How to Remove Uncommitted Changes in Git
-
Use
git checkout
to Remove Uncommitted Changes in Git -
Use
git reset
to Remove Uncommitted Changes in Git -
Use
git stash
andgit stash
to Remove Uncommitted Changes in Git
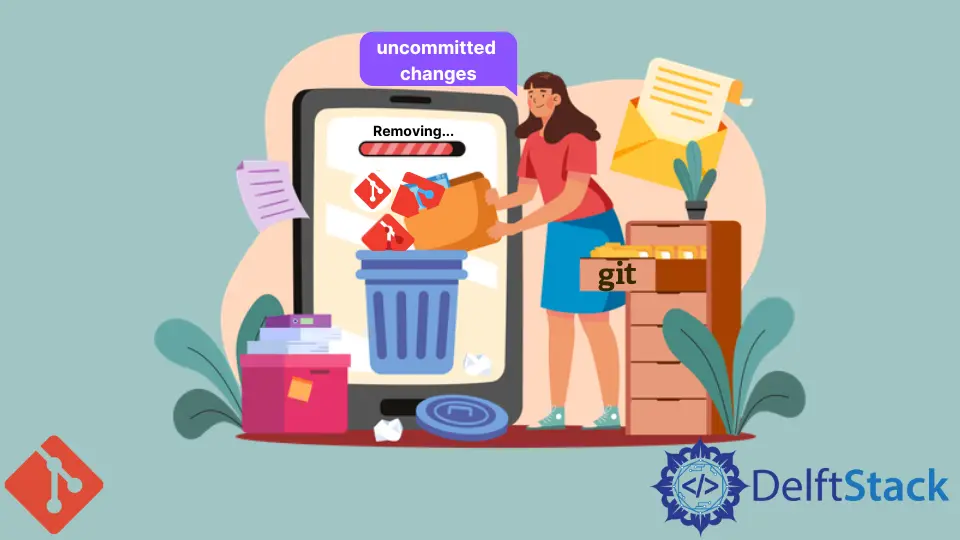
This article will guide you on how to undo uncommitted changes we have made to the local repository.
When working with a feature, we might first create new files, add changes to existing files, and then delete some files. Eventually, we realize it’s all wrong and need to go back to the previous commit. What should we do?
$ echo 'Add new implementation' > feature.txt
$ echo 'Enhance exising feature' >> file.txt
$ git add file.txt
$ rm deprecated_feature.txt
$ git status
On branch main
Changes to be committed:
(use "git restore --staged <file>..." to unstage)
modified: file.txt
Changes not staged for commit:
(use "git add/rm <file>..." to update what will be committed)
(use "git restore <file>..." to discard changes in working directory)
deleted: deprecated_feature.txt
Untracked files:
(use "git add <file>..." to include in what will be committed)
feature.txt
We have several ways to accomplish it.
Use git checkout
to Remove Uncommitted Changes in Git
This command will revert uncommitted changes for tracked files. Tracked files are files that git knows about, generally after being added by git add
$ git checkout .
Updated 2 paths from the index
$ git status
On branch main
Untracked files:
(use "git add <file>..." to include in what will be committed)
feature.txt
nothing added to commit but untracked files present (use "git add" to track)
Please note that git checkout
won’t work out if files have already been added to the staging area via git add
.
$ echo 'Enhance exising feature' >> file.txt
$ git add file.txt
$ git checkout file.txt
Updated 0 paths from the index
$ git status
On branch main
Changes to be committed:
(use "git restore --staged <file>..." to unstage)
modified: file.txt
Untracked files:
(use "git add <file>..." to include in what will be committed)
feature.txt
In the example above, changes to file.txt
are not reverted because this file is in the staging area.
Use git reset
to Remove Uncommitted Changes in Git
To remove uncommitted changes in the staging area, we need to take the following steps.
- Unstage file from staging area with
git reset
. - Undo changes using
git checkout
.
$ git reset file.txt
Unstaged changes after reset:
M file.txt
$ git checkout file.txt
Updated 1 path from the index
$ git status
On branch main
Untracked files:
(use "git add <file>..." to include in what will be committed)
feature.txt
nothing added to commit but untracked files present (use "git add" to track)
Another way to remove uncommitted changes using git reset
is with option --hard
and params HEAD
.
$ git reset --hard HEAD
HEAD is now at 1e087f5 Make some change to file.txt
$ git status
On branch main
Untracked files:
(use "git add <file>..." to include in what will be committed)
feature.txt
nothing added to commit but untracked files present (use "git add" to track)
- The
--hard
option specifies Git to throw ALL changes between the current state and the commit in the last argument. For that reason, this command is considered dangerous and should be used after you rungit status
to check working files. HEAD
alias for the latest commit.
Use git stash
and git stash
to Remove Uncommitted Changes in Git
The drawback of git checkout
and git reset
is that they couldn’t remove untracked files. feature.txt
persists after executing those commands.
Consider the first example.
$ echo 'Add new implementation' > feature.txt
$ echo 'Enhance exising feature' >> file.txt
$ git add file.txt
$ rm deprecated_feature.txt
$ git status
On branch main
Changes to be committed:
(use "git restore --staged <file>..." to unstage)
modified: file.txt
Changes not staged for commit:
(use "git add/rm <file>..." to update what will be committed)
(use "git restore <file>..." to discard changes in working directory)
deleted: deprecated_feature.txt
Untracked files:
(use "git add <file>..." to include in what will be committed)
feature.txt
To remove all uncommitted changes, including the staged files, tracked but unstaged, and untracked files. We’re going to use neatly approach git stash
.
git stash
allows us to save the changes but does not require a git commit
; it acts as temporary storage for uncommitted files.
After adding changes to temporary storage, we’ll tell Git to drop
those are stored. Hence, all uncommitted changes will be gone.
$ git add .
$ git stash
Saved working directory and index state WIP on main: 16b9767 deprecated_feature.txt
$ git stash drop
Dropped refs/stash@{0} (aebeb2cbdcec917331f5793ef1238f5a525d29ec)
$ git status
On branch main
nothing to commit, working tree clean
In summary, we have several approaches to remove uncommitted changes:
git checkout
is only useful when files are not in the staging area.git reset
useful for changes that are in staging area but cannot remove changes on untracked files, requires a combination withgit checkout
.git reset --hard HEAD
may be shorter than the above but is potentially dangerous.git stash
withgit add .
can remove everything, including untracked files.