How to Call Python Script From Java Code
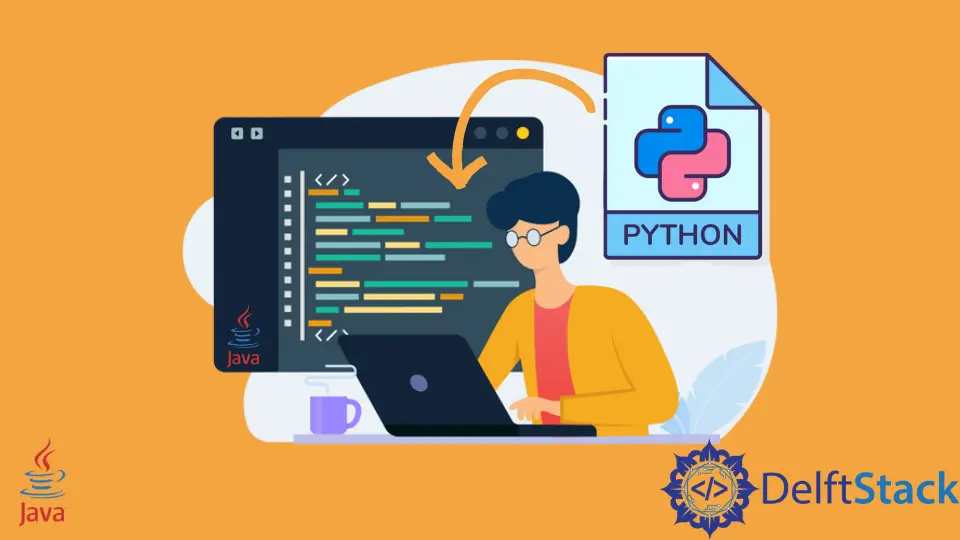
This tutorial demonstrates three different approaches to calling Python scripts from Java code.
Call Python Script From Java Code
Sometimes, we must call Python scripts from our Java code to meet the project requirements. We can do that using Process
, ProcessBuilder
, and Jython
. Let’s see how we can use each of them below.
Use Process
Class to Run Python Script From Java Code
We can use the Process
class of Java to run Python scripts from our Java code. Let’s try to create a Python script and run it using Java code. Follow the steps below:
- Copy your Python script in a string in Java.
- Create a file with the
.py
extension usingBufferedWriter
. - Write the content from the above string in the Python file and close the writer.
- Create a process to run the Python file using
Runtime.getRuntime().exec()
. - Read the data from the process using
BufferedReader
. - Use or print the data retrieved from running the Python code.
Now let’s implement the above steps in Java code:
import java.io.BufferedReader;
import java.io.BufferedWriter;
import java.io.FileWriter;
import java.io.InputStreamReader;
public class Example {
public static void main(String a[]) {
try {
String Python_Script = "print(\"Hello, This is Delftstack.com!The Best Tutorial Site!\")\n";
BufferedWriter Buffered_Writer = new BufferedWriter(new FileWriter("DemoPythonFile.py"));
Buffered_Writer.write(Python_Script);
Buffered_Writer.close();
Process Demo_Process = Runtime.getRuntime().exec("python DemoPythonFile.py");
BufferedReader Buffered_Reader =
new BufferedReader(new InputStreamReader(Demo_Process.getInputStream()));
} catch (Exception e) {
e.printStackTrace();
}
}
}
The code above tries to create and run a Python script in Java and write the following string in the DemoPythonFile.py
file.
Hello, This is Delftstack.com!The Best Tutorial Site!
We should mention that it is not necessary to create a script of Python and then run it; you can also directly load your Python script and its output in Java code.
Note that you may get a warning about deprecation for the Runtime.getRuntime().exec()
function, which means Java may remove this function in future releases.
Use ProcessBuilder
Class to Run Python Script From Java Code
The ProcessBuilder
class in Java is used to create an Operating system process. We can also use this class to run Python scripts in Java.
Follow the steps to run Python scripts in Java using the ProcessBuilder
Class:
- Make sure Python is installed on your system.
- Create a Python script with the
.py
extension or select one if you already have one. - Create an instance of the
ProcessBuilder
class and passpython
and script path as parameters. - Create a process to run the
ProcessBuilder
using thestart()
method; this will execute the Python script. - Create a
BufferedReader
to get the output of the Python script from the process. - Print the output.
Let’s implement the example in Java. Our Python script is:
print("Hello, This is Delftstack.com! The Best Tutorial Site!")
Here is the implementation of Java:
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
public class Example {
public static void main(String[] args) throws IOException, InterruptedException {
String Script_Path = "C:\\Users\\Sheeraz\\script.py";
ProcessBuilder Process_Builder = new ProcessBuilder("python", Script_Path).inheritIO();
Process Demo_Process = Process_Builder.start();
Demo_Process.waitFor();
BufferedReader Buffered_Reader =
new BufferedReader(new InputStreamReader(Demo_Process.getInputStream()));
String Output_line = "";
while ((Output_line = Buffered_Reader.readLine()) != null) {
System.out.println(Output_line);
}
}
}
The code above will execute the given Python script using Java and print it on the console. See output:
Hello, This is Delftstack.com! The Best Tutorial Site!
Use Jython
to Run Python and Java Code Together
The Jython
is a project to provide Python script implementation in Java code. Jython
runs the Python and Java code with each other.
To use Jython
in Java, we just need to download the Jython-standalone-2.7.2.jar
or any latest version and add it to your project’s build path
. Follow the steps below to add Jython
to your Java environment:
- Download
Jython Standalone
from here. - Add the
Jython-standalone-2.7.2.jar
to your buildpath
. - Go to project
Properties
->Java Build Path
. - Add External Jar to your
classpath
. - Select
Jython-standalone-2.7.2.jar
and Click Apply and Close.
Once the Jython
is included in our Java environment, we can now run Python scripts in Java. The PythonInterpreter
class is from Jython
to execute Python scripts and operations.
Here are the different methods from Jython
to run Python in Java:
Method | Description |
---|---|
setIn(PyObject) |
Used to set a Python object for the standard input stream. |
setIn(java.io.Reader) |
Used to set a Java IO Reader for the standard input stream. |
setIn(java.io.InputStream) |
Used to set a Java IO InputStream for the standard input stream. |
setOut(PyObject) |
Used to set a Python object for the standard output stream. |
setOut(java.io.Writer) |
Used to set a Java IO writer for the standard output stream. |
setOut(java,io.OutputStream) |
Used to set a Java IO OutputStream for the standard output stream. |
setErr(java.io.Writer) |
Used to set a Java IO writer for the standard error stream. |
setErr(PyObject) |
Used to set a Python object for the standard error stream. |
setErr(java.io.OutputStream) |
Used to set a Java IO OutputStream for the standard error stream. |
eval(String) |
Used to evaluate a Python string for Java. |
eval(PyObject) |
Used to evaluate a Python object for Java. |
exec(String) |
Used to execute a Python string in the local namespace. |
exec(PyObject) |
Used to execute a Python object in the local namespace. |
execfile(String filename) |
Used to execute a Python file in the local namespace. |
execfile(java.io.InputStream) |
Used to execute a Python InputStream in the local namespace. |
compile(String) |
Used to compile a Python string as a module of expression. |
compile(script, filename) |
Used to compile a Python script as a module of expression. |
set(String name, Object value) |
Used to set a variable of type object. |
set(String name, PyObject value) |
Used to set a variable of type PyObject . |
get(String) |
Used to get the value of a variable. |
get(String name, Classjavaclass) |
Used to get the value of a variable and return it as the instance of a given Java class. |
Now, we know about the methods from the Jython
projects. Let’s try to use some of them in our example: The script.py
file:
print("Hello, This is Delftstack.com! The Best Tutorial Site!, Message from Python")
The implementation of Jython:
import org.python.core.*;
import org.python.util.PythonInterpreter;
public class Example {
public static void main(String[] args) throws PyException {
PythonInterpreter Python_Interpreter = new PythonInterpreter();
System.out.println("Hello, This is Delftstack.com! The Best Tutorial Site!, Message from Java");
// Run a Python file
Python_Interpreter.execfile("C:\\Users\\Sheeraz\\script.py");
// Run Different Python operations
// Set a variable and print it using Python in java
Python_Interpreter.set("x", new PyInteger(100));
Python_Interpreter.exec("print x");
// Execute a sum
Python_Interpreter.exec("y = 25+45");
PyObject y = Python_Interpreter.get("y");
System.out.println("y: " + y);
}
}
The code above will execute a Python script from a file and run some Python operations using the methods from the above table. See output:
Hello, This is Delftstack.com! The Best Tutorial Site!, Message from Java
Hello, This is Delftstack.com! The Best Tutorial Site!, Message from Python
100
y: 70
Sheeraz is a Doctorate fellow in Computer Science at Northwestern Polytechnical University, Xian, China. He has 7 years of Software Development experience in AI, Web, Database, and Desktop technologies. He writes tutorials in Java, PHP, Python, GoLang, R, etc., to help beginners learn the field of Computer Science.
LinkedIn Facebook