How to Hash a String With SHA256 in C#
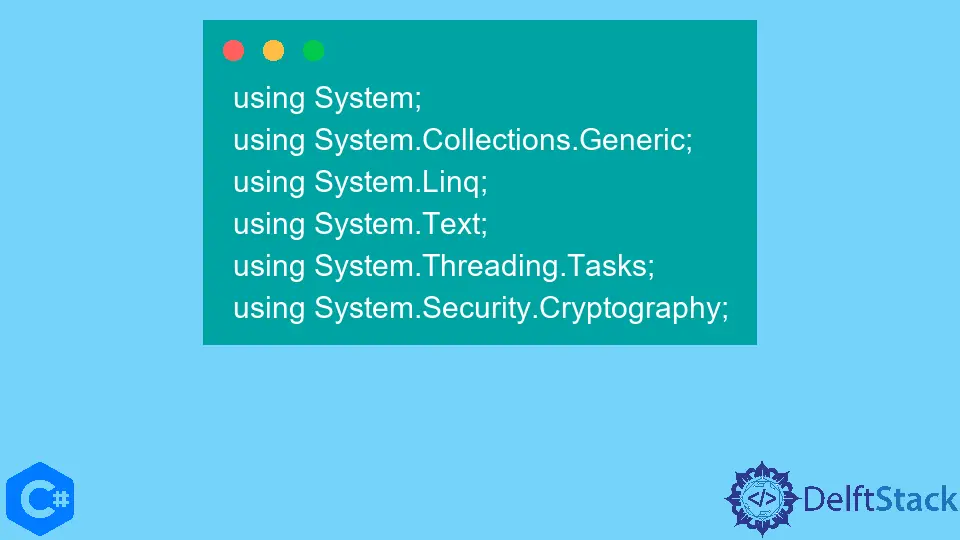
In the following article, you will learn how to hash a string using the sha256
algorithm in the C# programming language.
In cryptography, hashing refers to a method that maps a binary string of an indeterminate length to a short binary string with a defined length. This short binary string is known as a hash.
Hash functions are another name for hashing. It is standard practice to use hash functions to protect confidential information like passwords and digital certificates against unauthorized access.
Hash a String With SHA256 in C#
To better explain how to hash a string using sha256
, the following example can guide you.
To begin, import the following libraries:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Security.Cryptography;
First, we’ll create a function named HashWithSha256
that will convert user input to hash output. This function will take user input as a parameter.
static string HashWithSha256(string ActualData) {}
To obtain the SHA256 hash, we will utilize the SHA256
class. It is necessary to first create a SHA256
hash object by using the .Create()
method.
using (SHA256 s = SHA256.Create()) {}
Then, use the Encoding.GetBytes()
function to convert the provided string into a byte
array.
byte[] bytes = s.ComputeHash(Encoding.UTF8.GetBytes(ActualData));
Utilize the StringBuilder
to convert the byte
array into a string
.
StringBuilder b = new StringBuilder();
for (int i = 0; i < bytes.Length; i++) {
b.Append(bytes[i].ToString("x2"));
}
Let’s start by constructing the string we want to convert.
string s1 = "Muhammad Zeeshan";
Console.WriteLine("User Input: {0}", s1);
Lastly, you will need to store the output of the input string to a local variable HashString
after you provide it as a parameter to the HashWithSha256
function.
string HashString = HashWithSha256(s1);
Console.WriteLine("Hash Output: {0}", HashString);
Console.ReadLine();
Complete Source Code:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Security.Cryptography;
namespace HashStringByZeeshan {
class Program {
static void Main(string[] args) {
string s1 = "Muhammad Zeeshan";
Console.WriteLine("User Input: {0}", s1);
string HashString = HashWithSha256(s1);
Console.WriteLine("Hash Output: {0}", HashString);
Console.ReadLine();
}
static string HashWithSha256(string ActualData) {
using (SHA256 s = SHA256.Create()) {
byte[] bytes = s.ComputeHash(Encoding.UTF8.GetBytes(ActualData));
StringBuilder b = new StringBuilder();
for (int i = 0; i < bytes.Length; i++) {
b.Append(bytes[i].ToString("x2"));
}
return b.ToString();
}
}
}
}
Output:
User Input: Muhammad Zeeshan
Hash Output: b77387c5681141bfb72428c7ee23b67ce1bc08f976954368fa9cab070ffb837b
I have been working as a Flutter app developer for a year now. Firebase and SQLite have been crucial in the development of my android apps. I have experience with C#, Windows Form Based C#, C, Java, PHP on WampServer, and HTML/CSS on MYSQL, and I have authored articles on their theory and issue solving. I'm a senior in an undergraduate program for a bachelor's degree in Information Technology.
LinkedIn