Simple State Machine Example in C#
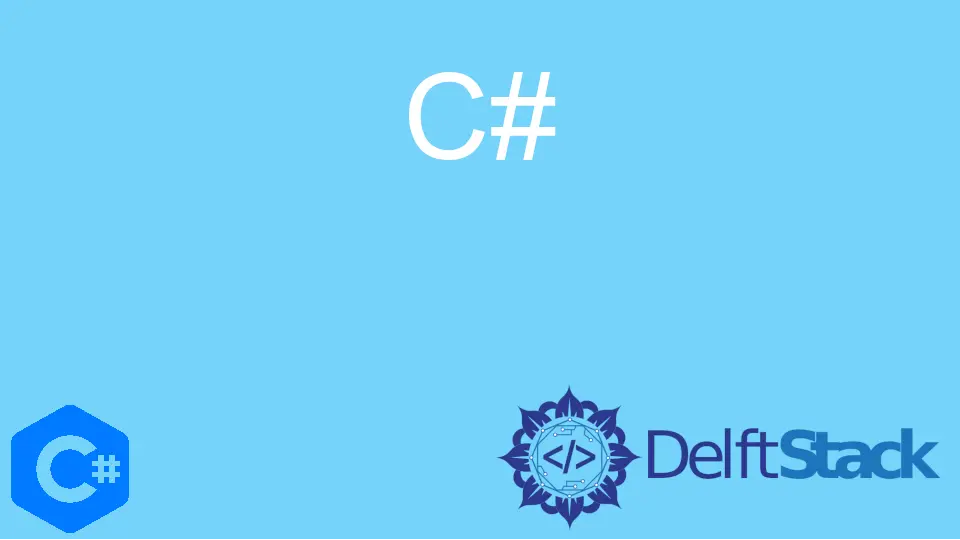
This article will walk you through an example of a basic state machine using the programming language C#. Let’s first look at the State machine.
State machines are any electronic apparatus that may transition from one state to another in response to external stimuli. Take, for instance, a bank teller machine, a remote control, the actual computer, etc.
Simple State Machine Example in C#
Let’s start building a basic state machine using one state pattern in the .NET
framework. Once the system has been activated, the state machine we will construct will be an IT Employee Working
system, which modifies the signals at certain intervals.
To begin, import the following libraries.
using System;
using System.Threading;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
Make a state interface called SignalState
, which all the states will use.
public interface SignalState {
void Enter(Worker system);
}
Next, let’s go ahead and set up the different states for the Worker
. The initial state is denoted by GoogleChrome
.
Let’s suppose the worker will finish his Google Chrome work in this state. It will last for 3 seconds.
public class GoogleChrome : SignalState {
const int time = 3000;
public void Enter(Worker system) {
Console.WriteLine("Working in Google Chrome");
Thread.Sleep(time);
system.NextWorkSignal = new MSWord();
system.ChangeSignal();
}
}
The next state will be MS Word
, where workers will work on Microsoft Word. It will also last for 3 seconds.
public class MSWord : SignalState {
const int time = 3000;
public void Enter(Worker system) {
Console.WriteLine("Working in MS Word now");
Thread.Sleep(time);
system.NextWorkSignal = new SendingtoClient();
system.ChangeSignal();
}
}
After completing his work on GoogleChrome
and MSWord
, the last state will be SendingtoClient
.
public class SendingtoClient : SignalState {
const int time = 3000;
public void Enter(Worker system) {
Console.WriteLine("Now Sending Work to My Client");
Thread.Sleep(time);
system.NextWorkSignal = new GoogleChrome();
system.ChangeSignal();
}
}
Now, we’ll create a Worker
class that will change the working state of Worker
.
public class Worker {
public SignalState NextWorkSignal { get; set; }
public void Start() {
NextWorkSignal = new MSWord();
NextWorkSignal.Enter(this);
}
public void ChangeSignal() {
Console.WriteLine("Work Changed");
NextWorkSignal.Enter(this);
}
}
Complete Source Code:
using System;
using System.Threading;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace Worker {
public interface SignalState {
void Enter(Worker system);
}
public class GoogleChrome : SignalState {
const int time = 3000;
public void Enter(Worker system) {
Console.WriteLine("Working in Google Chrome");
Thread.Sleep(time);
system.NextWorkSignal = new MSWord();
system.ChangeSignal();
}
}
public class MSWord : SignalState {
const int time = 3000;
public void Enter(Worker system) {
Console.WriteLine("Working in MS Word now");
Thread.Sleep(time);
system.NextWorkSignal = new SendingtoClient();
system.ChangeSignal();
}
}
public class SendingtoClient : SignalState {
const int time = 3000;
public void Enter(Worker system) {
Console.WriteLine("Now Sending Work to My Client");
Thread.Sleep(time);
system.NextWorkSignal = new GoogleChrome();
system.ChangeSignal();
}
}
public class Worker {
public SignalState NextWorkSignal { get; set; }
public void Start() {
NextWorkSignal = new MSWord();
NextWorkSignal.Enter(this);
}
public void ChangeSignal() {
Console.WriteLine("Work Changed");
NextWorkSignal.Enter(this);
}
}
class Program {
static void Main(string[] args) {
Worker system = new Worker();
system.Start();
Console.ReadLine();
}
}
}
Output:
Working in MS Word now
Work Changed
Now Sending Work to My Client
Work Changed
Working in Google Chrome
Work Changed
Working in MS Word now
Work Changed
Now Sending Work to My Client
Work Changed
Working in Google Chrome
Work Changed
Working in MS Word now
......
I have been working as a Flutter app developer for a year now. Firebase and SQLite have been crucial in the development of my android apps. I have experience with C#, Windows Form Based C#, C, Java, PHP on WampServer, and HTML/CSS on MYSQL, and I have authored articles on their theory and issue solving. I'm a senior in an undergraduate program for a bachelor's degree in Information Technology.
LinkedIn