How to Send Email With Attachment in C#
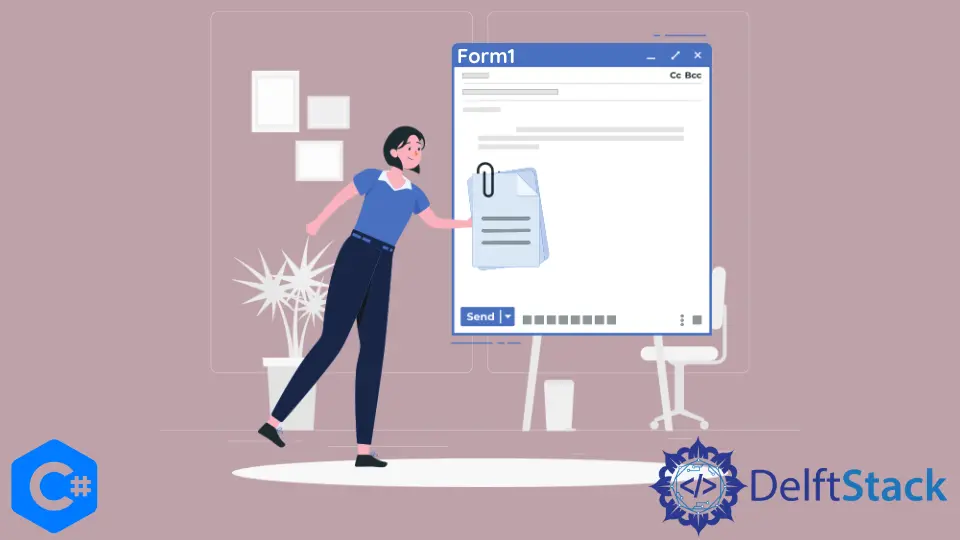
This tutorial will demonstrate how to use the SMTP client in C# to send emails with attachments.
NET and .NET Core have built-in capability for sending emails via the System.Net.Mail namespace. While it may be the easiest option, this requires an SMTP server.
Use your existing Gmail account or create a new one to send emails over SMTP. You must enable less secure app access on your profile’s security tab to utilize Google’s SMTP servers.
Create a Form to Be Used to Send Email With Attachment in C#
First, create a new Windows Form Application in Visual Studio.
Open your .cs
design file and create a panel inside your form. Add a label with the text Compose
, label1
, and at the right-most, X
to close this form.
To add functionality, double-click on the label, and it will navigate you to the label_Click
function. Write the following line inside.
this.Dispose();
Create 5 text boxes named as follows:
txtFrom
txtPassword
txtTo
txtTitle
txtBody
In the txtPassword
text box, add a character (*
or •
) in the passwordChar
property to hide the password entered by the user in the bottom-right Solution Explorer panel.
Create a button named btnSend
with the Send
inside it, and assign it a blue color. This button will send the email to its destination email address.
Now, you need one more button to attach a file with your email before sending it and a label to show the selected file path. So, let’s create a LinkLabel and name it linkLabel1
and a label called lblLocation
.
Assign the LinkLabel an image and a text Attach File
from the Solution Explorer. Now, double-click on this LinkLabel in your .cs
form design file, and it will navigate you to this button clicked function code.
Inside this function, we need to open a dialog. But before that, you have to drag and drop OpenFileDialog into your form design and connect it to the Attach File
button.
We’ll write the following code inside the linkLabel1_linkClicked
function to open the Dialog and connect its selected file path to display in lblLocation
.
openFileDialog1.ShowDialog();
lblLocation.Text = openFileDialog1.FileName;
Output:
Now that everything is ready, gather the sender’s email and password, recipient email, the message, and the attached file. Let’s write the functional code to send an email using the SMTP client.
Double-click the Send
button we created in our form design. Inside its button1_Click
function, we’ll perform the following operations.
Send Email With Attachment in C#
To start, create a try-catch
statements block. The program execution tries the try
block, and if any error occurs, it automatically catches the exception.
try {
} catch () {
}
Inside the try
block, we declare a variable of data type MailMessage named mail
and initialize its default constructor MailMessage()
to use its properties in the email sending process.
MailMessage mail = new MailMessage();
Now, declare another variable, smtp
, of data type SmtpClient, and initialize a new instance of the SmtpClient that requires a string host. We pass smtp.gmail.com
because we need to exchange mails between Gmail.
SmtpClient smtp = new SmtpClient("smtp.gmail.com");
Then, we’ll use .From
, .To.Add()
, .Subject
, and .Body
of the MailMessage class and assign them the values of our text boxes.
mail.From = new MailAddress(txtFrom.Text);
mail.To.Add(txtTo.Text);
mail.Subject = txtTitle.Text;
mail.Body = txtBody.Text;
Then, we need to attach the selected file with the mail. We will create a variable attachment
of the System.Net.Mail.Attachment
data type and assign it the path of the selected file.
Attach the file with the mail using the .Attachments.Add()
method by passing it the attachment.
System.Net.Mail.Attachment attachment;
attachment = new System.Net.Mail.Attachment(lblLocation.Text);
mail.Attachments.Add(attachment);
We need to assign some values to SMTP, including the port that Gmail uses, the sender’s email address, and the password. We should enable the SSL (Secure Sockets Layer) to transmit the data securely.
Then, we call the Send()
method and pass our mail. After sending the mail, let’s create a message box that will show a message with an information icon and an OK button.
smtp.Port = 587;
smtp.Credentials = new System.Net.NetworkCredential(txtFrom.Text, txtPassword.Text);
smtp.EnableSsl = true;
smtp.Send(mail);
MessageBox.Show("Mail has been sent successfully!", "Email Sent", MessageBoxButtons.OK,
MessageBoxIcon.Information);
Now, the catch
block will contain the following code. These lines of code will catch any exception that occurs and will output it in a MessageBox.
catch (Exception ex) {
MessageBox.Show(ex.Message);
}
Complete Source Code
using System;
using System.Net.Mail;
namespace send_email_with_attachment {
public partial class Form1 : Form {
public Form1() {
InitializeComponent();
}
private void label1_Click(object sender, EventArgs e) {
this.Dispose();
}
private void label5_Click(object sender, EventArgs e) {}
private void label7_Click(object sender, EventArgs e) {}
private void button1_Click(object sender, EventArgs e) {
try {
MailMessage mail = new MailMessage();
SmtpClient smtp = new SmtpClient("smtp.gmail.com");
mail.From = new MailAddress(txtFrom.Text);
mail.To.Add(txtTo.Text);
mail.Subject = txtTitle.Text;
mail.Body = txtBody.Text;
System.Net.Mail.Attachment attachment;
attachment = new System.Net.Mail.Attachment(lblLocation.Text);
mail.Attachments.Add(attachment);
smtp.Port = 587;
smtp.Credentials = new System.Net.NetworkCredential(txtFrom.Text, txtPassword.Text);
smtp.EnableSsl = true;
smtp.Send(mail);
MessageBox.Show("Mail has been sent successfully!", "Email Sent", MessageBoxButtons.OK,
MessageBoxIcon.Information);
} catch (Exception ex) {
MessageBox.Show(ex.Message);
}
}
private void linkLabel1_LinkClicked(object sender, LinkLabelLinkClickedEventArgs e) {
openFileDialog1.ShowDialog();
lblLocation.Text = openFileDialog1.FileName;
}
private void txtFrom_TextChanged(object sender, EventArgs e) {}
}
}
Output:
Press send, and the following Message Box will appear.
I'm a Flutter application developer with 1 year of professional experience in the field. I've created applications for both, android and iOS using AWS and Firebase, as the backend. I've written articles relating to the theoretical and problem-solving aspects of C, C++, and C#. I'm currently enrolled in an undergraduate program for Information Technology.
LinkedIn