The Zero Value Nil in Golang
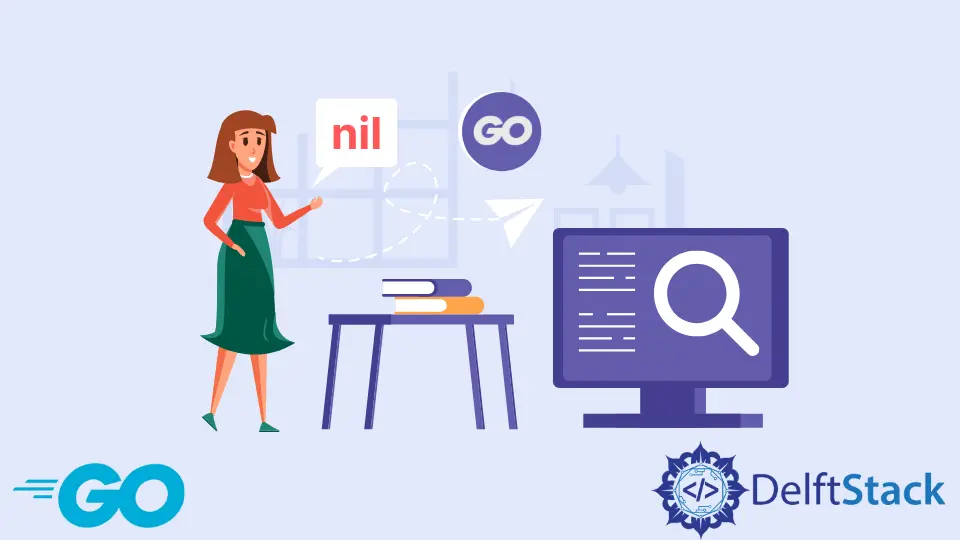
nil
is a zero value in the Go programming language, a well-known and significant predefined identifier. It is the literal representation of several different types of zero values.
Many novice Go programmers who have worked in other popular languages may consider nil
the equivalent of null
(or NULL
) in other languages. This is partially correct, but there are several distinctions between nil
in Go and null
(or NULL
) in other languages.
We’ll look at a few instances to see what nil
means in Golang.
Check the Size of nil
With Different Types in Golang
The memory layouts of all types of values are always the same. The type’s nil
values are not exceptions (assume zero value as nil
).
A nil
value’s size is always the same as the sizes of non-nil
values with the same type as the nil
value. However, nil
values of various types may have different sizes.
Code:
package main
import (
"fmt"
"unsafe"
)
func main() {
var p *struct{} = nil
fmt.Println(unsafe.Sizeof(p))
var s []int = nil
fmt.Println(unsafe.Sizeof(s))
var m map[int]bool = nil
fmt.Println(unsafe.Sizeof(m))
var c chan string = nil
fmt.Println(unsafe.Sizeof(c))
var f func() = nil
fmt.Println(unsafe.Sizeof(f))
var i interface{} = nil
fmt.Println(unsafe.Sizeof(i))
}
Output:
8
24
8
8
8
16
Return nil
String in Golang
The pointer variable type that refers to a string value is string
. A pointer’s zero value is nil
.
Code:
package main
import (
"fmt"
)
func main() {
var data *string = nil
if data == nil {
fmt.Println(data)
}
}
Output:
<nil>
In this following example, we will check if nil == nil
in Golang.
Code:
package main
import (
"fmt"
)
func main() {
var i *int = nil
var j *int = nil
if i == j {
fmt.Println("equal")
} else {
fmt.Println("no")
}
}
Output:
equal