How to Composite Literals in Go
- Understanding Composite Literals
- Using Composite Literals with Arrays
- Using Composite Literals with Slices
- Using Composite Literals with Maps
- Using Composite Literals with Structs
- Conclusion
- FAQ
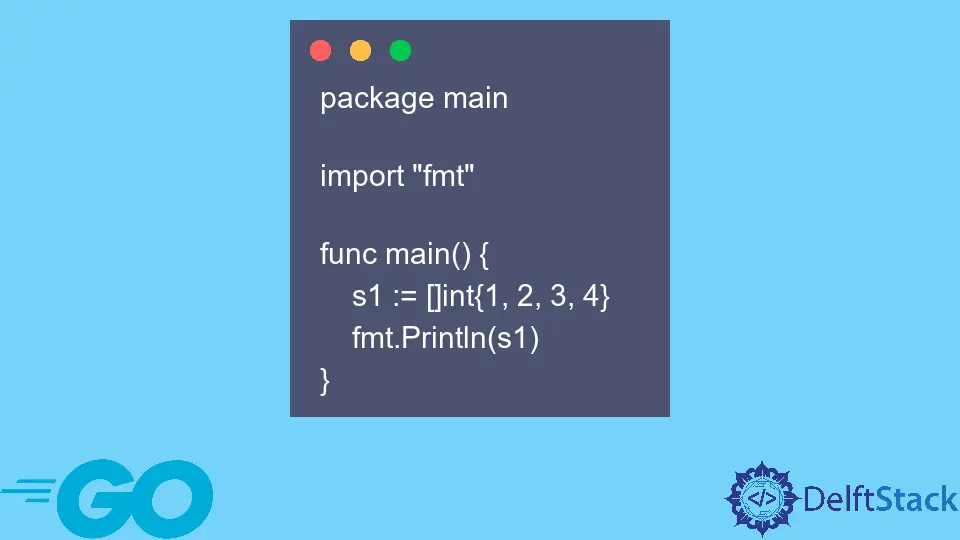
Composite literals in Go are a powerful feature that allows you to create complex data structures quickly and efficiently. Whether you’re working with arrays, slices, maps, or structs, understanding how to use composite literals can enhance your coding experience and improve performance.
This tutorial will walk you through the various types of composite literals in Go, explaining their syntax and offering practical examples. By the end of this guide, you’ll have a solid grasp of how to leverage composite literals in your Go applications, allowing you to write cleaner and more maintainable code.
Understanding Composite Literals
Composite literals allow you to create and initialize complex types in Go, such as arrays, slices, maps, and structs, all in one concise expression. The syntax typically involves specifying the type and using curly braces to define the initial values. This feature is particularly useful when you want to create a data structure without the need for multiple lines of code.
For example, when initializing a struct, you can directly assign values to its fields using a composite literal. This not only makes your code cleaner but also easier to read. Let’s dive into some specific examples to illustrate how to use composite literals effectively in Go.
Using Composite Literals with Arrays
Arrays are fixed-size collections of elements of the same type. Using composite literals to initialize arrays is straightforward. Here’s how you can do it:
package main
import "fmt"
func main() {
arr := [5]int{1, 2, 3, 4, 5}
fmt.Println(arr)
}
Output:
[1 2 3 4 5]
In the code above, we define an array of integers with a size of 5 and initialize it with values from 1 to 5. The composite literal [5]int{1, 2, 3, 4, 5}
specifies the type of the array, followed by the values in curly braces. This concise syntax allows for quick initialization, making your code cleaner and more efficient.
Using Composite Literals with Slices
Slices are more flexible than arrays, as they can grow and shrink dynamically. You can also use composite literals to initialize slices. Here’s an example:
package main
import "fmt"
func main() {
slice := []string{"apple", "banana", "cherry"}
fmt.Println(slice)
}
Output:
[apple banana cherry]
In this example, we create a slice of strings and initialize it with three fruit names. The syntax []string{"apple", "banana", "cherry"}
indicates that we are creating a slice of strings. This method is particularly useful for initializing collections where the number of elements may vary, as slices can easily accommodate changes in size.
Using Composite Literals with Maps
Maps are key-value pairs that allow for efficient data retrieval. You can initialize maps using composite literals as well. Here’s how:
package main
import "fmt"
func main() {
m := map[string]int{"apple": 5, "banana": 3, "cherry": 2}
fmt.Println(m)
}
Output:
map[apple:5 banana:3 cherry:2]
In this code snippet, we create a map where the keys are strings (fruit names) and the values are integers (quantities). The syntax map[string]int{"apple": 5, "banana": 3, "cherry": 2}
clearly defines the structure of the map. This allows you to initialize the map with values in a single line, making it easy to manage and understand.
Using Composite Literals with Structs
Structs are user-defined types that group related fields. Composite literals are particularly useful for initializing structs. Here’s an example:
package main
import "fmt"
type Person struct {
Name string
Age int
}
func main() {
p := Person{Name: "John", Age: 30}
fmt.Println(p)
}
Output:
{Name:John Age:30}
In this example, we define a struct called Person
with two fields: Name
and Age
. We then initialize it using a composite literal Person{Name: "John", Age: 30}
. This approach makes it clear which values correspond to which fields, enhancing readability and maintainability.
Conclusion
Composite literals in Go are an essential feature that allows developers to create and initialize complex data structures efficiently. By understanding how to use composite literals with arrays, slices, maps, and structs, you can write cleaner, more maintainable code. This guide has provided you with practical examples and explanations to help you get started with composite literals in your Go applications. Embrace this powerful feature, and watch your coding efficiency soar!
FAQ
-
What are composite literals in Go?
Composite literals are a way to create and initialize complex data structures like arrays, slices, maps, and structs in Go. -
How do I initialize an array using composite literals?
You can initialize an array by specifying its type followed by values in curly braces, e.g.,[5]int{1, 2, 3, 4, 5}
. -
Can I use composite literals for maps?
Yes, you can use composite literals to initialize maps by specifying the key-value pairs in curly braces, e.g.,map[string]int{"apple": 5}
.
-
Are composite literals only for built-in types?
No, composite literals can also be used for user-defined types such as structs. -
How do composite literals improve code readability?
Composite literals allow for concise initialization of data structures, making the code cleaner and easier to understand at a glance.