C# Private Set
- Understanding C# Properties
-
Use the Getters as a
get
Accessor in C# -
Use the Setters as a
set
Accessor in C# -
Introducing
private set
in C# -
Use Cases for
private set
in C# -
Advantages of Using
private set
in C# - Potential Pitfalls and Considerations
- Conclusion
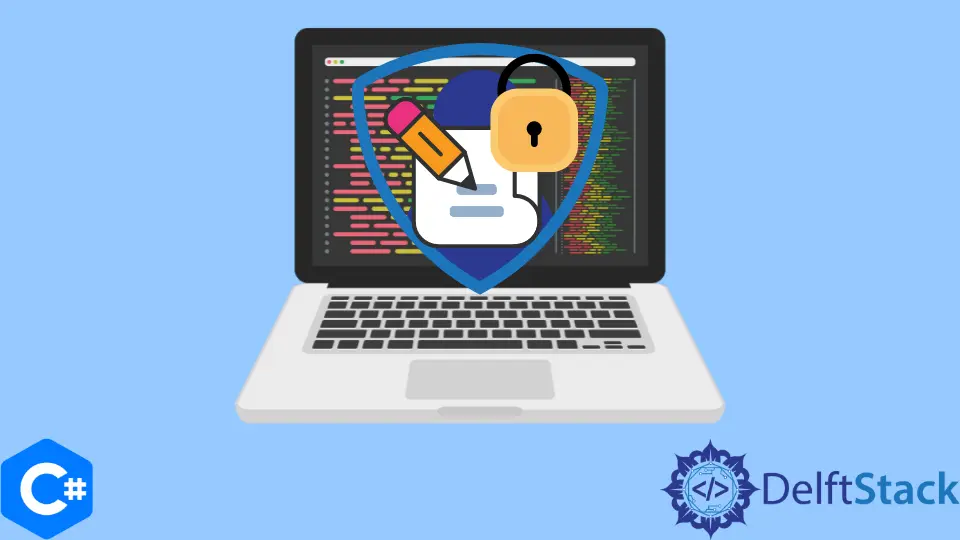
C# is a versatile and powerful programming language that continues to evolve with each new version, providing developers with features that enhance code readability, maintainability, and security. One such feature is the ability to use a private set
, which allows developers to control the write access to a property within a class.
In this article, we will look into the intricacies of the C# private set
, exploring its syntax, use cases, advantages, and potential pitfalls.
Understanding C# Properties
In C#, properties are used to encapsulate the state of an object, providing a way to access and modify its values. A property is typically defined with a getter and a setter method, allowing developers to read and write its value, respectively.
However, there are scenarios where restricting the write access to a property becomes crucial for maintaining the integrity of the class.
Properties in C# are a combination of fields and methods. They are not variables; hence, they can’t be passed as out
or ref
parameters in functions.
They help control access to data, perform validation of data before editing, and promote cleaner code as it avoids the declaration of explicit private
variables with the help of getters and setters.
The Basics of C# Properties
Let’s start by revisiting the basic syntax of C# properties:
public class MyClass {
private int myProperty;
public int MyProperty {
get { return myProperty; }
set { myProperty = value; }
}
}
In the example above, MyProperty
is a simple property with both getter and setter methods. This allows any external code to read and modify the myProperty
value.
While this is suitable for many situations, there are cases where a more restricted approach is desirable.
Before diving deep into the usage of private setters, let’s first discuss the concepts of getters and setters and slowly transition to the roles of access modifiers.
Use the Getters as a get
Accessor in C#
The code for getters is executed when reading the value. Since it is a read
operation on a field, a getter must always return the result.
A public getter means that the property is readable by everyone. In contrast, the private getter implies that the property can only be read by class and, hence, is a write-only property.
We have to choose between getters according to our requirements.
Use the Setters as a set
Accessor in C#
The code for setters is executed when writing the value. It is a write
operation and hence is a void
method.
It takes the value of the field as an argument. The value may be assigned to the property in its current form, or we can perform some computations before assigning it.
The public setter means that the value is editable by any object present outside the class. On the other hand, the private setter means that the property is read-only and can’t be modified by others.
Now that we know the difference between getter, setter, and the impact of accessor modifiers, we can look specifically at private setters.
public int Prop { get; private set; }
// The code below is the same as above. See the cleanliness use of auto property brings.
private int prop;
public int Prop {
get { return prop; }
}
We can see from the above code that using private setters is not just good coding practice but makes our code inherently encapsulated.
Encapsulation means that the objects in our class stick to an interface and always maintain their state. Here, denying the property to be editable from the outside makes classes more robust and performant.
Introducing private set
in C#
The private set
in C# allows developers to limit the write access to a property within the class that defines it. Let’s modify the previous example to include a private set
:
public class MyClass {
private int myProperty;
public int MyProperty {
get { return myProperty; }
private
set { myProperty = value; }
}
}
Now, the set
accessor is marked as private
. This means that external code cannot directly modify MyProperty
from outside the MyClass
scope. Only methods within the same class can set the value of MyProperty
.
Use Cases for private set
in C#
Immutable Objects:
A private set
is commonly used in creating immutable objects. Once an object is instantiated, its state cannot be changed. This enhances predictability and makes the code more robust.
public class ImmutableClass {
public int ImmutableProperty { get; private set; }
public ImmutableClass(int value) {
ImmutableProperty = value;
}
}
Internal State Management:
When a class needs to manage its internal state without exposing the ability for external classes to modify certain properties directly, a private set
proves useful.
public class StatefulClass {
private int internalState;
public int ExternalState {
get { return internalState; }
private
set { internalState = value; }
}
public void PerformStateChange(int newValue) {
// Some internal logic
ExternalState = newValue;
}
}
Read-Only Properties:
A private set
is instrumental in creating read-only properties, allowing external code to read the property value but preventing modifications.
public class ReadOnlyClass {
public int ReadOnlyProperty { get; private set; }
public ReadOnlyClass(int value) {
ReadOnlyProperty = value;
}
}
Advantages of Using private set
in C#
- Encapsulation: A
private set
enhances encapsulation by restricting access to the property. This helps in hiding implementation details and preventing unintended modifications. - Immutable Objects: As mentioned earlier, a
private set
facilitates the creation of immutable objects, which can be advantageous in multithreaded environments and for ensuring data consistency. - Code Security: By limiting write access to properties,
private set
adds an extra layer of security, reducing the risk of unintended side effects caused by external modifications.
Potential Pitfalls and Considerations
- Limited Accessibility: While restricting write access is beneficial in many scenarios, it’s essential to carefully consider whether external classes genuinely need access to modify the property.
- Maintainability: Overuse of private sets can lead to less maintainable code if not applied judiciously. It’s crucial to strike a balance between encapsulation and flexibility.
- Initialization Challenges: When using a
private set
in combination with constructors or initialization methods, ensure proper validation and handling to avoid inconsistent or invalid states.
Conclusion
C# private set
is a powerful feature that enhances the control and security of your code by restricting write access to properties. By carefully applying private sets
in the appropriate scenarios, developers can create more robust, encapsulated, and maintainable code.
Whether it’s for building immutable objects, managing internal state, or creating read-only properties, a private set
is a valuable tool in the C# developer’s toolbox. Understanding its nuances and leveraging its capabilities can lead to cleaner, more secure, and more efficient code.
Harshit Jindal has done his Bachelors in Computer Science Engineering(2021) from DTU. He has always been a problem solver and now turned that into his profession. Currently working at M365 Cloud Security team(Torus) on Cloud Security Services and Datacenter Buildout Automation.
LinkedIn