How to Wait for Event in C#
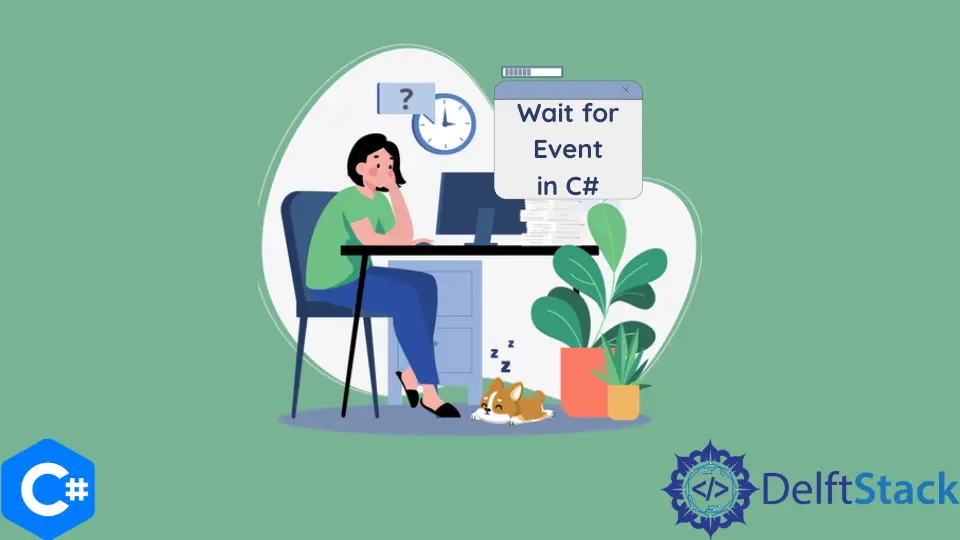
This programming article will briefly introduce Events in C# and then move toward waiting for an event in C#.
Events in C#
Events allow classes and objects to alert other classes and objects when certain things happen. The publisher class sends the event, and the classes that receive or handle it are known as subscribers.
Some events are declared by default, like button clicks, mouse events(mouse move, click, double click, etc.), keyboard events, and many others. But you can declare your events as well.
Event Handlers
Event handlers are the functions that are called when an event occurs. These handlers are automatically called when that event occurs and perform their action.
Multiple event handlers can be connected to an event, and when the event happens, they will all be called synchronously. Events that alert clients of an object’s changing state can also be handled by event handlers.
So we can say that event handlers are called when an event is fired, and you can give the code what you need to do when that event is fired in the event handler function.
Wait for an Event in C#
Things are very simple when you need to call a function on the fire of an event. For example, you need to call a function when a button is clicked.
When another event finishes, things become complicated when you need to call the function. This means you must make an event handler wait until another event completes its task.
Let’s demonstrate this with a simple example: two buttons and a text field. We need to click the first button, and when this button is clicked, we need to give the "Welcome text"
in the text field but display that text when the second button is also clicked.
We will first create a Form in a Windows form application and put three controls on it, 2 buttons and a text field like the following image.
We need to populate the text field when both button1
and button2
are clicked. To accomplish this task, we need to wait in the handler of button1
for button2
to finish its task and then set the text
of the text field.
The event handler function of button1
will use a class TaskCompletionSource
. This class will make the handler for button1
wait till the handler for button2
is finished.
Code:
TaskCompletionSource<bool> tcs = null;
private async void button1_Click(object sender, EventArgs e) {
tcs = new TaskCompletionSource<bool>();
label1.Text = "Click the Second Button";
await tcs.Task; // system will wait here
textBox1.Text = "You are done! ThankYou";
}
If we need a method to wait for another method, we declare that method as async
and then make that method await
. This will return at this point when the second task is completed.
private void button2_Click(object sender, EventArgs e) {
tcs.TrySetResult(true);
}
For the second button, when the button is clicked, the handler function above will be called. It will set the task completion to true, signaling to the awaited task that the other task is completed so that it will execute its remaining function.
Output:
When the first button is clicked, it also gives a message to click the second button. Let’s see what happens when the second button is clicked.
After clicking the second button, the text field shows the text which we set in the handler function of the first button. This means that the handler function of the first button was waiting for the second button to be clicked and then finishing running its remaining implementation.
In this way, we can wait for an event to finish its task.