WebSocket Client in C#
-
WebSocket in
C#
-
Create a WebSocket Client in
C#
-
Create a WebSocket Client With the Disposing Method in
C#
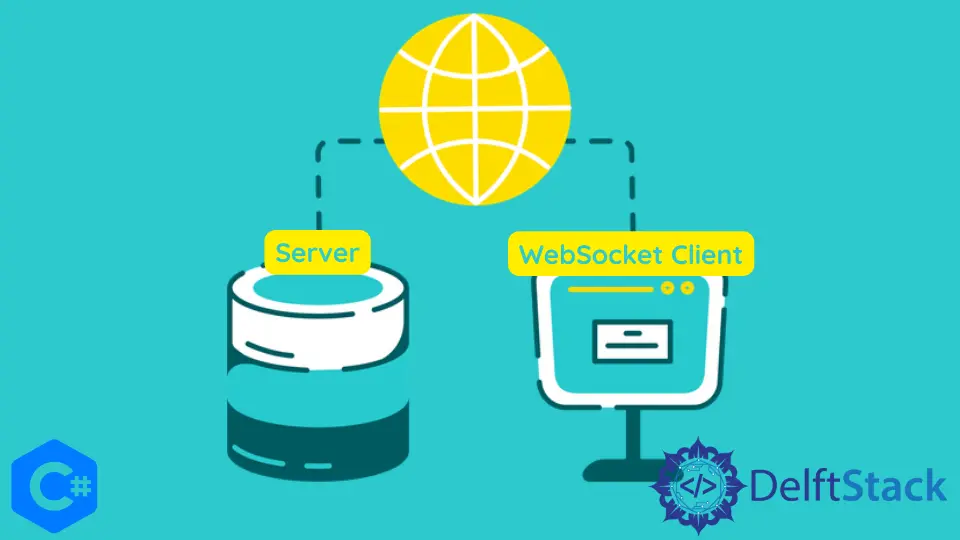
This guide will discuss what C# WebSocket is and how to establish a connection in C#. And finally, the article will tackle the WebSocket client in C# and how to write it.
So, without any further delay, let’s dive in.
WebSocket in C#
To properly understand the concept of WebSocket, we will have to look into the networking field. Now, WebSocket is a communication protocol that uses a TCP connection to provide a full-duplex connection for communication.
TCP enables a connection between servers so that they communicate. Since it’s a full-duplex communication, it also provides the opportunity to engage in communication between servers back and forth.
The WebSocket protocol enables a web browser to interact with a web server. Unlike other protocols like HTTP, which is a half-duplex communication, WebSocket enables the server and the client to engage in two-way communication without any interruptions fully.
The client would not need to request the content as the server will send it, making it easier for communication.
This also enables a stream of messages. WebSocket enables these messages that stream over TCP so that it deals with byte streams with no concept of a message of its own.
Establish a Connection (HandShaking)
A connection is established between a server and client through TCP, and TCP uses the Handshake concept. This means that the client requests permission to form a connection to the server, and the server then decides whether to form this connection or not.
Once the connection is formed, the Client and Server can communicate without interruption.
Use TCP Connection
You may know the other connection called UDP. Now the question arises: why use TCP and not UDP?
Well, first, UDP doesn’t provide the features that TCP does. There is no reliability, no proper connection.
The only advantage of using UDP would be its fast speed, but this reason is not enough to use this connection. So, we use TCP, which is reliable because of its Handshaking rule.
Create a WebSocket Client in C#
Now, the .NET framework has some support for WebSocket; it’s just that it’s a little tiresome process and extremely tricky to write the code from scratch. So we use a library specifically for WebSocket, WebSocketSharp
.
It is prevalent as it makes our programming experience much easier. To use this, we would have to download it in our software; recommended would be Visual Studio.
Let’s see how we will use this library to create a Client-side connection with our server. Note that in this example, we have to use the URL of the server we want to connect to and that it should always start with ws
since we are creating a WebSocket connection.
using System;
using System.Linq;
using System.Collections.Generic;
using System.Threading.Tasks;
using System.Text;
namespace WebSocket_Client {
public class Program {
static void Main(string[] args) {
// creating a client WebSocket
WebSocket webSocket = new WebSocket(
"ws://simple-websocket-server-echo.glich.me/"); // in this string, we will type URL of
// server we want to connect to
webSocket.OnMessage += webSocket_OnMessage; // message for the function below
webSocket.Connect(); // connection to server
webSocket.Send("Hello server, i am client"); // message to server
}
public static void webSocket_OnMessage(object viewer,
MessageEventArgs a) // function for server to respond
{
Console.WriteLine("Recived from the server " + a.Data);
}
}
}
Create a WebSocket Client With the Disposing Method in C#
Another way to write the Client-side code would be by using the using()
function. Using this function, we can dispose of the block of code written inside this function.
It is important to use this function since we are working with a TCP connection. As discussed above, in TCP, we establish a connection by the handshaking rule to secure our connection and increase its reliability.
But sometimes, the connection established isn’t properly closed. This can create problems in creating the connection again since, from the compiler’s point of view, the connection was never closed.
So to escape this loop of errors, we use the using()
function and write our block of code to properly execute and dispose of the connection.
using System;
using System.Linq;
using System.Collections.Generic;
using System.Threading.Tasks;
using System.Text;
namespace WebSocket_Client {
class Program {
static void Main(string[] args) {
using (WebSocket webSocket = new WebSocket(
"ws://simple-websocket-server-echo.glich.me/")) // write the URL of server to
// connect to
{
webSocket.OnMessage += webSocket_OnMessage; // message for the function below
webSocket.Connect(); // connection to the server
webSocket.Send("Hello server, i am client"); // sending a message to the server
}
}
public static void webSocket_OnMessage(object viewer,
MessageEventArgs a) // function for server to respond
{
Console.WriteLine("Recived from the server " + a.Data);
}
}
}
Haider specializes in technical writing. He has a solid background in computer science that allows him to create engaging, original, and compelling technical tutorials. In his free time, he enjoys adding new skills to his repertoire and watching Netflix.
LinkedIn