How to Generate a UUID in C#
- Universally Unique Identifier or UUID
-
Use
Guid.NewGuid()
to Generate a UUID in C# -
Use
System.Security.Cryptography
to Generate a UUID in C# - GUID Format in C#
- Conclusion
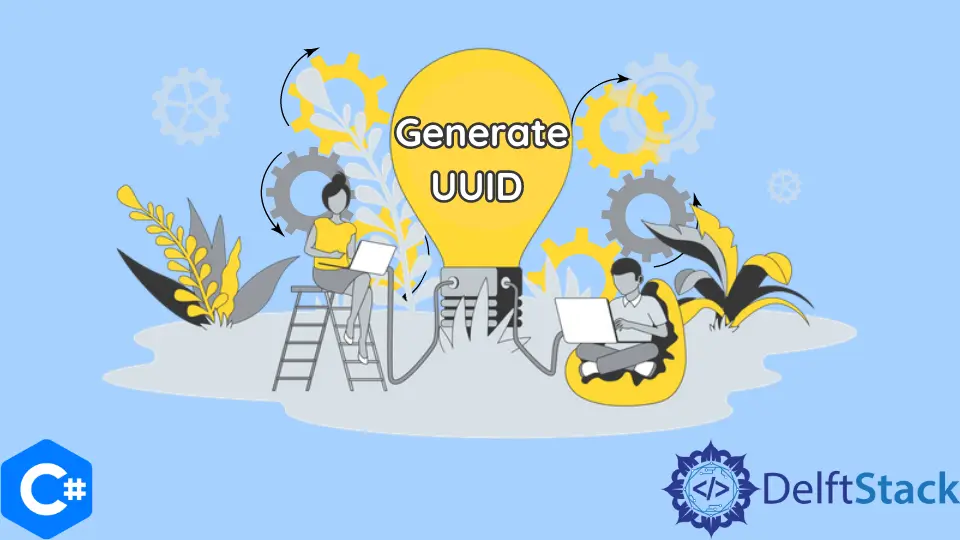
Universally Unique Identifier (UUID), also known as GUID (Globally Unique Identifier), is a unique identifier standard used in software development across various platforms.
In C#, there are different methods to generate UUIDs. Let’s explore these methods in detail.
Universally Unique Identifier or UUID
UUID stands for Universally Unique Identifier. It’s a standardized 128-bit format for a unique identifier, typically represented as a sequence of hexadecimal digits separated by hyphens.
UUIDs are used in software development and computer systems to uniquely identify information without the need for a central coordinating authority.
The uniqueness of a UUID is guaranteed across space and time, ensuring that no two UUIDs are the same, even if generated on different systems at different times. This uniqueness is achieved by combining various factors such as timestamps, unique machine identifiers, and random numbers.
Suppose we already have a primary key. In that case, we will utilize GUID because it is a 128-bit integer (16 bytes) with a low risk of duplicating, allowing it to be used across all databases and computers, avoiding data clash.
UUIDs are commonly used in various scenarios, including:
- Database Primary Keys: UUIDs can serve as unique identifiers for database records instead of auto-incrementing integers.
- Distributed Systems: They are valuable in distributed systems where multiple systems need to generate unique identifiers without central coordination.
- Software Development: UUIDs help uniquely identify entities or resources in software applications and systems.
There are different versions of UUIDs, each generated using various algorithms to ensure uniqueness. Some common UUID versions include UUID1 (based on timestamp and MAC address), UUID3 and UUID5 (based on hashing of a namespace identifier and name), and UUID4 (based on random or pseudo-random numbers).
UUIDs are represented in different formats, such as UUIDv1, UUIDv3, UUIDv4, and UUIDv5. Despite their format, the core principle remains the same: providing a universally unique identifier across different systems and platforms.
Use Guid.NewGuid()
to Generate a UUID in C#
The .Net
Framework contains built-in functionality for creating Version 4 UUIDs in the C# language.
In C#, the Guid.NewGuid()
method is used to generate a new, unique UUID (Universally Unique Identifier) or GUID (Globally Unique Identifier). The syntax for Guid.NewGuid()
is as follows:
Guid MyUniqueId = Guid.NewGuid();
This method belongs to the Guid
structure and is a static method that does not require an instance of Guid
to be created. It directly generates a new UUID and returns it as a Guid
structure.
Here’s a breakdown of the syntax:
Guid
: Refers to theGuid
structure in C#, which represents a globally unique identifier.MyUniqueId
: This is the variable name being declared. It’s of typeGuid
, indicating that it will store a UUID.NewGuid()
: This method generates a new UUID. It doesn’t take any parameters and directly produces a unique identifier every time it’s called.
The simplest and most common way to generate a UUID in C# is by using the Guid.NewGuid()
method. This method creates a new UUID based on random or pseudo-random numbers.
Example 1:
using System;
class Program {
static void Main() {
Guid uuid = Guid.NewGuid();
Console.WriteLine(uuid);
}
}
Output:
d36a1b06-9a88-4e60-b059-6a5e9fc5bbff
In this basic example, the Guid.NewGuid()
generates a new UUID and assigns it to the uuid
variable, then Console.WriteLine(uuid)
prints the generated UUID to the console.
Example 2:
using System;
using System.Diagnostics;
namespace MyUUIDGeneration {
class UUID {
public static void Main(string[] args) {
Guid myUUId = Guid.NewGuid();
string convertedUUID = myUUId.ToString();
Console.WriteLine("Current UUID is: " + convertedUUID);
}
}
}
Output:
Current UUID is: 123z0987-d23b-43gd-s566-000414174266
This code within the MyUUIDGeneration
namespace generates a new UUID (Universally Unique Identifier) using the Guid.NewGuid()
method. It converts this generated UUID into its string representation using ToString()
and prints it to the console with a message indicating "Current UUID is:"
.
Use System.Security.Cryptography
to Generate a UUID in C#
You can create a UUID using the System.Security.Cryptography
namespace, providing a cryptographically strong random number generator.
In C#, the System.Security.Cryptography
namespace provides classes and methods for cryptographic operations. Here is the basic syntax for using this namespace in C#:
using System.Security.Cryptography;
This using
directive allows access to the types and methods within the System.Security.Cryptography
namespace throughout the code file where it is included.
Once you’ve included this directive, you can access various cryptographic classes and methods available within the namespace to perform operations such as encryption, decryption, hashing, generating secure random numbers, digital signatures, and more.
Example:
using System;
using System.Security.Cryptography;
class Program {
static void Main() {
RNGCryptoServiceProvider rng = new RNGCryptoServiceProvider();
byte[] bytes = new byte[16];
rng.GetBytes(bytes);
Guid uuid = new Guid(bytes);
Console.WriteLine(uuid);
}
}
Output:
0dbae7ab-3bd7-0bd5-7551-d4eb5ec6a9bc
Explanation:
RNGCryptoServiceProvider()
creates a cryptographically secure random number generator.rng.GetBytes(bytes)
fills the byte arraybytes
with random bytes.new Guid(bytes)
generates a UUID based on the bytes obtained from the random number generator.Console.WriteLine(uuid)
prints the generated UUID.
GUID Format in C#
GUID is a structure found in the System
namespace. A string of hexadecimal integers is the most systematic approach to writing a GUID in the language.
Something like this: { 123z0987-d23b-43gd-s566-000414174266 }
.
GUID Identifier in C#
GUID is the name for this one-of-a-kind identifier. Generated by a Windows program, GUID can be used to determine a certain item, software, part, or repository record.
Consider a distributed system in which many programmers work independently and generate an ID. Two programmers may generate the same ID, causing a problem when the system is merged.
Therefore, we must use GUID when we have multiple independent systems or users generating IDs that must be unique. When we construct an interface or method in programming, we assign a unique ID that will be used to call it.
GUID prevents data collisions. It’s simple to integrate databases between various machines, networks, and so on. It helps you to determine your main key before inserting a record. It’s not easy to figure out.
Drawbacks of GUID in C#
Every GUID has both advantages and disadvantages. In a database with 10,000 entries, the GUID will take up 160000 bytes.
Another negative point is that most of the primary
keys in other tables are foreign keys. Only the GUID takes up around 60 bytes in only 5 FK entries, and it becomes the dominant source of performance concerns over time.
An out-of-order technique produces a random GUID. As an insertion timestamp, we must apply several methods for sorting.
Conclusion
The article explores UUIDs (Universally Unique Identifiers) in C#, covering their significance and practical applications in software development. It details various methods to generate UUIDs:
- Using
Guid.NewGuid()
: This method is demonstrated for straightforwardly generating UUIDs. Examples and syntax are provided, showcasing its simplicity and effectiveness. - Utilizing
System.Security.Cryptography
Namespace: The article explains how cryptographic functionalities can be used to create secure UUIDs. Code examples illustrate the process of generating UUIDs with cryptographic strength.
Additionally, the article discusses the format, utility, benefits, and drawbacks of GUIDs (Globally Unique Identifiers) in C#. It emphasizes their role as unique identifiers, addresses considerations for database implementations, and highlights the trade-offs involved in using GUIDs.
I have been working as a Flutter app developer for a year now. Firebase and SQLite have been crucial in the development of my android apps. I have experience with C#, Windows Form Based C#, C, Java, PHP on WampServer, and HTML/CSS on MYSQL, and I have authored articles on their theory and issue solving. I'm a senior in an undergraduate program for a bachelor's degree in Information Technology.
LinkedIn