How to Rebuild a Container in a Docker-Compose File
- Create a New Project
- Create a Dockerfile for the Image
- Create a Compose File for the Containers
-
Run Containers Using
compose
-
Rebuild a Single Container Using
compose
- Conclusion
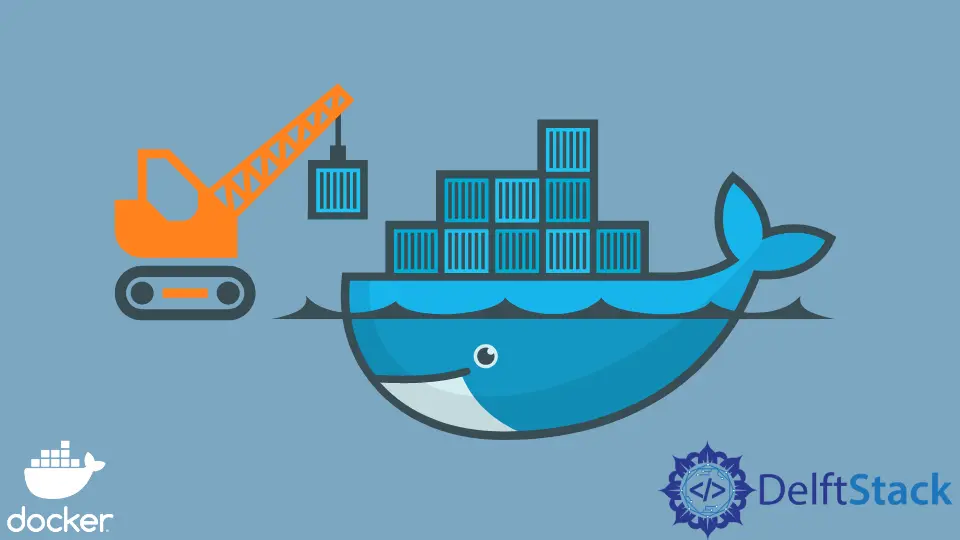
Before we implement our application, we need to understand the difference between a Dockerfile
and a compose.yaml
file.
When creating custom images for our applications, we usually use a file named Dockerfile
and run containers from it on the command line using the docker run
command. If we want to create multiple images, these command becomes too tedious and hard to manage the containers, so this is where the compose.yaml
file comes in.
The compose.yaml
file builds the images, runs the containers, creates a network for these containers, and adds them to the network. We can then access the network applications using the service’s name.
These two commands must exist in the application so that the docker compose up
command can create and run the images.
If any changes were made to the image, these command gets the changes, stops the containers, and recreates the images. In this tutorial, we will see how to rebuild a single docker container from multiple containers defined in a Docker file.
Create a New Project
Open IntelliJ IDEA and select File > New > Project
. On the opened window, select Node.js and change the project name from untitled
to docker-rebuild-container
or use any name preferred.
Ensure the Node runtime environment is installed so that the Node interpreter and Package manager sections are added automatically from the computer. Lastly, click the Create button to generate the project.
Create a file named index.js
under the docker-rebuild-container
folder and copy and paste the following code into the file.
const express = require('express')
const app = express()
const port = 3000
app.get('/', (req, res) => {
res.json(
[
{
name: "Java in action",
author: "chad darby",
price: 345
},
{
name: 'React cookbook',
author: "mary public",
price: 600
},
])
})
app.listen(port, () => {
console.log(`Example app listening on port ${port}`)
})
In this file, we have created a sample Node.js application to create an image and run containers from it. This application exposes an API that can be accessed by issuing a get request to /
on the browser.
Create a Dockerfile for the Image
Create a file named Dockerfile
and copy and paste the following instructions into the file.
FROM node:16.17.0-alpine
WORKDIR /com/app
ADD package*.json ./
RUN npm install
ADD . .
CMD node index.js
This file defines the instructions to build an image and run a container from it. The docker documentation provides a detailed explanation of how these instructions work.
Create a Compose File for the Containers
Create a compose.yaml
file in the docker-rebuild-container
folder and copy and paste the following instructions into the file.
services:
service-one:
restart: on-failure
build: .
hostname: service-one
ports:
- '3000:3000'
service-two:
restart: on-failure
build: .
hostname: service-two
ports:
- '5000:3000'
This file defines two services named service-one
and service-two
with the services listening to port 3000 in the container from port 3000 and 5000 in the host, respectively.
services
- This defines the computing components of an application.restart
- This defines the action to take when a container is terminated.build
- This defines the source of theDockerfile
containing the configuration to build an image.hostname
- This sets a custom name to be used by the service.ports
- This exposes the ports on the host machine to serve the application.
The Docker documentation provides a docker compose file reference explaining these instructions in detail.
Run Containers Using compose
In this example, we will see how to build and run all the containers defined at once. To execute our docker compose.yaml
file, open a new terminal window on your development environment using the keyboard shortcut ALT + F12 and the following command to build and run the containers.
~/WebstormProjects/docker-rebuild-container$ docker compose up --build --force-recreate --no-deps -d
With this command, it becomes very easy to run and manage containers. Before using this command, we run docker compose up -d
to ensure we are rebuilding the image and recreating the containers with this command.
=> CACHED [docker-rebuild-container_service-two 2/5] WORKDIR /com/app 0.0s
=> CACHED [docker-rebuild-container_service-two 3/5] ADD package*.json ./ 0.0s
=> CACHED [docker-rebuild-container_service-two 4/5] RUN npm install 0.0s
=> [docker-rebuild-container_service-two 5/5] ADD . . 1.2s
=> [docker-rebuild-container_service-one] exporting to image
--build
- This ensures the images are built before running the containers.--force-recreate
- This recreates the containers without considering the state of their configurations and images.--no-deps
- This ensures that the linked services are not started.
Rebuild a Single Container Using compose
To rebuild a single container from multiple containers defined in the compose.yaml
file, use the command used in the previous example and add the name of the service to be rebuilt, as shown below.
~/WebstormProjects/docker-rebuild-container$ docker compose up --build --force-recreate --no-deps -d service-one
This command rebuilds the image and recreates the container named service-one
since we only want to recreate a single container. We can verify from this command’s output that only one container is recreated, as shown below.
[+] Running 1/1
⠿ Container docker-rebuild-container-service-one-1 Started
To verify whether the containers are working as expected, issue two requests on the browser, one on localhost:3000
(http://localhost:3000/) and another on localhost:5000
(http://localhost:5000/), and note that we can access the same application from the two containers. The following is the JSON response returned by the containers.
[
{
"name": "Java in action",
"author": "chad darby",
"price": 345
},
{
"name": "React cookbook",
"author": "mary public",
"price": 600
}
]
Conclusion
In this tutorial, we’ve learned how to define an image using a Dockerfile
, define containers using the compose.yaml
file, run containers in a compose file, and recreate a single container in a compose file.
Note that the syntax for running containers from a compose file is docker compose up [OPTIONS] [SERVICE...]
, and the optional SERVICE
parameter allows us to specify one or more services we want to rebuild their images or recreate their containers.
David is a back end developer with a major in computer science. He loves to solve problems using technology, learning new things, and making new friends. David is currently a technical writer who enjoys making hard concepts easier for other developers to understand and his work has been published on multiple sites.
LinkedIn GitHubRelated Article - Docker Container
- How to Check if the Docker Container Is Running or Not
- How to Restart a Docker Container
- The Difference Between Docker Container and Docker Image
- How to Delete Containers Permanently in Docker
- How to List Only the Stopped Containers in Docker
- How to Enter a Running Docker Container With a New Pseudo TTY