Telnet Library in C#
-
The Telnet Library in
C#
-
Applications of the Telnet Library in
C#
-
Consequences of the Telnet Library in
C#
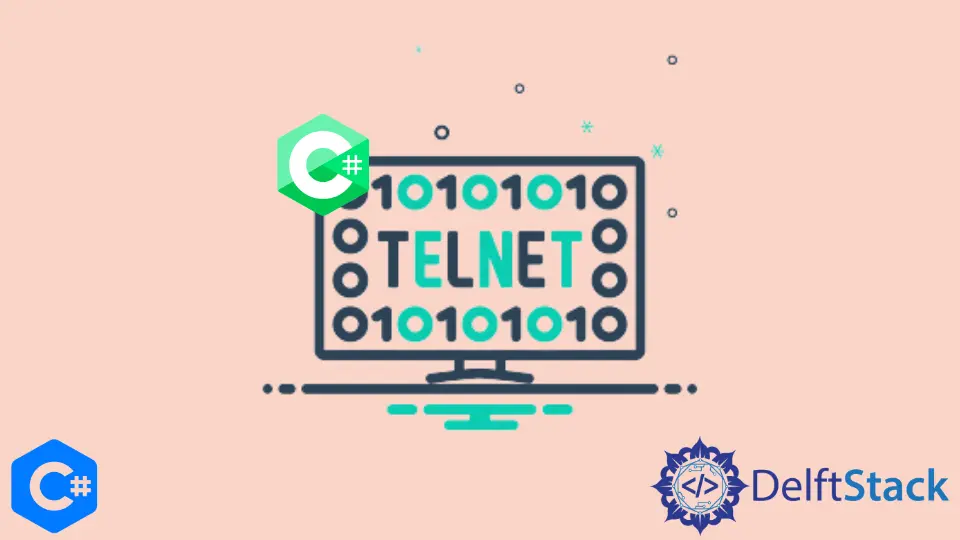
This tutorial will explain the Telnet library and demonstrate a simple Telnet interface in C#.
The Telnet Library in C#
Telnet is a networking protocol that allows you to virtually connect to a computer and establish a two-way, mutually supportive, text-based communication channel between two workstations.
It creates remote sessions using a user command Transmission Control Protocol/Internet Protocol (TCP/IP) networking protocol.
On the web, Hypertext Transfer Protocol (HTTP) and File Transfer Protocol (FTP) merely allow users to request specific files from remote computers.
However, Telnet allows users to log in as ordinary users with the capabilities provided to the specific applications and data on that machine.
Example:
The possible applications of this class include a Telnet terminal, a Windows GUI based on some UNIX commands that must be run on a server, and software that executes telnet scripts.
This code provides both a minimalistic terminal and the option to deploy a script through file piping.
To begin, import the following libraries:
using System;
using System.Collections.Generic;
using System.Text;
Create a Telnet
class in the namespace MinimalisticTelnet
to write the code.
namespace MinimalisticTelnet {
class Telnet {}
}
Create a new telnet connection named Tcon
to the hostname shani
on port 23
in the Main
function. The Telnet protocol usually uses port Port 23
.
TelnetConnection Tcon = new TelnetConnection("shani", 23);
Construct a string variable t
that will contain Login credentials such as root
, rootpassword
, and timeout
with the help of the Login()
method. We’ve supplied root
, rootpassword
, and a timeout
of 130 seconds.
It will show server output, as you can see in the following line of code.
string t = Tcon.Login("root", "rootpassword", 130);
Console.Write(t);
After getting the server output, we’ll verify the server output. It should conclude with $
or >
; otherwise, an exception will be thrown with the message Something went wrong, failed to connect
.
string Prmpt = t.TrimEnd();
Prmpt = t.Substring(Prmpt.Length - 1, 1);
if (Prmpt != "$" && Prmpt != ">")
throw new Exception("Something went wrong failed to connect ");
Prmpt = "";
Now, we’ll use a while
loop and pass the telnet connection Tcon
as a parameter to see if it’s connected or not. If it is connected, the loop will run; otherwise, it will terminate and display the message Disconnected
.
while (Tcon.IsConnected && Prmpt.Trim() != "exit") {
}
Console.WriteLine("Disconnected");
Console.ReadLine();
Lastly, write the following code in the body of the while
loop.
while (Tcon.IsConnected && Prmpt.Trim() != "exit") {
Console.Write(Tcon.Read());
Prmpt = Console.ReadLine();
Tcon.WriteLine(Prmpt);
Console.Write(Tcon.Read());
}
The server’s output will be shown in the following line of code.
Console.Write(Tcon.Read());
This will transmit all the client’s information or input to the server.
Prmpt = Console.ReadLine();
Tcon.WriteLine(Prmpt);
This code will display the server’s output when the client’s information has been entered.
Console.Write(Tcon.Read());
Example code:
using System;
using System.Collections.Generic;
using System.Text;
namespace MinimalisticTelnet {
class Telnet {
static void Main(string[] args) {
TelnetConnection Tcon = new TelnetConnection("shani", 23);
string t = Tcon.Login("root", "rootpassword", 130);
Console.Write(t);
string Prmpt = t.TrimEnd();
Prmpt = t.Substring(Prmpt.Length - 1, 1);
if (Prmpt != "$" && Prmpt != ">")
throw new Exception("Something went wrong failed to connect ");
Prmpt = "";
while (Tcon.IsConnected && Prmpt.Trim() != "exit") {
Console.Write(Tcon.Read());
Prmpt = Console.ReadLine();
Tcon.WriteLine(Prmpt);
Console.Write(Tcon.Read());
}
Console.WriteLine("Disconnected");
Console.ReadLine();
}
}
}
Applications of the Telnet Library in C#
Telnet allows users to connect to any program that uses text-based, unencrypted protocols, such as web servers and ports.
Telnet may be used to do a range of tasks on a server, including file editing, program execution, and email checking.
Various servers provide remote access to public data through Telnet, allowing users to play simple games or look up weather forecasts. Many of these features persist because they are nostalgic or compatible with earlier systems requiring certain data.
Consequences of the Telnet Library in C#
Telnet has a security flaw. It’s an unencrypted, non-secure protocol.
During a Telnet session, anyone monitoring the user’s connection can see the user’s login, password, and other private information written in plaintext. This data can be used to get access to the user’s device.
I have been working as a Flutter app developer for a year now. Firebase and SQLite have been crucial in the development of my android apps. I have experience with C#, Windows Form Based C#, C, Java, PHP on WampServer, and HTML/CSS on MYSQL, and I have authored articles on their theory and issue solving. I'm a senior in an undergraduate program for a bachelor's degree in Information Technology.
LinkedIn