How to Get Property Value Using Reflection in C#
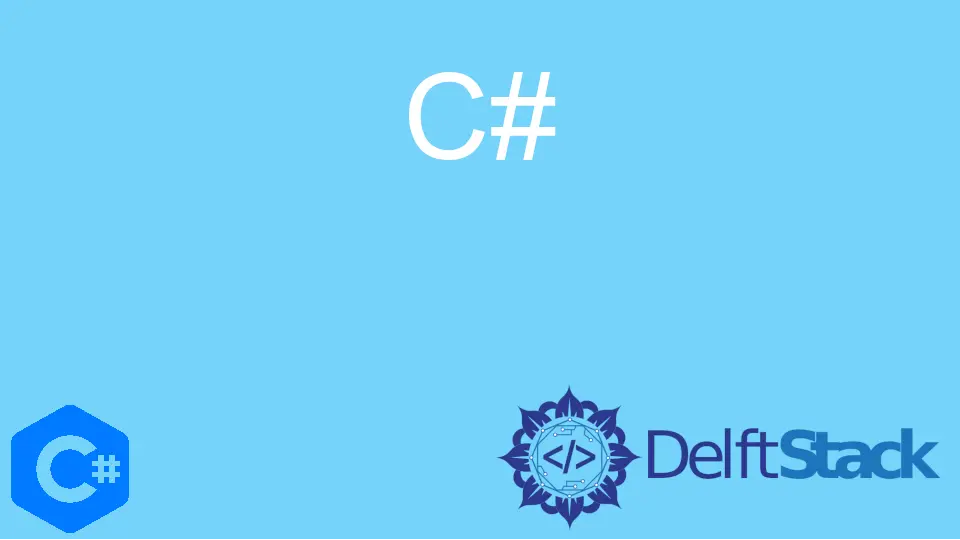
This article elaborates using the System.Reflection
namespace in C#. Using the Reflection
namespace, we will look at a sample project to get the property values.
the System.Reflection
in C#
The System.Reflection
namespace in C# is used to get information about the code’s parameters, assemblies, methods, modules, and other elements. You can get any class type, parameter values, methods, etc., by importing this namespace into your program.
We will demonstrate the technique to get the property value of an object using the System.Reflection
functionalities.
Get the Property’s Value Using Reflection in C#
Create a C# project in Visual Studio. Firstly, import the Reflection
namespace into your program.
using System.Reflection;
The System
namespace contains a class Type
that returns the object type.
Type type = _obj.GetType();
The Reflection
namespace contains a class named PropertyInfo
to get the properties of a type. The Type
class has a method GetProperty()
and GetProperties()
to return a property and an array of a class’s properties, respectively.
PropertyInfo[] properties = type.GetProperties();
The PropertyInfo
class contains a member attribute and method named Name
and GetValue()
to return the name of the property and the value assigned to it, respectively.
Console.WriteLine(p.Name + " : " + p.GetValue(_obj));
Following is the complete code snippet of the example project:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Reflection;
namespace GettingPropertyValue {
public class Book {
private String bookTitle;
private int numberOfPages;
public Book(String title, int pages) {
bookTitle = title;
numberOfPages = pages;
}
public String Title {
get { return bookTitle; }
}
public int Pages {
get { return numberOfPages; }
set { numberOfPages = value; }
}
}
public class MyGetValue {
public static void Main() {
Console.WriteLine("Getting all properties...");
Book book = new Book("Get Property Value in C#", 198);
GetPropertyValues(book);
Console.WriteLine("\n\nGetting value of a single property...");
GetPropertyValue(book, "Pages");
Console.ReadKey();
}
private static void GetPropertyValues(Object _obj) {
// returns the type of _obj
Type type = _obj.GetType();
Console.WriteLine("Type of Object is: " + type.Name);
// create an array of properties
PropertyInfo[] properties = type.GetProperties();
Console.WriteLine("Properties (Count = " + properties.Length + "):");
// print each property name and value
foreach (var p in properties)
if (p.GetIndexParameters().Length == 0)
Console.WriteLine(p.Name + "(" + p.PropertyType.Name + ") : " + p.GetValue(_obj));
else
Console.WriteLine(" {0} ({1}): <Indexed>", p.Name, p.PropertyType.Name);
}
// method to get a single property value from a string
private static void GetPropertyValue(Object _obj, string property) {
// prints property name
Console.WriteLine("Property : " + _obj.GetType().GetProperty(property).Name);
// prints property value
Console.WriteLine("Value : " + _obj.GetType().GetProperty(property).GetValue(_obj));
}
}
}
We have created a class named Book
with two properties (title and number of pages). We created two methods (i.e., GetPropertyValue
and GetPropertyValues
) in another class, MyGetValue
.
The GetProjectValue(Object _obj, string property)
takes an object and a string or property as parameters and prints the property name and value as output.
On the other hand, the GetPropertyValues(Object _obj)
method takes an object as a parameter. It first determines the type of object and gets all the properties along with the values of that object.
The output of the code snippet is as follows:
Getting all properties...
Type of Object is: Book
Properties (Count = 2):
Title(String) : Get Property Value in C#
Pages(Int32) : 198
Getting value of a single property...
Property : Pages
Value : 198
Geeks can visit System.Reflection
C# for an in-depth reference to the Reflections in C#.