How to Simulate Mouse Click Events in C#
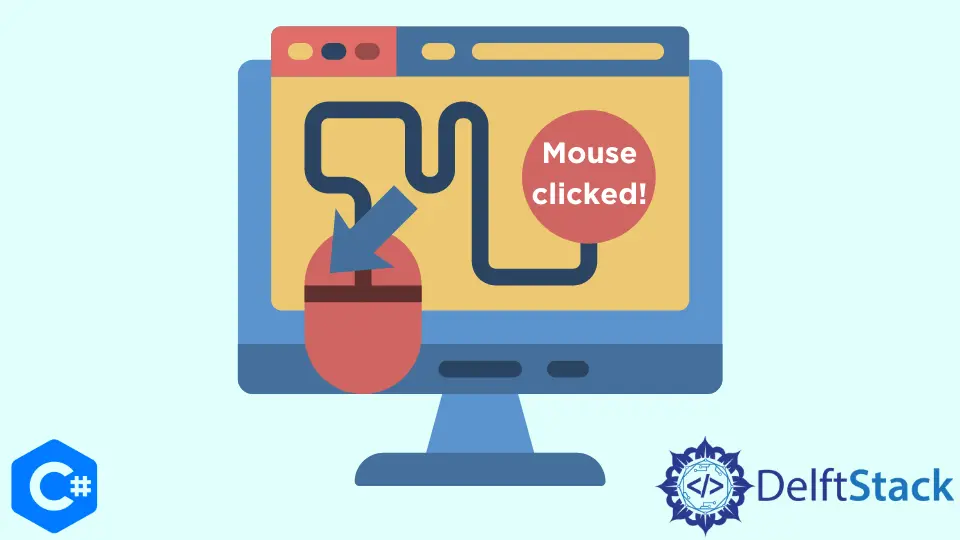
This brief article is about mouse-click event simulation in C#. We will discuss some of the methods available in C# libraries to help us simulate the mouse click event.
Simulating mouse events means writing a code that can control the mouse movements or trigger the mouse click event, which, in turn, can trigger the relevant mouse event handlers. We can use native Windows functionalities for manipulating the mouse (i.e., simulating the cursor movements and clicks).
Let’s start by discussing what the mouse events are and why these can’t be used to simulate the events.
Mouse Events in C#
Most events have a corresponding handler method that executes certain pieces of code when these events occur. These methods usually follow the format of On
followed by EventName
, such as OnMouseMove
.
Due to the protected nature of these methods, which prevent access from outside the control’s or form’s context, this option is only available within custom controls or forms.
These methods are just event handlers (e.g., OnMouseMove
) and execute when the corresponding event occurs. These methods don’t control the events.
For example, the OnMouseMove
method will do something with the cursor’s movement but cannot control the cursor itself.
The list of available events handlers that execute associated routines/actions when a specific mouse event happens are as follows:
OnMouseDown
OnMouseEnter
OnMouseHover
OnMouseLeave
OnMouseMove
OnMouseUp
OnMouseWheel
OnMouseClick
OnMouseDoubleClick
As discussed in this section, event handlers can’t be used for simulating events. However, some other OS native methods are available to simulate the events.
Let’s explore them in the subsequent section.
Simulate Mouse Events in C#
Windows Forms offers simple methods to initiate the click because most controls have some click-associated actions, such as a button
executing user code or a checkbox
changing its checked state. The Comboboxes
is one type of control that doesn’t respond when clicked, and simulating a click has no impact on the control.
the PerformClick()
Method
Microsoft’s System.Windows.Forms.IButtonControl
provides the PerformClick
method, available through the IButtonControl
interface, and simulates a click on some form control. All controls implement this interface, including System.Windows.Forms.Button
.
In the following example, we have created a Windows Form Application. The form contains two buttons. On clicking the first button, we can also perform the click on the second button using the PerformClick
method.
The interface of this coding example will look like this:
The corresponding handler functions for these buttons, named button1
and button2,
are as follows:
private void button1_Click(object sender, EventArgs e) {
button2.PerformClick();
}
private void button2_Click(object sender, EventArgs e) {
MessageBox.Show("Button 2 Clicked!!");
}
In the above code, you can see that we have called the method button2.PerformClick()
in the event handler of button1
. This will call the click event handler for button2
and eventually show the Message Box coded in the hander function of button2
.
Invoke Click for Checkbox and Handle Mouse Movements
Use the InvokeOnClick
function to simulate a mouse click with a form’s custom control. This method is protected
and can only be used by the form or derived custom control.
For instance, the code below selects a checkbox on clicking the button. The interface of the example contains two checkboxes and their corresponding two buttons.
One label
displaying the top heading is placed at the top, and a button
is at the bottom to simulate the mouse movement. This interface is shown below:
The event handler functions for the two buttons will be:
private void button1_Click(object sender, EventArgs e) {
InvokeOnClick(checkBox1, EventArgs.Empty);
}
private void button2_Click(object sender, EventArgs e) {
InvokeOnClick(checkBox2, EventArgs.Empty);
}
Native Windows Methods to Move the Cursor
Windows operating system provides a few native methods to control mouse movements and keyboard events. For this example, we will discuss and demonstrate only the functions to control mouse movements and change the cursor position.
In the example above, a non-functional button at the bottom says: Click me to Move Mouse Pointer to Heading
. This button is added to move the cursor position toward the top heading: Mouse Simulation Demo
.
Let’s make this button functional.
The user32.dll
provides the SetCursorPos
API, defined in the namespace System.Runtime.InteropServices
. This method invokes native Windows procedures and must be defined as an external method.
The following code will set the cursor position:
[DllImport("user32.dll", EntryPoint = "SetCursorPos")]
[return:MarshalAs(UnmanagedType.Bool)]
private static extern bool SetCursorPos(int x, int y);
private void button3_Click(object sender, EventArgs e) {
Point position = PointToScreen(label1.Location) + new Size(label1.Width / 2, label1.Height / 2);
SetCursorPos(position.X, position.Y);
}
We created a Point
object to get the label’s location at the top, added the points to get the middle point of the label, and then passed its coordinates to the SetCursorPos
function.
The complete code for this example will be:
using System;
using System.Drawing;
using System.Runtime.InteropServices;
using System.Windows.Forms;
namespace WinFormsApp2 {
public partial class Form1 : Form {
[DllImport("user32.dll", EntryPoint = "SetCursorPos")]
[return:MarshalAs(UnmanagedType.Bool)]
private static extern bool SetCursorPos(int x, int y);
public Form1() {
InitializeComponent();
}
private void button1_Click(object sender, EventArgs e) {
InvokeOnClick(checkBox1, EventArgs.Empty);
}
private void button2_Click(object sender, EventArgs e) {
InvokeOnClick(checkBox2, EventArgs.Empty);
}
private void button3_Click(object sender, EventArgs e) {
Point position =
PointToScreen(label1.Location) + new Size(label1.Width / 2, label1.Height / 2);
SetCursorPos(position.X, position.Y);
}
}
}
The output of this mouse simulation is depicted in the image below: