How to Include an HTML File in Another HTML File
-
Use the jQuery
load()
Method to Include an HTML File in an HTML File - Use JavaScript to Include an HTML File in an HTML File
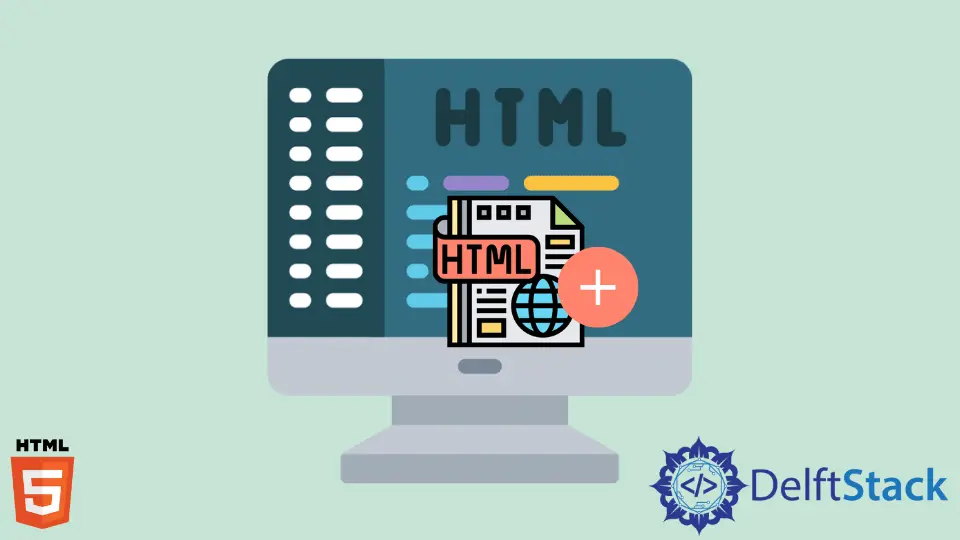
This article will introduce methods to include an HTML file in an HTML file.
Use the jQuery load()
Method to Include an HTML File in an HTML File
The jQuery load()
method allows us to load the data from the server. The data will be displayed in the selected container. We can use the load()
method to include another HTML file in the current HTML file. The syntax of the load()
method is as follows.
.load(url, data, callback);
The load()
method takes the URL to be loaded as the first parameter. It has two other parameters, data
and callback
function, which are optional. The parameter data
is the data to be sent to the server while processing the request. The callback
function will execute if the load()
method completes.
To run jQuery, we need to use the jQuery CDN in the script
tag in HTML. For example, go to CDN and choose the minified option on the latest version of jQuery. Afterward, copy the code and paste it into the index.html
file. Next, create a div
with id anotherContent
in HTML. Then, create a p
tag and write the text This is from index.html
. Create another HTML file named lake.html
and write a paragraph This is from lake.html
. In index.html
, write a jQuery function. Select the id anotherContent
and call the load()
method with lake.html
as the parameter.
When we run the file index.html
we can also see the text This is from lake.html
. However, we should run the file index.html
from an HTTP server. Cross-Origin Request will be blocked when we include another file (lake.html
in our case) in the current file using the local file
server. To eliminate this problem, we can create an HTTP server with the following python command from the directory of the index.html
file.
python3 -m SimpleHTTPServer 1337
Then, we can access our webpage from http://localhost:1337
. Thus, we can use jQuery to include another HTML file in the current HTML file.
Example Code:
<script src="https://code.jquery.com/jquery-3.6.0.min.js" integrity="sha256-/xUj+3OJU5yExlq6GSYGSHk7tPXikynS7ogEvDej/m4=" crossorigin="anonymous">
</script>
<script>
$(function(){
$("#anotherContent").load("lake.html");
});
</script>
<div id="anotherContent"></div>
<p>This is from index.html</p>
<p> This is from lake.html</p>
Output:
This is from lake.html
This is from index.html
Use JavaScript to Include an HTML File in an HTML File
We can use JavaScript to include HTML in an HTML file. The benefit of using this method using jQuery is that we do not have to load any of the jQuery files that make our file’s size smaller. We can use the template literals in JavaScript to write the HTML. We can achieve our goal by including the JavaScript file in our HTML file and writing the HTML in the JavaScript file.
For example, in the index.hmtl
file, link the lake.js
file using the src
attribute in the script
tag. In the body section, write the text This is from index.html
. In the lake.js
file, use document.write()
to write the HTML. Use the template literals to write the HTML inside the method. In other words, wrap the HTML content with the backticks. Write the p
tag inside the method and write the text This is HTML from lake.js
.
In the output section, we can see the texts from both files. Thus, we can include HTML in an HTML file using JavaScript.
Example Code:
<head>
<script src="lake.js "></script>
</head>
<body>
<p>This is from index.html</p>
</body>
document.write(`
<p>This is HTML from lake.js</p>
`);
Output:
This is HTML from lake.js
This is from index.html
Subodh is a proactive software engineer, specialized in fintech industry and a writer who loves to express his software development learnings and set of skills through blogs and articles.
LinkedIn