Lambda Expression in Golang
- Understanding Lambda Expressions in Golang
- Using Lambda Expressions as Function Arguments
- Closures in Golang
- Conclusion
- FAQ
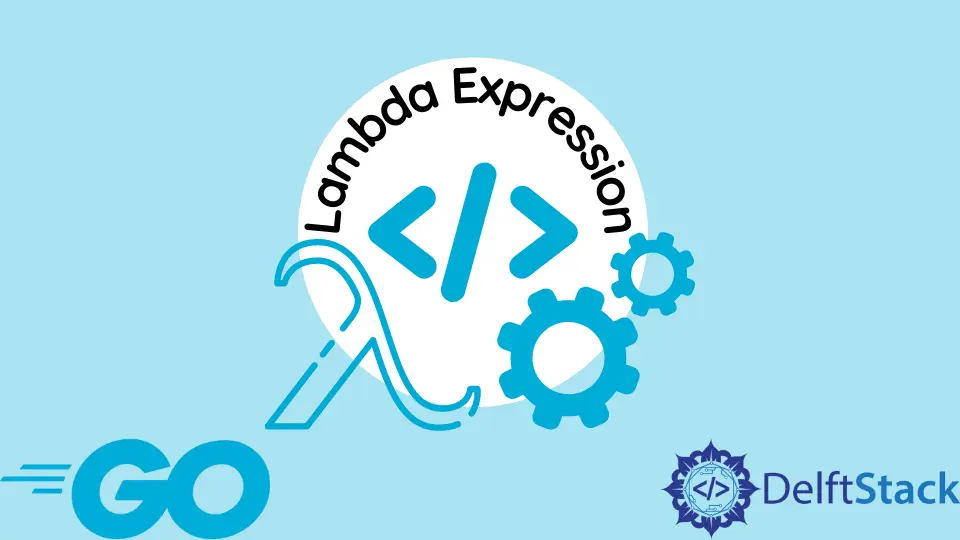
Lambda expressions, also known as anonymous functions, are a powerful feature in programming languages, including Golang. They allow developers to create functions without explicitly naming them, making code cleaner and more modular.
In this tutorial, we’ll dive into the concept of lambda expressions in Golang, exploring how to create and use them effectively. Whether you’re a beginner or an experienced programmer, understanding lambda expressions can significantly enhance your coding skills and improve your ability to write concise and efficient code. So, let’s get started and unlock the potential of lambda expressions in Golang!
Understanding Lambda Expressions in Golang
Lambda expressions in Golang are essentially functions that can be defined inline and can be assigned to variables or passed as arguments to other functions. This feature is particularly useful for creating short-lived functions that don’t require a formal definition. Here’s a simple example to illustrate how to create a lambda expression in Golang.
package main
import "fmt"
func main() {
add := func(a int, b int) int {
return a + b
}
result := add(5, 3)
fmt.Println(result)
}
In this example, we define a lambda expression that adds two integers. The function is assigned to the variable add
, which we can then call just like a regular function. The output of this code will be the sum of 5 and 3.
Output:
8
The flexibility of lambda expressions allows you to create functions on-the-fly, which can be particularly useful in scenarios like sorting or filtering slices. By using anonymous functions, you can keep your code organized and enhance readability.
Using Lambda Expressions as Function Arguments
One of the most powerful aspects of lambda expressions is their ability to be passed as arguments to other functions. This feature can be particularly useful when working with higher-order functions. Let’s take a look at a practical example where we use a lambda expression as an argument to filter a slice of integers.
package main
import "fmt"
func filter(numbers []int, condition func(int) bool) []int {
var result []int
for _, num := range numbers {
if condition(num) {
result = append(result, num)
}
}
return result
}
func main() {
numbers := []int{1, 2, 3, 4, 5, 6}
evenNumbers := filter(numbers, func(n int) bool {
return n%2 == 0
})
fmt.Println(evenNumbers)
}
In this code, we define a filter
function that takes a slice of integers and a lambda expression as its parameters. The lambda expression defines the condition for filtering. In this case, we want to extract even numbers from the slice. The output will be a new slice containing only the even integers.
Output:
[2 4 6]
Using lambda expressions in this way makes your functions more reusable and flexible. You can easily change the filtering condition without modifying the core logic of your filter
function.
Closures in Golang
Closures are another fascinating aspect of lambda expressions in Golang. A closure is a function that captures the variables from its surrounding environment. This means that the function retains access to those variables even after the outer function has finished executing. Let’s see an example of closures in action.
package main
import "fmt"
func makeCounter() func() int {
count := 0
return func() int {
count++
return count
}
}
func main() {
counter := makeCounter()
fmt.Println(counter())
fmt.Println(counter())
fmt.Println(counter())
}
In this example, we define a makeCounter
function that returns a lambda expression. The lambda expression increments and returns the count
variable each time it is called. Even after makeCounter
has completed execution, the returned function retains access to the count
variable.
Output:
1
2
3
Closures are incredibly useful for maintaining state in your functions without using global variables. They allow for encapsulation and can help prevent naming conflicts in larger programs.
Conclusion
Lambda expressions in Golang provide a powerful way to create concise and flexible code. By understanding how to use them as standalone functions, as arguments to other functions, and as closures, you can significantly enhance your programming capabilities. Whether you’re filtering data, creating callbacks, or maintaining state, lambda expressions can make your code cleaner and more efficient. With this knowledge, you’re now equipped to implement lambda expressions in your own Golang projects. Happy coding!
FAQ
-
What are lambda expressions in Golang?
Lambda expressions, or anonymous functions, are functions defined without a name, allowing for more concise and modular code. -
How do I pass a lambda expression as an argument in Golang?
You can pass a lambda expression to a function by defining it inline as a parameter. -
What is a closure in Golang?
A closure is a function that captures and retains access to variables from its surrounding context, even after the outer function has executed. -
Can lambda expressions be stored in variables?
Yes, you can assign lambda expressions to variables and invoke them like regular functions. -
Are lambda expressions in Golang similar to those in other programming languages?
Yes, while the syntax may vary, the concept of lambda expressions is consistent across many programming languages.