C# でリフレクションを使用してプロパティ値を取得する
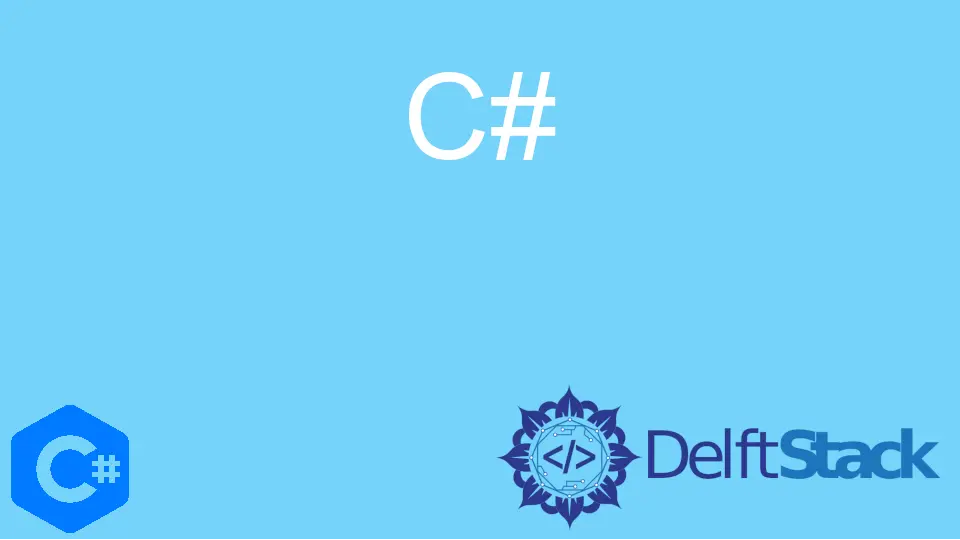
この記事では、C# での System.Reflection
名前空間の使用について詳しく説明します。 Reflection
名前空間を使用して、サンプル プロジェクトを見てプロパティ値を取得します。
C#
の System.Reflection
C# の System.Reflection
名前空間は、コードのパラメーター、アセンブリ、メソッド、モジュール、およびその他の要素に関する情報を取得するために使用されます。 この名前空間をプログラムにインポートすることで、任意のクラス タイプ、パラメーター値、メソッドなどを取得できます。
System.Reflection
機能を使用してオブジェクトのプロパティ値を取得するテクニックを紹介します。
C#
でリフレクションを使用してプロパティの値を取得する
Visual Studio で C# プロジェクトを作成します。 まず、Reflection
名前空間をプログラムにインポートします。
using System.Reflection;
System
名前空間には、オブジェクト タイプを返すクラス Type
が含まれています。
Type type = _obj.GetType();
Reflection
名前空間には、型のプロパティを取得するための PropertyInfo
という名前のクラスが含まれています。 Type
クラスには、それぞれプロパティとクラスのプロパティの配列を返すメソッド GetProperty()
と GetProperties()
があります。
PropertyInfo[] properties = type.GetProperties();
PropertyInfo
クラスには、Name
および GetValue()
という名前のメンバー属性とメソッドが含まれており、プロパティの名前とそれに割り当てられた値をそれぞれ返します。
Console.WriteLine(p.Name + " : " + p.GetValue(_obj));
以下は、サンプル プロジェクトの完全なコード スニペットです。
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Reflection;
namespace GettingPropertyValue {
public class Book {
private String bookTitle;
private int numberOfPages;
public Book(String title, int pages) {
bookTitle = title;
numberOfPages = pages;
}
public String Title {
get { return bookTitle; }
}
public int Pages {
get { return numberOfPages; }
set { numberOfPages = value; }
}
}
public class MyGetValue {
public static void Main() {
Console.WriteLine("Getting all properties...");
Book book = new Book("Get Property Value in C#", 198);
GetPropertyValues(book);
Console.WriteLine("\n\nGetting value of a single property...");
GetPropertyValue(book, "Pages");
Console.ReadKey();
}
private static void GetPropertyValues(Object _obj) {
// returns the type of _obj
Type type = _obj.GetType();
Console.WriteLine("Type of Object is: " + type.Name);
// create an array of properties
PropertyInfo[] properties = type.GetProperties();
Console.WriteLine("Properties (Count = " + properties.Length + "):");
// print each property name and value
foreach (var p in properties)
if (p.GetIndexParameters().Length == 0)
Console.WriteLine(p.Name + "(" + p.PropertyType.Name + ") : " + p.GetValue(_obj));
else
Console.WriteLine(" {0} ({1}): <Indexed>", p.Name, p.PropertyType.Name);
}
// method to get a single property value from a string
private static void GetPropertyValue(Object _obj, string property) {
// prints property name
Console.WriteLine("Property : " + _obj.GetType().GetProperty(property).Name);
// prints property value
Console.WriteLine("Value : " + _obj.GetType().GetProperty(property).GetValue(_obj));
}
}
}
2つのプロパティ (タイトルとページ数) を持つ Book
という名前のクラスを作成しました。 別のクラス MyGetValue
に 2つのメソッド (つまり、GetPropertyValue
と GetPropertyValues
) を作成しました。
GetProjectValue(Object _obj, string property)
は、オブジェクトと文字列またはプロパティをパラメーターとして取り、プロパティ名と値を出力として出力します。
一方、GetPropertyValues(Object _obj)
メソッドはオブジェクトをパラメーターとして受け取ります。 最初にオブジェクトのタイプを判別し、そのオブジェクトの値とともにすべてのプロパティを取得します。
コード スニペットの出力は次のとおりです。
Getting all properties...
Type of Object is: Book
Properties (Count = 2):
Title(String) : Get Property Value in C#
Pages(Int32) : 198
Getting value of a single property...
Property : Pages
Value : 198
ギークは System.Reflection
C# にアクセスして、C# のリフレクションの詳細なリファレンスを参照できます。