Puntero de función en Go
- Declaración de punteros en Go
- Inicializar un puntero en Go
- Usar puntero no inicializado en Go
-
Use la sintaxis abreviada
:=
para punteros en Go - Cambiar los valores de una variable usando un puntero en Go
-
Crear punteros utilizando la función
nuevo()
en Go - Pasar un puntero como argumento a una función en Go
- Pasar una dirección de una variable como argumento a una función en Go
- Devolver un puntero desde una función en Go
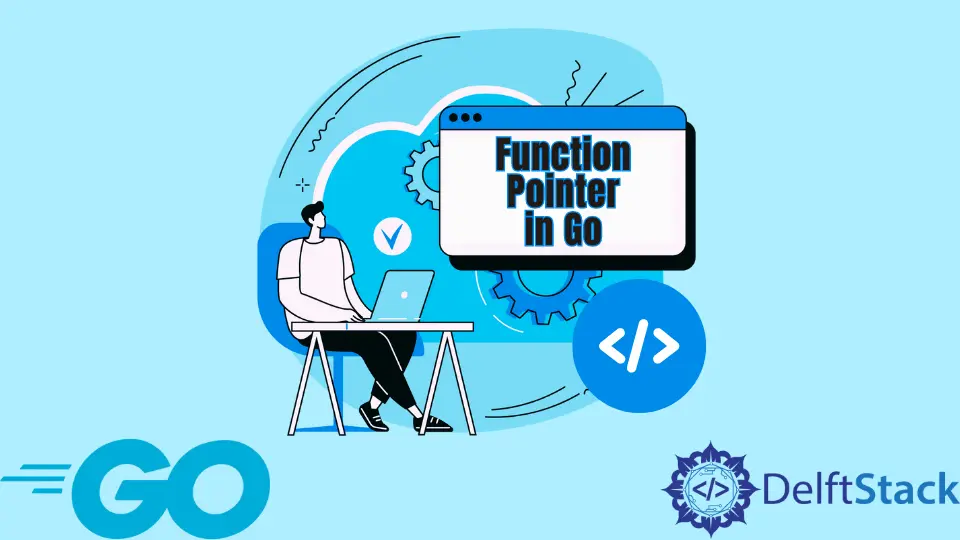
Un puntero en el lenguaje Go es una variable de propósito especial que se utiliza para almacenar las direcciones de memoria de otras variables y puntos donde se encuentra la memoria. También se puede acceder al valor almacenado en esa ubicación de memoria.
Declaración de punteros en Go
var pointer_name *Data_Type
Operadores utilizados en punteros de función
*
- Es un operador de desreferenciación que declara una variable puntero. Se puede acceder al valor almacenado en la dirección desreferenciada usándolo.&
- Es un operador de dirección que se utiliza para dar la dirección de una variable.
Inicializar un puntero en Go
package main
import "fmt"
func main() {
var myVariable int = 2
var myPointer *int
myPointer = &myVariable
fmt.Println("The value stored in 'myVariable' = ", myVariable)
fmt.Println("The address of 'myVariable' = ", &myVariable)
fmt.Println("The value stored in 'myPointer' = ", myPointer)
fmt.Println("The value pointed by 'myPointer' after dereferencing = ", *myPointer)
}
Producción :
The value stored in 'myVariable' = 2
The address of 'myVariable' = 0xc000090020
The value stored in 'myPointer' = 0xc000090020.
The value pointed by 'myPointer' after dereferencing = 2
Tenga en cuenta que el valor almacenado en la variable myPointer
es la dirección de la variable myVariable
.
Usar puntero no inicializado en Go
package main
import "fmt"
func main() {
var myPointer *int
fmt.Println("The value stored in 'myPointer' = ", myPointer)
}
Producción :
The value stored in 'myPointer' = <nil>
Tenga en cuenta que un puntero no inicializado tendrá un valor predeterminado nil
.
Use la sintaxis abreviada :=
para punteros en Go
package main
import "fmt"
func main() {
myVariable := 2
myPointer := &myVariable
fmt.Println("The value stored in 'myVariable' = ", myVariable)
fmt.Println("The address of 'myVariable' = ", &myVariable)
fmt.Println("The value stored in 'myPointer' = ", myPointer)
fmt.Println("The value pointed by 'myPointer' after dereferencing = ", *myPointer)
}
Producción :
The value stored in 'myVariable' = 2
The address of 'myVariable' = 0xc000090020
The value stored in 'myPointer' = 0xc000090020
The value pointed by 'myPointer' after dereferencing = 2
Tenga en cuenta que si ha especificado el tipo de datos con la declaración del puntero, puede usar el puntero solo para manejar la dirección de memoria de esa variable de tipo de datos especificada.
El operador :=
reconoce automáticamente el tipo de datos y se asegura de que el puntero creado pueda manejar el mismo tipo de datos.
Cambiar los valores de una variable usando un puntero en Go
package main
import "fmt"
func main() {
myVariable := 2
myPointer := &myVariable
fmt.Println("The value stored in 'myVariable' = ", myVariable)
fmt.Println("The address of 'myVariable' = ", &myVariable)
fmt.Println("The value stored in 'myPointer' = ", myPointer)
fmt.Println("The value pointed by 'myPointer' after dereferencing = ", *myPointer)
*myPointer = 5
fmt.Println("The updated value pointed by 'myPointer' after dereferencing = ", *myPointer)
fmt.Println("The updated value stored in 'myVariable' = ", myVariable)
}
Producción :
The value stored in 'myVariable' = 2
The address of 'myVariable' = 0xc000016058
The value stored in 'myPointer' = 0xc000016058
The value pointed by 'myPointer' after dereferencing = 2
The updated value pointed by 'myPointer' after dereferencing = 5
The updated value stored in 'myVariable' = 5
Tenga en cuenta que puede cambiar el valor del puntero en lugar de asignar un nuevo valor a la variable. El valor modificado con un puntero también se refleja en el valor original.
Crear punteros utilizando la función nuevo()
en Go
package main
import "fmt"
func main() {
var myPointer = new(int)
*myPointer = 2
fmt.Println("The value stored in 'myPointer' = ", myPointer)
fmt.Println("The value pointed by 'myPointer' after dereferencing = ", *myPointer)
}
Producción :
The value stored in 'myPointer' = 0xc000016058
The value pointed by 'myPointer' after dereferencing = 2
Tenga en cuenta que al utilizar la función nuevo()
, puede crear directamente un puntero a un número entero.
Pasar un puntero como argumento a una función en Go
package main
import "fmt"
func myFunction(myVariable *int) {
*myVariable = 5
}
func main() {
var myVariable = 2
fmt.Printf("The value of myVariable before function call is: %d\n", myVariable)
var myPointer *int = &myVariable
myFunction(myPointer)
fmt.Printf("The value of myVariable after function call is: %d\n", myVariable)
}
Producción :
The value of myVariable before function call is: 2
The value of myVariable after function call is: 5
Tenga en cuenta que la función creada anteriormente cambió el valor de la variable myVariable
. El valor cambiado en la función se refleja fuera de la función porque el puntero hace referencia directamente a la ubicación de la memoria donde se almacena la variable.
Pasar una dirección de una variable como argumento a una función en Go
package main
import "fmt"
func myFunction(myVariable *int) {
*myVariable = 5
}
func main() {
var myVariable = 2
fmt.Printf("The value of myVariable before function call is: %d\n", myVariable)
myFunction(&myVariable)
fmt.Printf("The value of myVariable after function call is: %d\n", myVariable)
}
Producción :
The value of myVariable before function call is: 2
The value of myVariable after function call is: 5
Tenga en cuenta que la función creada anteriormente cambió el valor de la variable myVariable
. El valor cambiado en la función se refleja fuera de la función.
El cambio se realiza en la ubicación de memoria donde se almacena la variable. Este es un método alternativo para pasar un puntero a una función.
Devolver un puntero desde una función en Go
package main
import "fmt"
func main() {
displayText := display()
fmt.Println("Hello,", *displayText)
}
func display() *string {
myMessage := "World!"
return &myMessage
}
Producción :
Hello, World!
Tenga en cuenta que la función creada anteriormente devolverá un puntero que apunta a la cadena myMessage
. A continuación, el valor se desreferencia en la función main
.
Musfirah is a student of computer science from the best university in Pakistan. She has a knack for programming and everything related. She is a tech geek who loves to help people as much as possible.
LinkedIn