How to Convert HTML to JSON
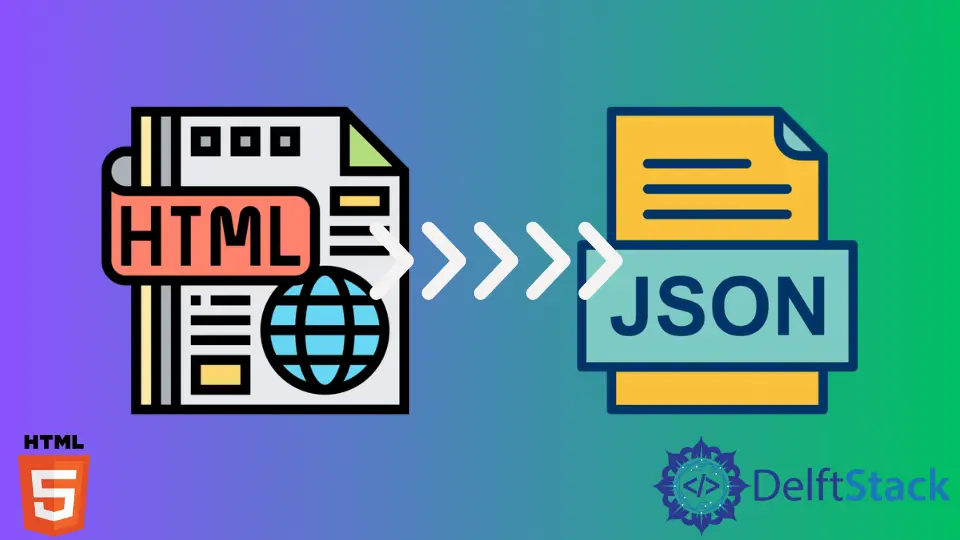
In the world of web development, data interchange formats play a crucial role in how information is shared and processed. One common task developers face is converting HTML to JSON. This transformation is essential for applications that need to manipulate data in a structured format.
In this tutorial, we will walk you through the process of converting HTML to JSON using Python. Whether you’re pulling data from a webpage or processing form submissions, understanding how to perform this conversion can save you time and enhance your projects. So, let’s dive into the methods that will help you achieve this efficiently.
Method 1: Using BeautifulSoup
BeautifulSoup is a powerful Python library that makes it easy to scrape and parse HTML documents. By using BeautifulSoup in conjunction with the built-in json
library, you can convert HTML data into a JSON format seamlessly.
First, you’ll need to install BeautifulSoup if you haven’t already. You can do this using pip:
pip install beautifulsoup4
Now, let’s take a look at the code that performs the conversion.
from bs4 import BeautifulSoup
import json
html_data = """
<html>
<head><title>Sample Page</title></head>
<body>
<h1>Welcome to My Page</h1>
<p>This is a sample paragraph.</p>
<ul>
<li>Item 1</li>
<li>Item 2</li>
</ul>
</body>
</html>
"""
soup = BeautifulSoup(html_data, 'html.parser')
data = {
'title': soup.title.string,
'heading': soup.h1.string,
'paragraph': soup.p.string,
'list_items': [li.string for li in soup.find_all('li')]
}
json_data = json.dumps(data, indent=4)
print(json_data)
Output:
{
"title": "Sample Page",
"heading": "Welcome to My Page",
"paragraph": "This is a sample paragraph.",
"list_items": [
"Item 1",
"Item 2"
]
}
In this method, we start by importing the necessary libraries and defining a sample HTML string. We then create a BeautifulSoup object to parse the HTML. Next, we extract relevant data such as the title, heading, paragraph, and list items. Finally, we convert this data into a JSON format using json.dumps()
, which creates a nicely formatted JSON string. This method is straightforward and effective for extracting structured data from HTML.
Method 2: Using lxml
Another powerful library for parsing HTML in Python is lxml. It’s known for its speed and efficiency, making it a great choice for large documents. Like BeautifulSoup, lxml can be used to extract data and convert it to JSON.
To get started with lxml, you need to install it first:
pip install lxml
Here’s how you can convert HTML to JSON using lxml:
from lxml import html
import json
html_data = """
<html>
<head><title>Sample Page</title></head>
<body>
<h1>Welcome to My Page</h1>
<p>This is a sample paragraph.</p>
<ul>
<li>Item 1</li>
<li>Item 2</li>
</ul>
</body>
</html>
"""
tree = html.fromstring(html_data)
data = {
'title': tree.find('.//title').text,
'heading': tree.find('.//h1').text,
'paragraph': tree.find('.//p').text,
'list_items': [li.text for li in tree.xpath('//li')]
}
json_data = json.dumps(data, indent=4)
print(json_data)
Output:
{
"title": "Sample Page",
"heading": "Welcome to My Page",
"paragraph": "This is a sample paragraph.",
"list_items": [
"Item 1",
"Item 2"
]
}
In this example, we import the lxml library and parse the same HTML string. We create an HTML tree structure using html.fromstring()
. By using XPath expressions, we can easily navigate through the document and extract the title, heading, paragraph, and list items. The extracted data is then converted to JSON format with json.dumps()
. This method is particularly useful when dealing with complex HTML structures and is optimized for performance.
Conclusion
Converting HTML to JSON is a valuable skill for developers, especially when dealing with web scraping or data interchange. In this tutorial, we explored two popular methods using Python libraries: BeautifulSoup and lxml. Both libraries provide simple and effective ways to extract data from HTML and convert it into a structured JSON format. By mastering these techniques, you can streamline your data processing tasks and enhance your web development projects. Remember to choose the method that best suits your project’s requirements.
FAQ
-
What is the purpose of converting HTML to JSON?
Converting HTML to JSON allows developers to manipulate and process data in a structured format, making it easier to work with in applications. -
Can I use other libraries to convert HTML to JSON?
Yes, besides BeautifulSoup and lxml, there are other libraries like html5lib and PyQuery that can also be used for parsing HTML and converting it to JSON. -
Is it necessary to have programming experience to convert HTML to JSON?
While some basic understanding of Python programming is helpful, the methods outlined in this tutorial are designed to be straightforward and accessible for beginners. -
Are there any limitations when using these libraries?
Each library has its strengths and weaknesses. BeautifulSoup is great for ease of use, while lxml is known for speed. Depending on the complexity of your HTML, you may need to choose accordingly. -
Can I convert large HTML documents to JSON?
Yes, both BeautifulSoup and lxml are capable of handling large documents, but performance may vary based on the library and the specific structure of the HTML.
Subodh is a proactive software engineer, specialized in fintech industry and a writer who loves to express his software development learnings and set of skills through blogs and articles.
LinkedIn