How to Convert String to Byte Array in Golang
-
Using the
byte()
Function to Convert String to Byte Array in Golang -
Using the
[]byte(strToConvert)
Method to Convert String to Byte Array in Golang -
Using the
copy()
Function to Convert String to Byte Array in Golang - Conclusion
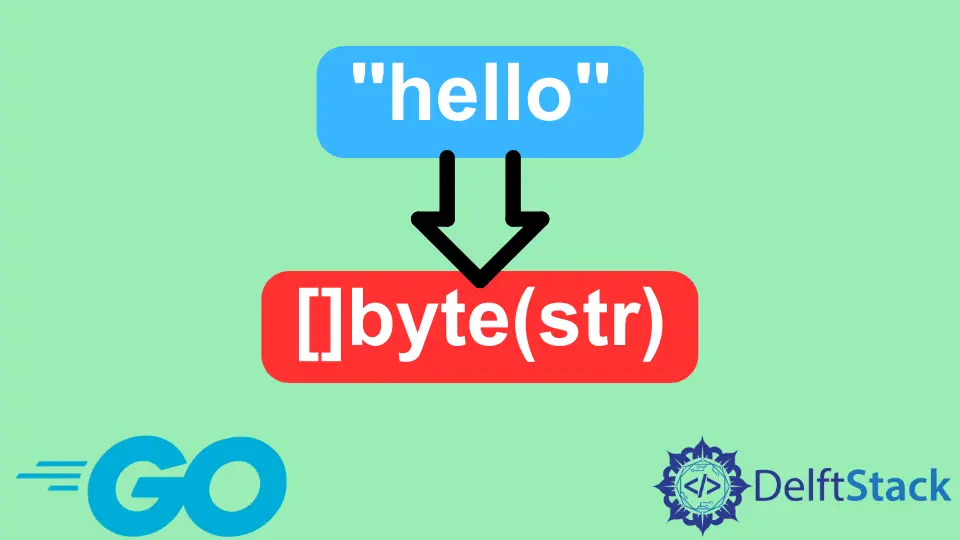
In Golang, when you convert a string to a byte array, what you get is a slice containing the string’s bytes. It’s crucial to grasp that in Go, a string is essentially a read-only byte slice.
Regardless of whether your string represents Unicode text, UTF-8 text, or follows any other specific format, it can be seen as a sequence of bytes. This is a fundamental concept to keep in mind as you work with strings in Go.
In this article, we’ll discuss the methods on how to convert string to byte array in Go.
Using the byte()
Function to Convert String to Byte Array in Golang
Use the byte()
function in Golang to convert a String to a Byte array.
A byte is an unsigned 8-bit integer. An array is returned by the byte()
method, which takes a string as an input.
When you make a string, you’re actually making an array of bytes. As a result, you can read individual bytes as if they were an array.
Basic Syntax:
data := []byte("hello boss!")
In this syntax, we create a byte slice named data
, aiming to convert the string "hello boss!"
into a byte array. Our intention is to transform the string into its byte representation using the []byte
type conversion.
The data variable now stores a byte slice with ASCII or Unicode values corresponding to each character in the original string.
The following code, for example, iterates through every byte in a string and outputs it as both a string and a byte.
Code 1:
package main
import "fmt"
func main() {
str := "hello boss!"
data := []byte(str)
fmt.Println(data)
}
In this code, we declare a string variable str
with the value "hello boss!"
. Next, we use the []byte
conversion to transform the string into a byte slice named data.
Finally, we print the resulting byte slice using the fmt.Println
function.
Output:
[104 101 108 108 111 32 98 111 115 115 33]
The output of our program is a representation of the bytes corresponding to each character in the string, presented within square brackets: [104 101 108 108 111 32 98 111 115 115 33]
.
Let’s have another example.
Code 2:
package main
import "fmt"
func main() {
var s string = "hello boss!"
fmt.Println(s)
var b []byte
b = []byte(s)
fmt.Println(b)
for i := range b {
fmt.Println(string(b[i]))
}
s = string(b)
fmt.Println(s)
}
In the code, we initialize a string variable s
with the value "hello boss!"
. We then print the original string using fmt.Println
.
Next, we create a byte slice b
by directly converting the string into a slice of bytes using the []byte
conversion. Subsequently, we print the byte slice, displaying the byte values associated with each character in the string.
We further iterate through the byte slice using a for
loop, printing each individual character as a string. Finally, we convert the byte slice back to a string and print it again.
Output:
hello boss!
[104 101 108 108 111 32 98 111 115 115 33]
h
e
l
l
o
b
o
s
s
!
hello boss!
The output of our program showcases the original string, its byte representation, each character individually, and the string reconstructed from the byte slice.
This method is concise and commonly used in Golang for simple conversions between strings and byte slices. It’s particularly useful when you don’t need to perform additional operations on the byte data and want a straightforward representation of the string as a slice of bytes.
Using the []byte(strToConvert)
Method to Convert String to Byte Array in Golang
Using []byte(strToConvert)
is a method to convert a string to a byte array or, more precisely, a byte slice. The expression []byte
is a type conversion that transforms a string into its corresponding byte representation.
This conversion creates a new byte slice where each element corresponds to the ASCII or Unicode value of the characters in the original string.
Basic Syntax:
strToConvert := "hello boss!"
byteString := []byte(strToConvert)
In the syntax, we initialize a variable, strToConvert
, with the value "hello boss!"
. We then create a new variable, byteString
, employing the []byte
type conversion on strToConvert
. This expresses our intent to convert the string into a byte slice.
Lastly, the resulting byteString
variable now holds the byte representation of the initial string.
In this example, we will be using []byte(strToConvert)
to convert string to byte array in Golang.
Code:
package main
import (
"fmt"
)
func main() {
var strToConvert string
strToConvert = "hello boss!"
byteString := []byte(strToConvert)
fmt.Println(byteString)
}
In this code, we declare a string variable strToConvert
and assign it the value "hello boss!"
. Subsequently, we utilize the []byte
type conversion to convert the string into a byte slice named byteString
.
Finally, we print the resulting byte slice using the fmt.Println
function.
Output:
[104 101 108 108 111 32 98 111 115 115 33]
The output of our program is a representation of the byte values corresponding to each character in the original string, enclosed within square brackets: [104 101 108 108 111 32 98 111 115 115 33]
.
Using the copy()
Function to Convert String to Byte Array in Golang
The copy()
function in Golang converts a string to a byte array that involves copying the characters of the string into a pre-allocated byte array. This method allows for an efficient and controlled conversion process.
Basic Syntax:
copy(byteString, strToConvert)
The syntax uses the copy()
function to copy the elements of one slice (strToConvert
) into another slice (byteString
).
In this example, the string will be copied to a byte array using the copy()
function. As a result, we declared a byte array and copied the string to it using the copy
function.
Code:
package main
import (
"fmt"
)
func main() {
var strToConvert string
strToConvert = "hello boss!"
byteString := make([]byte, len(strToConvert))
copy(byteString, strToConvert)
fmt.Println(byteString)
}
In the code, we begin by declaring a string variable named strToConvert
and assigning it the value "hello boss!"
. Following this, we create a byte slice named byteString
using the make()
function, with the length determined by the size of the original string.
We then employ the copy()
function to copy the characters from the string into the newly created byte slice. Finally, we print the resulting byte slice using the fmt.Println
function.
Output:
[104 101 108 108 111 32 98 111 115 115 33]
The output of our program is a representation of the byte values associated with each character in the initial string, enclosed within square brackets: [104 101 108 108 111 32 98 111 115 115 33]
.
The combination of make()
and copy()
facilitates a precise and controlled process for handling string-to-byte conversions.
Conclusion
In Golang, converting a string to a byte array involves understanding that a string is essentially a read-only byte slice. This article explores various methods for this conversion, emphasizing the fundamental concept of representing strings as sequences of bytes in Golang.
The first method involves using the byte()
function, treating a string as an array of bytes, and providing a straightforward means of conversion. Direct type conversion, achieved through []byte(strToConvert)
, creates a byte slice where each element corresponds to the ASCII or Unicode value of the original string’s characters, offering simplicity in conversion.
The article also introduces the controlled and efficient conversion process using the copy()
function. By copying string characters into a pre-allocated byte array, coupled with the make()
function, developers gain precision in handling string-to-byte conversions.
Lastly, the direct type conversion using []byte
is presented as a versatile solution, demonstrated in a concise code snippet. This method offers simplicity in manipulating byte data while providing an effective means of converting strings to byte slices.
In summary, Golang provides diverse methods for converting strings to byte arrays, allowing developers to choose approaches based on their specific needs for simplicity, control, or versatility in string manipulations.