Question Mark in C#
- Understanding the Question Mark in C#
- The Dot Operator and Null Conditional Operator
- Benefits of Using the Question Mark in C#
- Conclusion
- FAQ
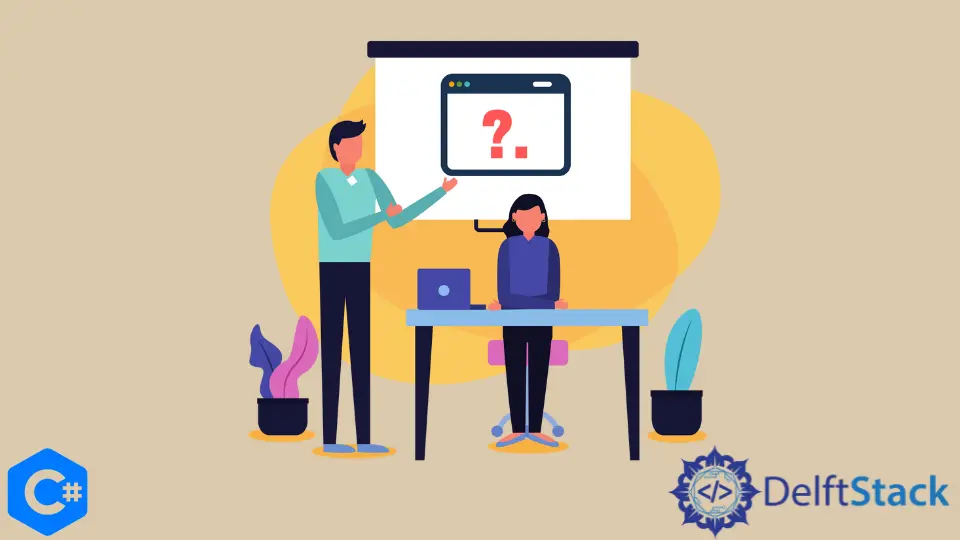
In the world of C#, the question mark is a powerful symbol that signifies more than just a punctuation mark. It plays a crucial role in handling null values and provides a more elegant way to access members of potentially null objects. Understanding the question mark and dot operator, particularly in the context of the Null Conditional operator, can significantly enhance your coding efficiency and reduce errors.
In this article, we will delve into the meaning of the question mark in C#, explore its applications, and demonstrate how it can streamline your code. Whether you’re a novice or an experienced developer, grasping these concepts will undoubtedly improve your programming skills.
Understanding the Question Mark in C#
The question mark in C# primarily serves two key purposes: as a part of nullable types and in the context of the Null Conditional operator. Nullable types allow you to assign null to value types, which is particularly useful when dealing with databases or optional data. For instance, instead of having a standard integer that cannot be null, you can declare a nullable integer using the question mark syntax. This provides flexibility in your code and helps avoid common pitfalls associated with null references.
Here’s how you can declare a nullable integer:
int? nullableInt = null;
In this example, nullableInt
can hold either an integer value or null, making it versatile for various scenarios. This is especially useful when working with data that may not always be present, such as user inputs or database queries.
Output:
nullableInt = null
The Dot Operator and Null Conditional Operator
The dot operator (.) is used in C# to access members of a class or structure. However, when you’re dealing with objects that might be null, using the dot operator can lead to a NullReferenceException
. To mitigate this risk, C# introduced the Null Conditional operator, represented by the question mark followed by a dot (?.
). This operator allows you to safely access members of an object without having to explicitly check for null.
Consider the following example where we have a class Person
that has a property Address
:
public class Person
{
public Address Address { get; set; }
}
public class Address
{
public string City { get; set; }
}
Person person = null;
string cityName = person?.Address?.City;
In this code, if person
is null, cityName
will also be null instead of throwing an exception. The use of ?.
ensures that the code remains clean and avoids unnecessary null checks.
Output:
cityName = null
Benefits of Using the Question Mark in C#
The primary advantage of employing the question mark in C# is the reduction of boilerplate code. Instead of writing multiple null checks, you can use the Null Conditional operator to streamline your code. This not only makes your code more readable but also less error-prone.
Furthermore, using nullable types allows for better database interactions, as they can represent the absence of a value without resorting to complex logic. This leads to cleaner, more maintainable code that is easier to debug and understand.
Consider a method that retrieves the city name from a person’s address. Without the Null Conditional operator, you might write:
if (person != null && person.Address != null)
{
return person.Address.City;
}
return null;
With the question mark, you can simplify this to:
return person?.Address?.City;
This concise approach not only enhances readability but also minimizes the risk of runtime errors.
Output:
cityName = null
Conclusion
The question mark in C# serves as a vital tool for developers, enabling the handling of null values gracefully and efficiently. By understanding its role in nullable types and the Null Conditional operator, you can write cleaner, more maintainable code. This not only improves your programming skills but also enhances your overall development experience. Embracing these concepts will empower you to tackle complex coding challenges with confidence.
FAQ
-
what is the purpose of the question mark in C#?
The question mark is used to define nullable types and to safely access members of potentially null objects using the Null Conditional operator. -
how do I declare a nullable type in C#?
You can declare a nullable type by appending a question mark to the type, like this:int? myNullableInt = null;
. -
what is the Null Conditional operator?
The Null Conditional operator (?.
) allows you to access properties or methods of an object without throwing aNullReferenceException
if the object is null. -
can I use the question mark with reference types?
Yes, the question mark can be used with value types to make them nullable, but reference types can naturally hold null values without needing a question mark. -
how does using the question mark improve code readability?
It reduces the amount of code needed for null checks, making the code cleaner and easier to understand at a glance.
#, focusing on its role in nullable types and the Null Conditional operator. Learn how this powerful symbol can enhance your coding efficiency, reduce errors, and improve overall code readability. Whether you’re a beginner or an experienced developer, understanding the question mark will empower you to write cleaner and more maintainable code in C#.