How to Force Clean Build of an Image in Docker
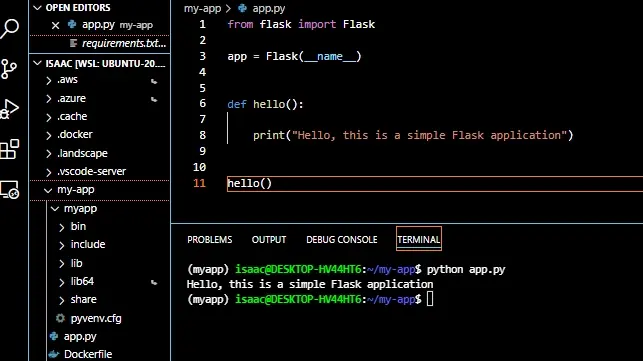
The tutorial will discuss and demonstrate how to force a clean build of an image in Docker.
Build an Image in Docker
We will be using a simple Flask application to demonstrate this concept. Using the command below, create a simple application named app.py
within the main folder my-app
.
touch app.py
Install Python3 if you have not used the command.
sudo apt update && sudo apt install python3-venv
We can also install Flask and Gunicorn using the command.
pip install flask gunicorn
Proceed to create a docker file using the command touch Dockerfile
. To make creating a docker file easier for us, we create a requirements.txt
file.
We can create a requirements.txt
file by running the pip freeze > requirements.txt
command. Once done, your file structure should be similar to the one below.
We can proceed to the app.py
file and create our application. The application contains a simple function that prints a console message, as shown below.
from flask import Flask
app = Flask(__name__)
def hello():
print("Hello, this is a simple Flask application")
hello()
Here is the result to expect when we execute the code above.
Now we will populate our Dockerfile with commands that Docker will use to build an image. These commands will be executed when we run the docker container.
We will be using Python as our base image in this case. The Dockerfile should look like this.
# base image
FROM python
# Set your working directory
WORKDIR /var/www/
# Copy the necessary files
COPY ./app.py /var/www/app.py
COPY ./requirements.txt /var/www/requirements.txt
# Install the necessary packages
RUN pip install -r /var/www/requirements.txt
# Run the app
CMD python3 app.py
Once we have our Dockerfile ready, we can save it and build our image locally for testing. We can use the docker build
command alongside the -t
tag.
Then, we can test the image using the docker run
command, as shown below.
(myapp) isaac@DESKTOP-HV44HT6:~/my-app$ docker run -it myapp
Hello, this is a simple Flask application
We’ll confirm that we have successfully built a new container using the docker ps
command.
$ docker ps -a
CONTAINER ID IMAGE COMMAND CREATED STATUS PORTS NAMES
dd10c9a6a8a1 myapp "/bin/sh -c 'python3…" 11 minutes ago Exited (0) 10 minutes ago vigilant_brattain
Use the --no-cache
Option to Force Clean Build of an Image in Docker
Having built a container using this image, suppose we want to build an image again using the same image. In that case, the docker daemon will use the existing cache of the image layers to build the image.
However, we can force a clean image build in Docker using the --no-cache
option. This overrides the docker daemon default behavior.
Here is how we can implement that.
myapp) isaac@DESKTOP-HV44HT6:~/my-app$ docker build --no-cache -t myapp .
[+] Building 119.0s (10/10) FINISHED
=> [internal] load .dockerignore 1.6s
=> => transferring context: 2B 0.4s
=> [internal] load build definition from Dockerfile 2.1s
=> => transferring dockerfile: 38B 0.4s
=> [internal] load metadata for docker.io/library/python:latest 37.9s
=> [1/5] FROM docker.io/library/python@sha256:ee0b617025e112b6ad7a4c76758e4a02f1c429e1b6c44410d95b024304698ff2 0.1s
=> [internal] load build context 0.4s
=> => transferring context: 63B 0.0s
=> CACHED [2/5] WORKDIR /var/www/ 0.1s
=> [3/5] COPY ./app.py /var/www/app.py 1.3s
=> [4/5] COPY ./requirements.txt /var/www/requirements.txt 2.2s
=> [5/5] RUN pip install -r /var/www/requirements.txt 68.4s
=> exporting to image 4.8s
=> => exporting layers 3.8s
=> => writing image sha256:ee771b73a9ec468308375d139a35580f6c7f62988db9c0bb0b85794716406e92 0.1s
=> => naming to docker.io/library/myapp
We can create a new container using the docker run
command.
(myapp) isaac@DESKTOP-HV44HT6:~/my-app$ docker run -it myapp
Hello, this is a simple Flask application
As seen below, we have successfully built a new container and forced Docker for a clean build of an image using the --no-cache
option.
$ docker ps -a
CONTAINER ID IMAGE COMMAND CREATED STATUS PORTS NAMES
826b8de2c92f myapp "/bin/sh -c 'python3…" 47 seconds ago Exited (0) 39 seconds ago kind_pike
dd10c9a6a8a1 ba84c5d3b157 "/bin/sh -c 'python3…" 28 minutes ago Exited (0) 28 minutes ago vigilant_brattain
Isaac Tony is a professional software developer and technical writer fascinated by Tech and productivity. He helps large technical organizations communicate their message clearly through writing.
LinkedIn