How to IIF Equivalent in C#
- Understanding the Ternary Operator in C#
- Using the Ternary Operator for Conditional Assignment
- Nesting the Ternary Operator
- Conclusion
- FAQ
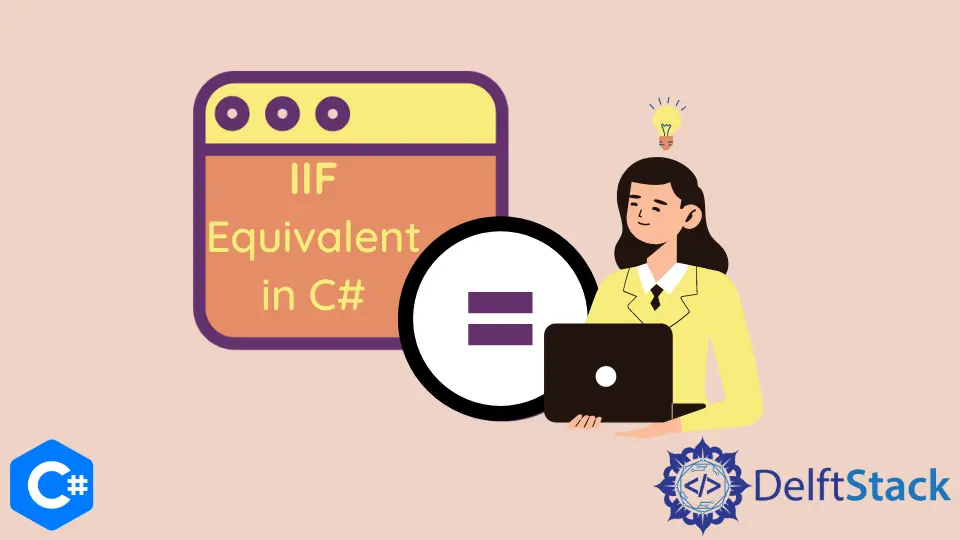
In the world of programming, efficiency and readability are paramount. If you’ve ever used Visual Basic, you’re likely familiar with the IIF
function. However, if you’re transitioning to C#, you might be wondering how to achieve the same functionality. Fear not!
This tutorial will guide you through using the ?
ternary operator in C# as the equivalent of IIF
. By the end, you’ll have a solid understanding of how to write concise conditional expressions that enhance your code’s clarity. Let’s dive into the world of C# and unlock the power of the ternary operator!
Understanding the Ternary Operator in C#
The ternary operator in C# is a compact way to express conditional logic. It takes three operands: a condition, a result for true, and a result for false. The syntax looks like this:
condition ? trueResult : falseResult;
This operator is particularly useful for simple conditional expressions where you want to assign a value based on a condition. It can replace more verbose if-else
statements, making your code cleaner and easier to read.
For example, if you want to assign a string based on a boolean condition, you can do it succinctly with the ternary operator. Here’s a simple illustration:
bool isSunny = true;
string weather = isSunny ? "It's a sunny day!" : "It's not sunny.";
Output:
It's a sunny day!
In this example, if isSunny
is true, the string “It’s a sunny day!” is assigned to weather
. If it’s false, “It’s not sunny.” is assigned instead. This approach streamlines your code and reduces the need for multiple lines dedicated to condition checks.
Using the Ternary Operator for Conditional Assignment
Let’s explore how to use the ternary operator for more complex conditional assignments. Imagine you are building a program that categorizes a person’s age. You want to determine if they are an adult or a minor. Here’s how you could implement this using the ternary operator:
int age = 20;
string category = age >= 18 ? "Adult" : "Minor";
Output:
Adult
In this snippet, the condition checks if age
is greater than or equal to 18. If true, “Adult” is assigned to category
. Otherwise, “Minor” is assigned. This not only makes your code concise but also enhances readability, allowing anyone reviewing your code to quickly understand the logic being applied.
It’s important to note that while the ternary operator is powerful, it’s best used for simple conditions. Overusing it in complex situations can lead to code that is hard to read and maintain. Always aim for clarity in your code.
Nesting the Ternary Operator
Sometimes, you may need to evaluate multiple conditions. In such cases, you can nest the ternary operator, although this should be done with caution to maintain readability. Here’s an example where we categorize a person’s age into three groups: minor, adult, and senior:
int age = 70;
string category = age < 18 ? "Minor" : (age < 65 ? "Adult" : "Senior");
Output:
Senior
In this case, if age
is less than 18, “Minor” is assigned. If age
is between 18 and 64, “Adult” is assigned. Otherwise, “Senior” is assigned. While nesting is a powerful feature, it can quickly become difficult to decipher. It’s essential to strike a balance between brevity and clarity.
Conclusion
The ternary operator in C# serves as a robust alternative to the IIF
function found in Visual Basic. By mastering this operator, you can write more concise and readable code. Whether you’re assigning simple values or evaluating multiple conditions, the ternary operator is an invaluable tool in your programming arsenal. Remember to keep your code clear and avoid excessive nesting to ensure maintainability. With these tips, you’re now equipped to use the ternary operator effectively in your C# projects!
FAQ
-
What is the ternary operator in C#?
The ternary operator is a shorthand way to write conditional statements in C#, using the syntax condition ? trueResult : falseResult. -
Can I use the ternary operator for multiple conditions?
Yes, you can nest ternary operators to evaluate multiple conditions, but be cautious as it can lead to complex and hard-to-read code. -
Is the ternary operator faster than if-else statements?
The performance difference is negligible; the choice should be based on code readability and maintainability. -
Can I use the ternary operator with non-primitive data types?
Yes, the ternary operator can be used with any data type, including strings, objects, and collections. -
Are there any limitations to using the ternary operator?
The main limitation is readability; overusing or nesting too many conditions can make your code difficult to understand.