Class Field and Instance Field in Java
- Difference between Class Fields and Instance Fields
- Class Field in Java
- Instance Field in Java
- Conclusion
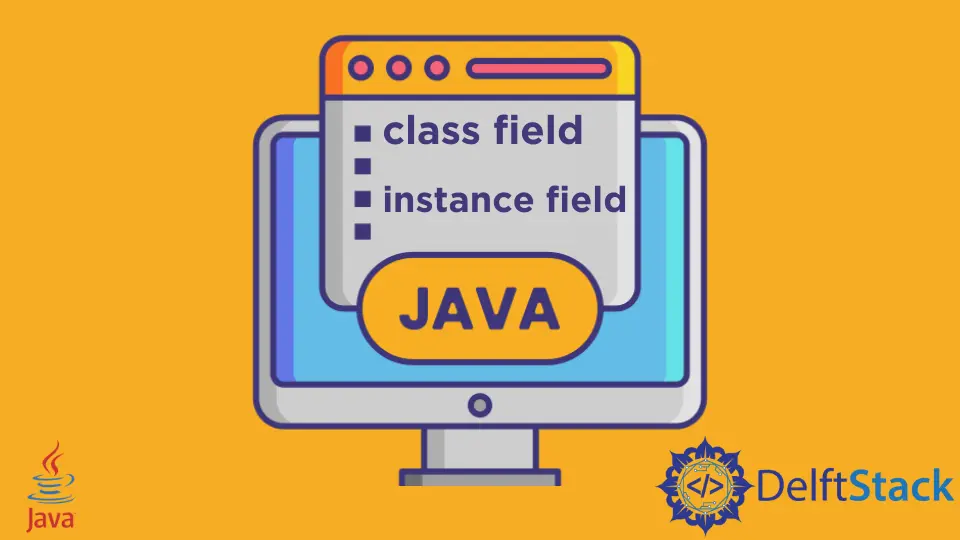
This article explains the importance of understanding the distinction between class fields and instance fields in Java programming. It highlights various aspects and considerations associated with these two types of fields within a class.
Difference between Class Fields and Instance Fields
Understanding the distinction between class fields and instance fields is crucial in Java programming because it directly impacts how data is stored, accessed, and shared within a class and its instances.
Understanding when to use class fields and when to use instance fields allows developers to design classes that reflect the desired behavior and structure of their software. It enables effective data encapsulation, promotes code clarity, and ensures that shared data behaves as intended across instances.
Here are the differences between class fields (static fields) and instance fields (non-static fields):
Aspect | Class Fields (Static Fields) | Instance Fields (Non-static Fields) |
---|---|---|
Scope | Shared among all instances of the class. | Unique to each instance of the class. |
Keyword | Declared using the static keyword. |
Declared without the static keyword. |
Memory Allocation | Allocated memory only once when the class is loaded into memory. | Allocated memory for each instance of the class when an object is created. |
Access | Can be accessed using the class name without creating an instance of the class. | Requires an instance of the class to access the instance field. |
Usage | Used for values that are common to all instances of the class (e.g., constants or shared resources). | Used for attributes or properties that are specific to each instance of the class. |
Class fields are shared among all instances of a class, and there is only one copy of them in memory. Instance fields, on the other hand, are unique to each instance of the class and have a separate copy for each object created from the class.
Class Field in Java
In the realm of Java programming, a crucial concept that often plays a pivotal role in crafting robust and efficient class designs is that of class fields. Class fields provide a mechanism for storing shared data that is common to all instances of a class.
This shared nature makes them particularly useful for scenarios where certain attributes should remain consistent across all objects derived from the same class.
Let’s consider a straightforward example that emphasizes the use of class fields without involving instance fields. We’ll create a Counter
class that keeps track of the total count of instances created.
Example:
public class Counter {
// Class field to store the total count of instances
private static int totalCount = 0;
// Constructor increments the class field on each object creation
public Counter() {
totalCount++;
}
// Method to retrieve the total count of instances
public static int getTotalCount() {
return totalCount;
}
// Main method for demonstration
public static void main(String[] args) {
// Creating instances of Counter
Counter counter1 = new Counter();
Counter counter2 = new Counter();
// Retrieving and printing the total count
System.out.println("Total Count of Instances: " + Counter.getTotalCount());
}
}
In our Counter
class, we introduce a class field named totalCount
using the static
keyword. This field is vital as it’s shared among all instances of the class, keeping a collective count of the total instances created.
The class constructor, a simple yet crucial element, increments the totalCount
field upon the creation of each object. This ensures that the total count precisely reflects the number of instances in existence.
Additionally, the class provides a static method, getTotalCount
, offering a way to retrieve the total count without needing to create an instance of the Counter
class.
In the main
method, we showcase this functionality by creating two instances of the class and using the getTotalCount
method to display the overall count of instances.
Output:
Total Count of Instances: 2
The output demonstrates how the class field totalCount
effectively keeps track of the total count of instances, showcasing the shared nature of class fields.
This example highlights the importance of class fields in maintaining shared data across objects instantiated from the same class.
Instance Field in Java
Instance fields represent the unique attributes or properties of individual objects created from a class. Unlike class fields, these are not shared among all instances but rather encapsulate state specific to each object.
This part of the article aims to unravel the concept of instance fields, showcasing their importance and providing a practical example for clarity.
Let’s explore instance fields through the lens of a simple Person
class. Each person will have instance fields such as name
and age
, demonstrating how these fields store distinct information for each object.
Example:
public class Person {
// Instance fields
private String name;
private int age;
// Constructor to initialize instance fields
public Person(String name, int age) {
this.name = name;
this.age = age;
}
// Method to display information about the person
public void displayInfo() {
System.out.println("Name: " + name);
System.out.println("Age: " + age);
}
// Other methods for additional functionalities
public static void main(String[] args) {
// Creating instances of Person
Person person1 = new Person("John Doe", 25);
Person person2 = new Person("Jane Doe", 30);
// Displaying information about each person
System.out.println("Person 1:");
person1.displayInfo();
System.out.println("\nPerson 2:");
person2.displayInfo();
}
}
In the Person
class, we define name
and age
as instance fields. These fields, distinct for each object, are not marked with the static
keyword, emphasizing their association with individual objects rather than the entire class.
Every instance of the Person
class possesses its own set of these fields. The constructor method plays a key role by taking parameters (name
and age
) and initializing the instance fields accordingly.
When a new Person
object is created, this constructor is called to set the specific values for that instance’s fields.
The displayInfo
method, a simple utility in the class, effectively showcases the information stored in the instance fields, demonstrating how these fields encapsulate behavior specific to each instance.
In the main
method, we illustrate this concept by creating two Person
instances (person1
and person2
). Each person object has its own unique set of instance fields (name
and age
).
Invoking the displayInfo
method for each person highlights the distinct data stored in their instance fields, exemplifying the individuality and encapsulation afforded by instance fields in Java classes.
Output:
Person 1:
Name: John Doe
Age: 25
Person 2:
Name: Jane Doe
Age: 30
The output shows the distinctive nature of instance fields. Each person object maintains its own values for name
and age
, showcasing the encapsulation of state within individual instances.
Conclusion
Comprehending the use of class and instance fields empowers developers to craft classes aligning with their software’s intended behavior and structure. This knowledge facilitates efficient data encapsulation, enhances code clarity, and ensures shared data behaves as intended across instances.
In Java, mastering class fields enhances code modularity and maintainability. Recognizing their shared nature empowers developers to design classes efficiently, ensuring data consistency across all instances.
On the other hand, understanding instance fields is vital for Java’s object-oriented applications. These fields model object uniqueness, fostering encapsulation and code reuse.
By grasping its role, developers craft flexible, scalable software.