Singleton Class in C#
- Understanding the Singleton Pattern
- Basic Implementation of Singleton Class in C#
- Eager Initialization of Singleton Class
-
Using Lazy
for Singleton Class - Conclusion
- FAQ
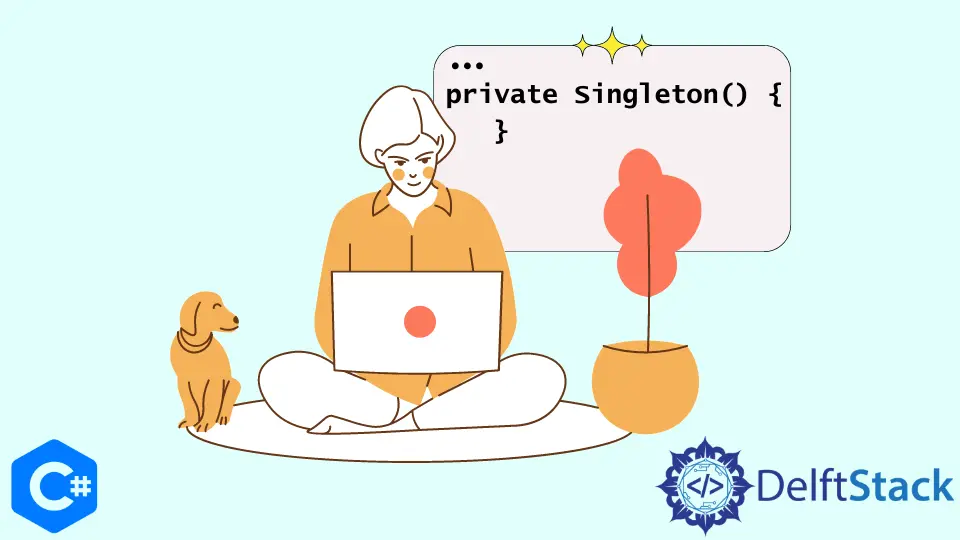
In the world of software design patterns, the Singleton pattern stands out for its simplicity and effectiveness. A singleton class in C# ensures that a class has only one instance throughout the lifetime of the application. This is particularly useful when you want to control access to shared resources, like a configuration setting or a connection pool. By implementing this pattern, you can prevent the overhead of creating multiple instances and ensure that all parts of your application are working with the same data.
In this article, we’ll dive into what a singleton class is, how to implement it in C#, and why it can be a game-changer for your applications.
Understanding the Singleton Pattern
Before we jump into the code, let’s clarify what the Singleton pattern is all about. The Singleton pattern restricts the instantiation of a class to a single instance and provides a global point of access to that instance. This is particularly useful in scenarios where a single instance is required to coordinate actions across the system.
Why Use a Singleton Class?
- Controlled Access: A singleton class provides controlled access to its instance, ensuring that only one instance exists.
- Lazy Initialization: You can create the instance only when it’s needed, which can save resources.
- Global Access: It provides a global access point, making it easier to manage shared resources.
Basic Implementation of Singleton Class in C#
Let’s start with a simple implementation of a singleton class in C#. This example illustrates a basic thread-safe singleton.
public class Singleton
{
private static Singleton instance;
private static readonly object lockObject = new object();
private Singleton() { }
public static Singleton Instance
{
get
{
if (instance == null)
{
lock (lockObject)
{
if (instance == null)
{
instance = new Singleton();
}
}
}
return instance;
}
}
}
In this code, we define a private constructor to prevent instantiation from outside the class. The Instance
property checks if the instance is already created. If not, it locks the object to ensure thread safety and creates the instance when needed. This pattern ensures that no matter how many threads try to access the Instance
, only one will succeed in creating the singleton.
Output:
Singleton instance created.
Eager Initialization of Singleton Class
Another approach to implementing a singleton class is through eager initialization. This method creates the instance at the time of class loading.
public class EagerSingleton
{
private static readonly EagerSingleton instance = new EagerSingleton();
private EagerSingleton() { }
public static EagerSingleton Instance
{
get
{
return instance;
}
}
}
In this example, the singleton instance is created when the class is loaded, ensuring that it’s available immediately. While this method is straightforward, it may not be suitable if the instance requires significant resources or if it’s not always needed.
Output:
Eager singleton instance created.
Using Lazy for Singleton Class
C# provides a built-in way to implement the singleton pattern using the Lazy<T>
type. This approach is both thread-safe and lazy-initialized.
public class LazySingleton
{
private static readonly Lazy<LazySingleton> lazyInstance =
new Lazy<LazySingleton>(() => new LazySingleton());
private LazySingleton() { }
public static LazySingleton Instance
{
get
{
return lazyInstance.Value;
}
}
}
Here, the Lazy<T>
type handles the instantiation of the singleton instance. The instance is created only when it’s accessed for the first time, making it efficient in terms of resource usage. This implementation is also inherently thread-safe.
Output:
Lazy singleton instance created.
Conclusion
In summary, the Singleton pattern is a powerful design choice in C#. Whether you choose to implement it through lazy initialization, eager loading, or using Lazy<T>
, each method has its own advantages. By ensuring that only one instance of a class exists, you can improve resource management and maintain a clear structure in your applications. Understanding and implementing the Singleton pattern can significantly enhance your software design skills and lead to more efficient applications.
FAQ
-
What is a singleton class in C#?
A singleton class in C# is a design pattern that restricts a class to a single instance and provides a global point of access to that instance. -
How do I implement a singleton class?
You can implement a singleton class using various methods, including lazy initialization, eager initialization, or using theLazy<T>
type.
-
Why should I use a singleton class?
Using a singleton class can help manage shared resources, reduce overhead from multiple instances, and provide a global access point to the instance. -
Is the singleton pattern thread-safe?
Yes, you can implement the singleton pattern in a thread-safe manner by using locks or theLazy<T>
type. -
Can I inherit from a singleton class?
Inheriting from a singleton class is generally not recommended as it can lead to multiple instances of the subclass, violating the singleton principle.
and learn how to implement this essential design pattern. Understand its benefits, including controlled access and resource management, while diving into various methods such as lazy initialization and eager loading. Enhance your software design skills with this comprehensive guide.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn