How to Set Up Client-Server Communication in C#
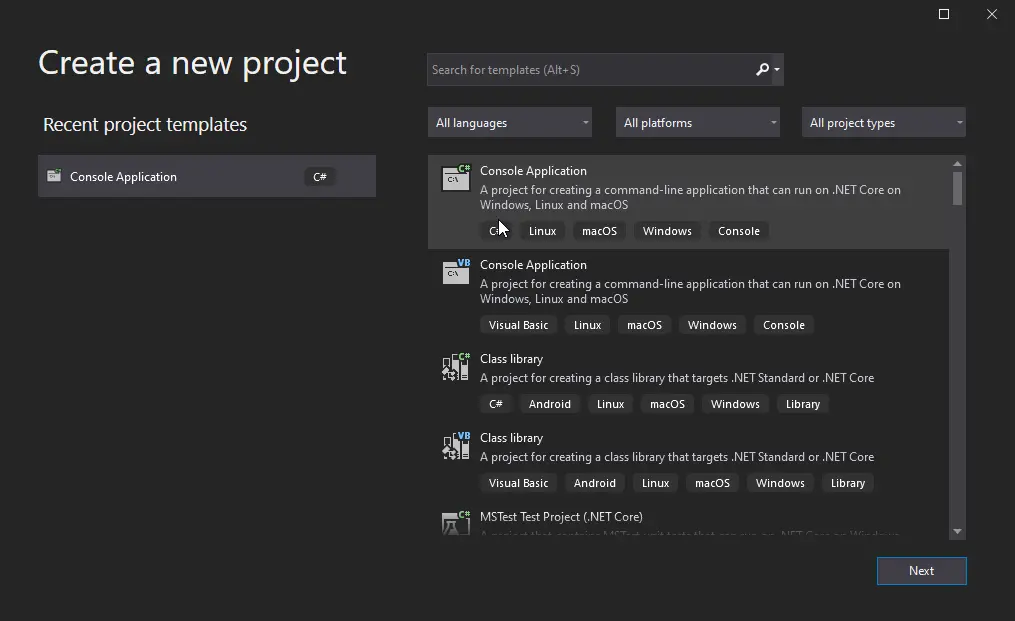
This trivial programming tutorial will discuss the data transmission in a client-server environment. It will also provide a C#-based implementation to the client-server communication model through TCP socket programming.
Transmission Control Protocol (TCP)
The Open Systems Interconnection (OSI) is a widely adopted communicational reference model which explains how computers can communicate with each other. It ensures that error-free data is delivered from one end to another.
The layered OSI model defines some rules and protocols to be followed when sending or receiving the data. All the layers work individually and perform their tasks in transmitting data from one end to the other.
This article will explain the Transport layer only. The main protocol used at this layer is transmission control protocol (TCP) and has the following key responsibilities:
- Establish a connection between two ends and then destroy that connection when communication is over.
- Transfer of data which consists of the following tasks:
- Ensures data reliability and checks if the data is transferred in sequential order and is error-free.
- Detect the error, if any, and resends the lost packets.
- Control the flow and rate of the data packets.
- Acknowledgment and set flags.
Set Up Client-Server Communication in C#
Let us move towards implementing the client/server application in the .NET framework using the C# language. We will be doing this implementation using Visual Studio Enterprise Edition 2019.
We will be creating two applications, one for the client-side and one for the server-side. For testing, both of these applications will reside on the same computer.
First, we will create a client application. For that, open Visual Studio and create a new project.
Then, select the project type Console Application
and click Next
.
Put the Project name as Client Application
and the Solution name as ClientServerApplication
. This will create a new project for the client-side.
For server-side programming, create another project in the same solution:
This project will be named ServerApplication
and in the same solution.
After creating the projects, you must add the Network Comms Dot Net package files. For that, you need to install them using the NuGet console.
Go to Tools > NuGet Package Manager > Package Manager Console
like this in the image:
You will get the Package Manager Console in the window, as highlighted in the image above. In this console, we will write the command:
PM > Install - Package NetworkCommsDotNet - Version 3.0.3
After successfully installing the package in both projects, you can start coding. Open the ServerApplication
project and write the code:
public static void ConnectServer() {
IPHostEntry myhost = Dns.GetHostEntry("localhost");
IPAddress ipAddr = myhost.AddressList[0];
IPEndPoint myEndPoint = new IPEndPoint(ipAddr, 11000);
try {
Socket listenerSocket = new Socket(ipAddr.AddressFamily, SocketType.Stream, ProtocolType.Tcp);
listenerSocket.Bind(myEndPoint);
listenerSocket.Listen(5);
Console.WriteLine("Waiting for Client Socket to Coonect...");
Socket socketHandler = listenerSocket.Accept();
// data from the client.
string myData = null;
byte[] dataBytes = null;
while (true) {
dataBytes = new byte[1023];
int bytesRece = socketHandler.Receive(dataBytes);
myData += Encoding.ASCII.GetString(dataBytes, 0, bytesRece);
if (myData.IndexOf("<EOF>") > -1) {
myData = myData.Remove(myData.Length - 5);
break;
}
}
Console.WriteLine("Data Received from Client : {0}", myData);
byte[] msg = Encoding.ASCII.GetBytes("This is the test msg from server");
socketHandler.Send(msg);
socketHandler.Shutdown(SocketShutdown.Both);
socketHandler.Close();
} catch (Exception e) {
Console.WriteLine(e.ToString());
}
Console.WriteLine("\n Press any key to continue...");
Console.ReadKey();
}
Then on the client-side, write the following code:
public static void ConnectClient() {
byte[] myBytes = new byte[1024];
try {
IPHostEntry myhost = Dns.GetHostEntry("localhost");
IPAddress ipAddr = myhost.AddressList[0];
IPEndPoint clientEP = new IPEndPoint(ipAddr, 11000);
Socket senderSocket = new Socket(ipAddr.AddressFamily, SocketType.Stream, ProtocolType.Tcp);
try {
// Connect to the EndPoint
senderSocket.Connect(clientEP);
Console.WriteLine("Client Connect to Server at {0}", senderSocket.RemoteEndPoint.ToString());
// Convert the string into a byte-type array.
Console.WriteLine("Sending a test message");
byte[] msg = Encoding.ASCII.GetBytes("This is a test message from Client<EOF>");
// Send the data using the socket.
int dataSent = senderSocket.Send(msg);
// Receive the reply from the server device.
int dataRec = senderSocket.Receive(myBytes);
Console.WriteLine("Data Received from Server = {0}",
Encoding.ASCII.GetString(myBytes, 0, dataRec));
// delete the socket
senderSocket.Shutdown(SocketShutdown.Both);
senderSocket.Close();
Console.ReadKey();
} catch (ArgumentNullException ane) {
Console.WriteLine(ane.ToString());
} catch (SocketException se) {
Console.WriteLine(se.ToString());
} catch (Exception e) {
Console.WriteLine(e.ToString());
}
} catch (Exception e) {
Console.WriteLine(e.ToString());
}
}
After you call these functions in the main function in the corresponding projects, you need to build the project, or you can build the entire solution. Then, for running the output, navigate to the file explorer of these projects and run the exe
file of both.
The following output is received:
Husnain is a professional Software Engineer and a researcher who loves to learn, build, write, and teach. Having worked various jobs in the IT industry, he especially enjoys finding ways to express complex ideas in simple ways through his content. In his free time, Husnain unwinds by thinking about tech fiction to solve problems around him.
LinkedIn