How to Add JQuery in HTML
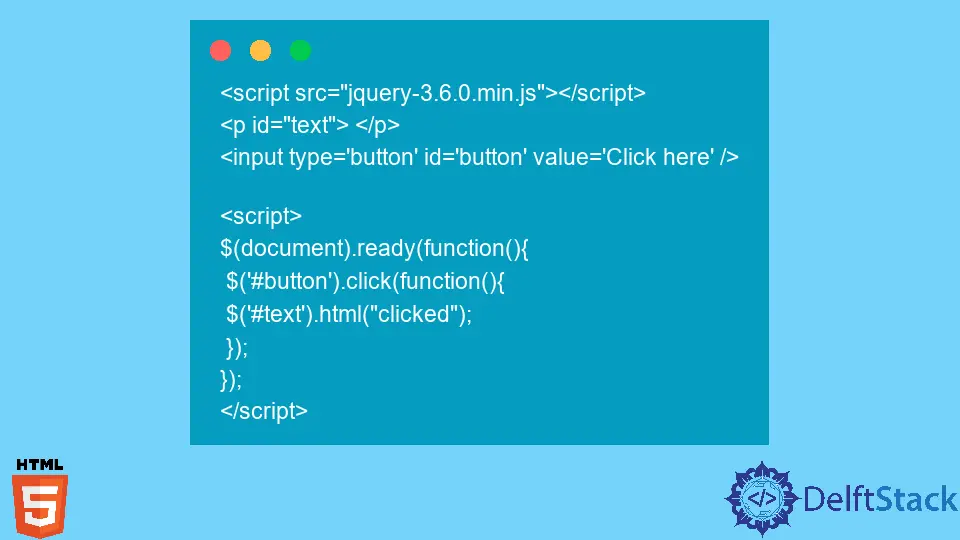
This article will guide you through adding or writing jQuery code on an HTML page.
Use the JQuery CDN to Include JQuery in HTML
JQeury is one of the most popular JavaScript libraries.
The library simplifies a lot of work in a website with features like DOM traversal and manipulation, CSS manipulation, event handling, animations, and AJAX calls. We can write less and do more using jQuery, which is the main advantage.
We can do operations with less code using jQuery rather than the regular JavaScript. Now, we will tackle how we can use jQuery on an HTML page.
The most common way of including jQuery in an HTML document is using the Content Delivery Network (CDN). A CDN is a network of servers that serves the requested content to the end-users by caching the content in the network location closer to the user.
In this way, the user will get served the requested data quickly and accurately. We can get the jQuery CDN from various sources like Google CDN and Microsoft CDN.
We can include the jQuery CDN URL in the script
tag and use jQuery in HTML. We can either write the jQuery in a separate .js
file and include it in the HTML file or write the jQuery inside the script
tag.
First, go to the JQuery CDN website and choose the latest stable version of the jQuery. For demonstration, we are using the uncompressed version.
Then the website will output the tag shown below.
<script src="https://code.jquery.com/jquery-3.6.0.js" integrity="sha256-H+K7U5CnXl1h5ywQfKtSj8PCmoN9aaq30gDh27Xc0jk=" crossorigin="anonymous"></script>
Next, copy the tag and place it in the HTML file. Then, create a p
tag with the id text
and do not write anything between the tags.
Below the p
tag, create a button with the id button
and write the text Click here
in the value
attribute. The HTML part is over, and now we will write the jQuery.
Inside a script
tag, write the ready()
jQuery method. Select the button
id and call the click()
method inside the method.
Again, inside the click()
method select the text
id and call the html()
method. Write the text clicked
as the html()
parameter.
In this section, we will learn about these various jQuery methods. The ready()
method contains all the other methods.
The other content inside the method will get executed when the DOM loads completely. Similarly, the click()
method executes when the selected element is clicked. The html()
method sets the content to the selected element.
In the example below, a text will appear saying clicked
when clicking the button. In this way, we can use jQuery in an HTML document using the CDN.
Example Code:
<script src="https://code.jquery.com/jquery-3.6.0.js" integrity="sha256-H+K7U5CnXl1h5ywQfKtSj8PCmoN9aaq30gDh27Xc0jk=" crossorigin="anonymous"></script>
<p id="text"> </p>
<input type='button' id='button' value='Click here' />
<script>
$(document).ready(function(){
$('#button').click(function(){
$('#text').html("clicked");
});
});
</script>
Download the JQuery and Include it in HTML
The other way of writing jQuery in HTML is downloading the jQuery file and including it in the HTML file.
We can download the file from the link here. We will write jQuery code in HTML after we include the downloaded .js
file in the script
tag.
For example, download the jQuery file from the link given above. Next, in the HTML file, write the file path of the jQuery file in the src
attribute of the script
tag.
This is the only change we have to make from the example in the method above. We will use the same HTML and jQuery code for the demonstration.
When we click the button, it will result in the text clicked
as in the example above. In this way, we can download the jQuery file and include it in HTML.
Example Code:
<script src="jquery-3.6.0.min.js"></script>
<p id="text"> </p>
<input type='button' id='button' value='Click here' />
<script>
$(document).ready(function(){
$('#button').click(function(){
$('#text').html("clicked");
});
});
</script>
Sushant is a software engineering student and a tech enthusiast. He finds joy in writing blogs on programming and imparting his knowledge to the community.
LinkedIn