. It is used to store data in key and value pairs. It implements Map, Cloneable, and Serializable interface. The class declaration is given below.
public class Hashtable<K, V> extends Dictionary<K, V> implements Map<K, V>, Cloneable, Serializable
HashTable Keypoints:
- It uses a single lock for the whole data.
- It is thread-safe, i.e., synchronized.
- It does not allow null keys or values.
Let’s see an example of HashTable.
Creating HashTable in Java
In this example, we created a HashTable that store data of string and integer type. We used put()
method to add elements and getKey()
, and getValue()
method to access key and value respectively. See the example below.
import java.util.Hashtable;
import java.util.Map;
public class SimpleTesting {
Hashtable<String, Integer> hm = new Hashtable<String, Integer>();
public void AddScore(String name, int score) {
hm.put(name, score);
}
public static void main(String[] args) {
SimpleTesting simpleTesting = new SimpleTesting();
simpleTesting.AddScore("Rohan", 95);
simpleTesting.AddScore("Sohan", 85);
simpleTesting.AddScore("Mohan", 93);
simpleTesting.AddScore("Lohan", 91);
System.out.println("Students Scores: ");
for (Map.Entry<String, Integer> entry : simpleTesting.hm.entrySet()) {
System.out.println(entry.getKey() + " - " + entry.getValue());
}
}
}
Output:
Students Scores:
Rohan - 95
Mohan - 93
Sohan - 85
Lohan - 91