How to Send a JSON String in a POST Request in Go
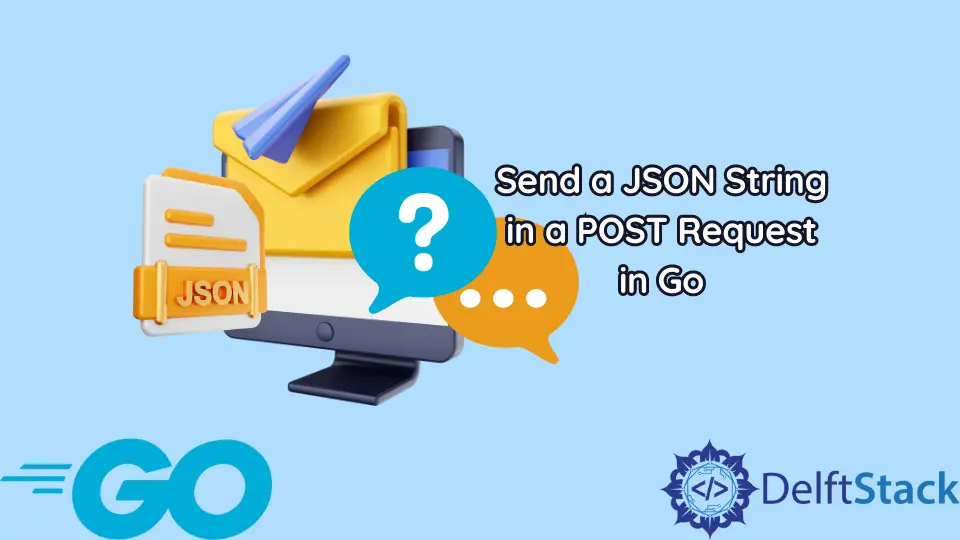
The JavaScript Object Notation (JSON) is a commonly used data transmission format in web development. It is simple to use and understand.
You can create a JSON POST
request with the Go language, but you need to import several Go packages. The net/HTTP
package includes good HTTP client and server support.
The JSON
package in Go also provides JSON encoding and decoding.
In this tutorial, you’ll learn how to use the Go language to perform JSON POST requests. In this tutorial, you’ll learn how to use the Go language to deliver a JSON string as a POST request.
Send a JSON String in a POST Request in Go
A basic JSON file containing a list of Courses and Paths is shown below.
{
"Courses": [
"Golang",
"Python"
],
"Paths": [
"Coding Interviews",
"Data Structure"
]
}
The code below shows how to submit the USER JSON object to the service reqres.in
to construct a user request.
package main
import (
"bytes"
"encoding/json"
"fmt"
"io/ioutil"
"net/http"
)
type User struct {
Name string `json:"name"`
Job string `json:"job"`
}
func main() {
user := User{
Name: "Jay Singh",
Job: "Golang Developer",
}
body, _ := json.Marshal(user)
resp, err := http.Post("https://reqres.in/api/users", "application/json", bytes.NewBuffer(body))
if err != nil {
panic(err)
}
defer resp.Body.Close()
if resp.StatusCode == http.StatusCreated {
body, err := ioutil.ReadAll(resp.Body)
if err != nil {
panic(err)
}
jsonStr := string(body)
fmt.Println("Response: ", jsonStr)
} else {
fmt.Println("Get failed with error: ", resp.Status)
}
}
Output:
Response: {
"name":"Jay Singh",
"job":"Golang Developer",
"id":"895",
"createdAt":"2022-04-04T10:46:36.892Z"
}
Sending a JSON string in a POST request in Golang
Here is the simple JSON code.
{
"StudentName": "Jay Singh",
"StudentId" : "192865782",
"StudentGroup": "Computer Science"
}
package main
import (
"bytes"
"encoding/json"
"fmt"
"io/ioutil"
"net/http"
)
type StudentDetails struct {
StudentName string `json:"StudentName"`
StudentId string `json:"StudentId"`
StudentGroup string `json:"StudentGroup"`
}
func main() {
studentDetails := StudentDetails{
StudentName: "Jay Singh",
StudentId: "192865782",
StudentGroup: "Computer Science",
}
body, _ := json.Marshal(studentDetails)
resp, err := http.Post("<https://reqres.in/api/users>", "application/json", bytes.NewBuffer(body))
if err != nil {
panic(err)
}
defer resp.Body.Close()
if resp.StatusCode == http.StatusCreated {
body, err := ioutil.ReadAll(resp.Body)
if err != nil {
panic(err)
}
jsonStr := string(body)
fmt.Println("Response: ", jsonStr)
} else {
fmt.Println("Get failed with error: ", resp.Status)
}
}
Output:
Response: {
"StudentName":"Deven Rathore",
"StudentId":"170203065",
"StudentGroup":"Computer Science",
"id":"868",
"createdAt":"2022-04-04T12:35:03.092Z"
}