How to Create StringStream in C#
-
Use the Tandem of
MemoryStream
andStreamReader
Classes to CreateStringStream
inC#
-
Create a
StringStream
Class inC#
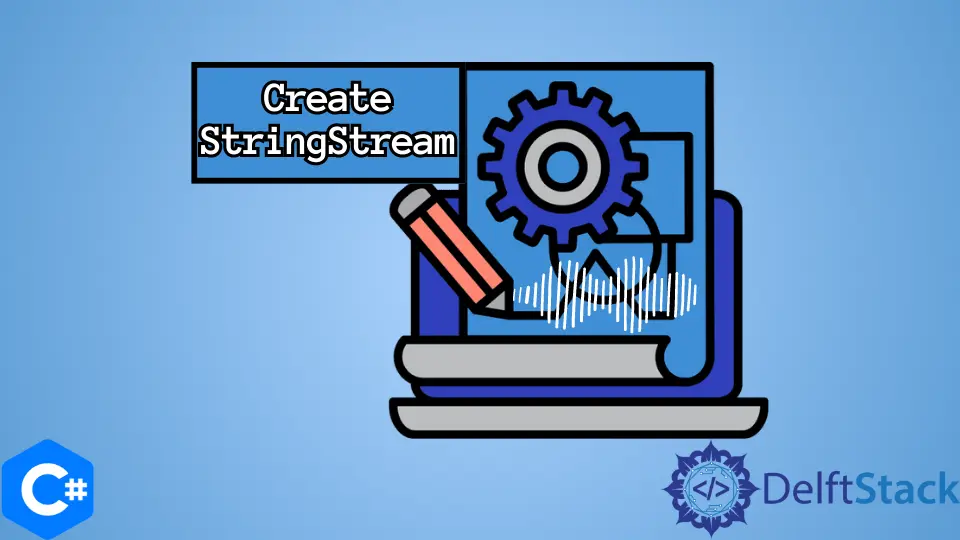
In C#, StringStream
is derived from Stream
with the help of StringBuilder
. Its primary purpose is to accept and pass the data currently stored only as a string into a method.
In this tutorial, you will learn multiple approaches to creating StringStream
in C#. The easiest way to derive a StringStream
from MemoryStream
is by taking advantage of its constructer that accepts the byte array of data contained within the Stream
.
Use the Tandem of MemoryStream
and StreamReader
Classes to Create StringStream
in C#
Use StreamReader
to read characters to stream in a specified encoding, and it is inherited from TextReader
that provides methods to read characters from the input stream. System.IO.Stream
as an abstract class provides methods to transfer bytes to the source.
The MemoryStream
inherit the Stream
class and provide the functionality to read and write data (in bytes) from a particular source. It plays a crucial part in the creation of StringStream
in C#.
// add `System.IO` library to your C# project
using System;
using System.IO;
using System.Text;
namespace createStringStream {
class StreamClass {
static void Main(string[] args) {
// creation of StringStream object for communication or data transfer
string StringStream;
using (var objStream = new MemoryStream()) {
Console.WriteLine(objStream);
// initial postition of the `MemoryStream` object
objStream.Position = 0;
using (var reader = new StreamReader(objStream)) {
// StringStream creation and initialization
StringStream = reader.ReadToEnd();
}
}
}
}
}
In StringStream
, passing data from the source to the MemoryStream
constructer requires data to be a MemoryStream
object. Use the GetBytes Encoding
method to create a byte array.
Create a StreamReader
object to convert a Stream
object to a C# string and call the ReadToEnd
method.
// add `System.IO` before executing the C# code
using System;
using System.IO;
using System.Text;
namespace convertVar {
class StringtoStream {
static void Main(string[] args) {
// declare a string variable
string test = "Experiment values: 3.1437";
// for converting string to stream
byte[] byteArray = Encoding.ASCII.GetBytes(test);
MemoryStream stream = new MemoryStream(byteArray);
// for converting stream to string
StreamReader reader = new StreamReader(stream);
string text = reader.ReadToEnd();
// output
Console.WriteLine(text);
Console.ReadLine();
}
}
}
Create a StringStream
Class in C#
Unlike Java, C# doesn’t have a pre-build StringStream
class. It’s easy to make a StringStream
class object to fulfill the purpose using MemoryStream
and StreamBuilder
.
However, you can create a StringStram
class with the help of the following C# code.
// add `System.IO` referance to your C# project to create `StringStream` class
using System;
using System.Text;
using System.Windows.Forms;
using System.IO;
namespace userStringStream {
public partial class Form1 : Form {
public Form1() {
InitializeComponent();
}
// a click even for `Create StringStream` button
private void button2_Click(object sender, EventArgs e) {
string s0 =
"It's an example data the `s0` string contains. Ur fndf, isnd sdnin.\r\n" +
"Ientif nt ieuth nnsh riwnw htune iw o nwinundhsi a xmunc, dusn ainde efn. Dncisdns asnw eunc usn sdu s n xcuxc sd c.\r\n";
// create `StringStream` class objects to access its functionality and methods
StringStream ss0 = new StringStream(s0);
StringStream ss1 = new StringStream();
int line = 1;
Console.WriteLine("Contents of input stream: \n");
using (StreamReader reader = new StreamReader(ss0)) {
using (StreamWriter writer = new StreamWriter(ss1)) {
while (!reader.EndOfStream) {
string s = reader.ReadLine();
Console.WriteLine("Line " + line++ + ": " + s);
writer.WriteLine(s);
Console.WriteLine();
}
}
}
Console.WriteLine("Contents of output stream: \n");
Console.Write(ss1.ToString());
}
}
// the creation of `StringStream` class
public class StringStream : Stream {
// create `memoryStream` object for data assigning through `MemoryStream`
private readonly MemoryStream memoryStream;
// In genral, `memoryStream` is named as `_memory` similar to Java
public StringStream(string text) {
memoryStream = new MemoryStream(Encoding.UTF8.GetBytes(text));
}
public StringStream() {
memoryStream = new MemoryStream();
}
public StringStream(int capacity) {
memoryStream = new MemoryStream(capacity);
}
public override void Flush() {
memoryStream.Flush();
}
public override int Read(byte[] buffer, int offset, int count) {
return memoryStream.Read(buffer, offset, count);
}
public override long Seek(long offset, SeekOrigin origin) {
return memoryStream.Seek(offset, origin);
}
public override void SetLength(long value) {
memoryStream.SetLength(value);
}
public override void Write(byte[] buffer, int offset, int count) {
memoryStream.Write(buffer, offset, count);
}
public override bool CanRead => memoryStream.CanRead;
public override bool CanSeek => memoryStream.CanSeek;
public override bool CanWrite => memoryStream.CanWrite;
public override long Length => memoryStream.Length;
public override long Position {
get => memoryStream.Position;
set => memoryStream.Position = value;
}
public override string ToString() {
return System.Text.Encoding.UTF8.GetString(memoryStream.GetBuffer(), 0,
(int)memoryStream.Length);
}
public override int ReadByte() {
return memoryStream.ReadByte();
}
public override void WriteByte(byte value) {
memoryStream.WriteByte(value);
}
}
}
Stream
provides a generic interface to the input and output types in C#. With the help of MemoryStream
and StreamReader
classes, developers can isolate themselves from the specific details of the underlying devices and OS.
Hence, StringStream
is vital to communicate between devices by receiving, processing, and transmitting data without complicating the Stream
.
Hassan is a Software Engineer with a well-developed set of programming skills. He uses his knowledge and writing capabilities to produce interesting-to-read technical articles.
GitHub