REST API in C#
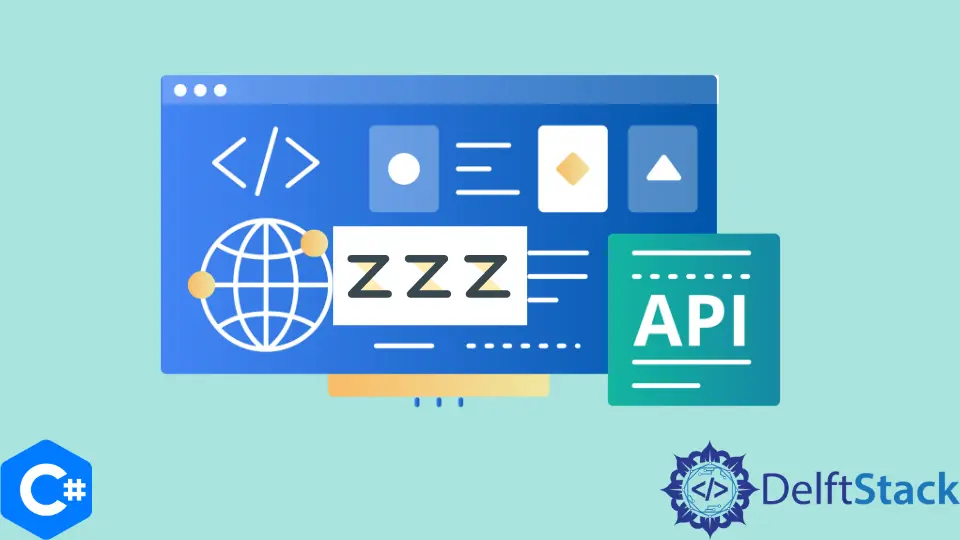
This tutorial will discuss the methods to make a REST API call in C#.
REST API Call With the RestSharp
Client in C#
The RestSharp
is probably the most popular REST API client in C#. We can cast the data received from the API into Plain Old Class Objects (POCO) with this client. For this purpose, We first have to make a data model class that contains fields to be returned by the API call. The following code example shows a sample data model class in C#. The RestSharp
client is a third-party package and does not come pre-installed. We need to install the RestSharp
package for this approach to work.
class dataModel {
public int UserID { get; set; }
public string UserName { get; set; }
}
The above dataModel
class can save the users’ ID and name returned in the API call response. The following code example shows us how we can execute an API call with the RestSharp
client in C#.
Uri Url = new Uri("https://exampleUrl.com");
IRestClient restClient = new RestClient(Url);
IRestRequest restRequest = new RestRequest(
"get", Method.GET) { Credentials = new NetworkCredential("Admin", "strongpassword") };
IRestResponse<dataModel> restResponse = restClient.Execute<dataModel>(restRequest);
if (restResponse.IsSuccessful) {
dataModel model = restResponse.Data;
} else {
Console.WriteLine(restResponse.ErrorMessage);
}
In the above code, we made a GET request to a rest API with the RestSharp
client in C#. We created a class that holds the data returned by the API called the dataModel
class. We then executed our request and saved the data returned in the response in an instance of the dataModel
class.
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn