Function Pointer in Go
- Declaration of Pointers in Go
- Initialize a Pointer in Go
- Use Uninitialized Pointer in Go
-
Use the Shorthand
:=
Syntax for Pointers in Go - Change the Values of a Variable Using a Pointer in Go
-
Create Pointers Using the
new()
Function in Go - Pass a Pointer as an Argument to a Function in Go
- Pass an Address of a Variable as an Argument to a Function in Go
- Return a Pointer From a Function in Go
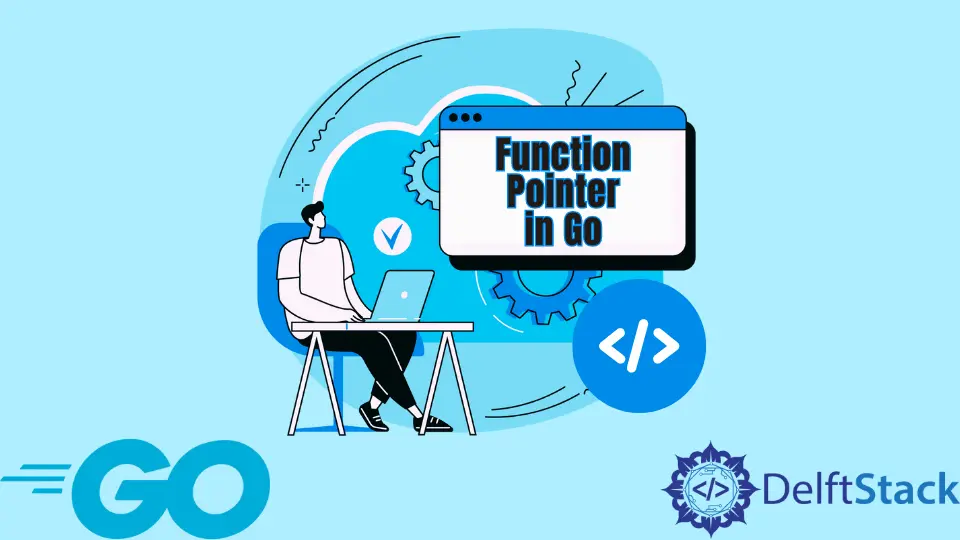
A pointer in the Go language is a special-purpose variable used to store the memory addresses of other variables and points where the memory is located. The value stored at that memory location can also be accessed.
Declaration of Pointers in Go
var pointer_name *Data_Type
Operators Used in Function Pointers
*
- It is a dereferencing operator that declares a pointer variable. The value stored at the dereferenced address can be accessed using it.&
- It is an address operator used to give the address of a variable.
Initialize a Pointer in Go
package main
import "fmt"
func main() {
var myVariable int = 2
var myPointer *int
myPointer = &myVariable
fmt.Println("The value stored in 'myVariable' = ", myVariable)
fmt.Println("The address of 'myVariable' = ", &myVariable)
fmt.Println("The value stored in 'myPointer' = ", myPointer)
fmt.Println("The value pointed by 'myPointer' after dereferencing = ", *myPointer)
}
Output:
The value stored in 'myVariable' = 2
The address of 'myVariable' = 0xc000090020
The value stored in 'myPointer' = 0xc000090020.
The value pointed by 'myPointer' after dereferencing = 2
Note that the value stored in the myPointer
variable is the variable myVariable
address.
Use Uninitialized Pointer in Go
package main
import "fmt"
func main() {
var myPointer *int
fmt.Println("The value stored in 'myPointer' = ", myPointer)
}
Output:
The value stored in 'myPointer' = <nil>
Note that an uninitialized pointer will have a default nil
value.
Use the Shorthand :=
Syntax for Pointers in Go
package main
import "fmt"
func main() {
myVariable := 2
myPointer := &myVariable
fmt.Println("The value stored in 'myVariable' = ", myVariable)
fmt.Println("The address of 'myVariable' = ", &myVariable)
fmt.Println("The value stored in 'myPointer' = ", myPointer)
fmt.Println("The value pointed by 'myPointer' after dereferencing = ", *myPointer)
}
Output:
The value stored in 'myVariable' = 2
The address of 'myVariable' = 0xc000090020
The value stored in 'myPointer' = 0xc000090020
The value pointed by 'myPointer' after dereferencing = 2
Note that if you have specified the data type with the pointer declaration, then you can use the pointer only to handle the memory address of that specified data type variable.
The :=
operator automatically recognizes the data type and makes sure that the pointer created can handle the same data type.
Change the Values of a Variable Using a Pointer in Go
package main
import "fmt"
func main() {
myVariable := 2
myPointer := &myVariable
fmt.Println("The value stored in 'myVariable' = ", myVariable)
fmt.Println("The address of 'myVariable' = ", &myVariable)
fmt.Println("The value stored in 'myPointer' = ", myPointer)
fmt.Println("The value pointed by 'myPointer' after dereferencing = ", *myPointer)
*myPointer = 5
fmt.Println("The updated value pointed by 'myPointer' after dereferencing = ", *myPointer)
fmt.Println("The updated value stored in 'myVariable' = ", myVariable)
}
Output:
The value stored in 'myVariable' = 2
The address of 'myVariable' = 0xc000016058
The value stored in 'myPointer' = 0xc000016058
The value pointed by 'myPointer' after dereferencing = 2
The updated value pointed by 'myPointer' after dereferencing = 5
The updated value stored in 'myVariable' = 5
Note that you can change the pointer’s value instead of assigning a new value to the variable. The value changed using a pointer is also reflected in the original value.
Create Pointers Using the new()
Function in Go
package main
import "fmt"
func main() {
var myPointer = new(int)
*myPointer = 2
fmt.Println("The value stored in 'myPointer' = ", myPointer)
fmt.Println("The value pointed by 'myPointer' after dereferencing = ", *myPointer)
}
Output:
The value stored in 'myPointer' = 0xc000016058
The value pointed by 'myPointer' after dereferencing = 2
Note that using the new()
function, you can directly create a pointer to an integer.
Pass a Pointer as an Argument to a Function in Go
package main
import "fmt"
func myFunction(myVariable *int) {
*myVariable = 5
}
func main() {
var myVariable = 2
fmt.Printf("The value of myVariable before function call is: %d\n", myVariable)
var myPointer *int = &myVariable
myFunction(myPointer)
fmt.Printf("The value of myVariable after function call is: %d\n", myVariable)
}
Output:
The value of myVariable before function call is: 2
The value of myVariable after function call is: 5
Note that the above-created function changed the variable myVariable
value. The value changed in the function is reflected outside the function because the pointer directly references the memory location where the variable is stored.
Pass an Address of a Variable as an Argument to a Function in Go
package main
import "fmt"
func myFunction(myVariable *int) {
*myVariable = 5
}
func main() {
var myVariable = 2
fmt.Printf("The value of myVariable before function call is: %d\n", myVariable)
myFunction(&myVariable)
fmt.Printf("The value of myVariable after function call is: %d\n", myVariable)
}
Output:
The value of myVariable before function call is: 2
The value of myVariable after function call is: 5
Note that the above-created function changed the variable myVariable
value. The value changed in the function is reflected outside the function.
The change is made to the memory location where the variable is stored. This is an alternative method for passing a pointer to a function.
Return a Pointer From a Function in Go
package main
import "fmt"
func main() {
displayText := display()
fmt.Println("Hello,", *displayText)
}
func display() *string {
myMessage := "World!"
return &myMessage
}
Output:
Hello, World!
Note that the above-created function will return a pointer pointing to the string myMessage
. The value is then dereferenced in the main
function.
Musfirah is a student of computer science from the best university in Pakistan. She has a knack for programming and everything related. She is a tech geek who loves to help people as much as possible.
LinkedIn