How to Check Prime Number in C#
-
User Input to Check Prime Numbers in a Given Range in
C#
-
Use
isPrime
Boolean to Check Prime Number inC#
-
Use Recursion to Check Prime Number in
C#
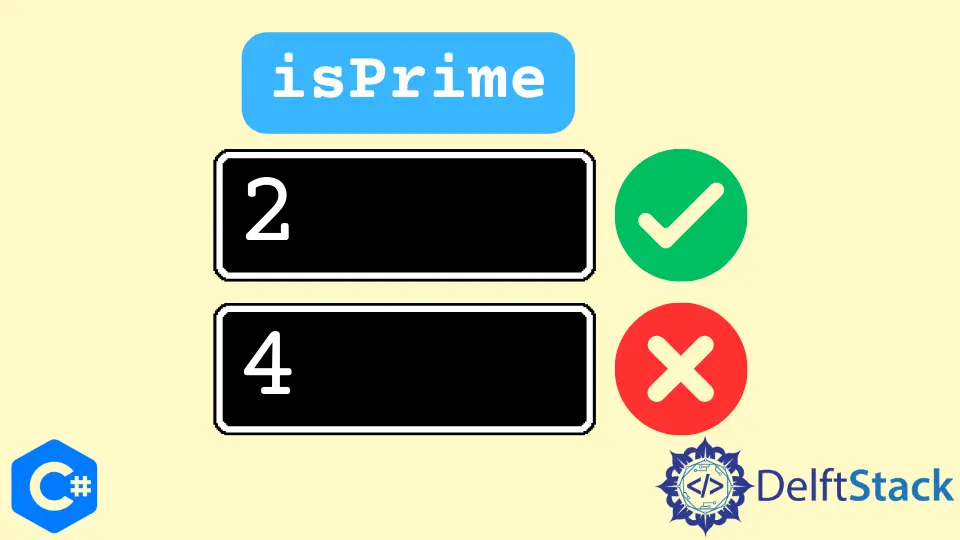
In programming, writing algorithms to find positive integers greater than 1
which do not have any other factors except 1
or itself gives us the prime numbers. This tutorial will teach you three solutions to check prime numbers in C#.
User Input to Check Prime Numbers in a Given Range in C#
In C#, the most efficient way to find prime numbers in a given range is by writing an algorithm using for
loops and if
conditions. The following C# program can help you understand algorithms to find prime numbers in a given range.
Code Example
using System;
class PrimeNumber {
static void checkPrime(int InputN) {
int n = 0;
// algorithm to check prime number
for (int i = 2; i < (InputN / 2 + 1); i++) {
if (InputN % i == 0) {
n++;
break;
}
}
if (n == 0) {
Console.Write(InputN + " ");
}
}
static void Main(string[] args) {
Console.WriteLine("Enter a number to check prime numbers in the given range: ");
string iN = Console.ReadLine();
int iNum = Convert.ToInt32(iN);
Console.WriteLine("Prime numbers less than " + iNum + " are: ");
// loop for finding every prime number in the given range
for (int i = 2; i < iNum + 1; i++) {
checkPrime(i);
}
}
}
Output:
Enter a number to check prime numbers in the given range:
30
Prime numbers less than 30 are:
2 3 5 7 11 13 17 19 23 29
Use isPrime
Boolean to Check Prime Number in C#
Use the isPrime
Boolean to check whether the user input number is a prime number or not. In the case of a prime number, the value of isPrime
will be true
otherwise false
.
It’s called trial division and consists of a for
loop and if-else
condition.
Code Example:
using System;
public class PrimeNumber {
public static void Main(string[] args) {
Console.WriteLine("Enter a number: ");
var n = int.Parse(Console.ReadLine());
bool isPrime = true;
for (var i = 2; i <= Math.Sqrt(n); i++) {
if (n % i == 0) {
isPrime = false;
break;
}
}
if (isPrime == true) {
Console.WriteLine("It's a prime number.");
} else {
Console.WriteLine("It's not prime number");
}
}
}
Output:
Enter a number: 19
It's a prime number.
The for
loop defines an integer n
as a prime number if n > 1
and n
is not divisible by i
. The for
loop output will either be a composite number or a prime number.
An if
condition will further check if n
is divisible or not by any other number. If it is divisible, the value of isPrime
will be false
; otherwise, true
.
After executing the for
loop, the number will be prime if the value of isPrime
is true
.
Use Recursion to Check Prime Number in C#
It is a native solution to find a prime number in C#. A C# algorithm checks if a number between 2
to n - 1
divides n
.
If it finds any number that divides, it will return false
meaning n
as a user-defined number is not a prime number.
Code Example:
using System;
class CheckPrime {
static int i = 2;
// checks if a number is prime
static bool isPrime(int n) {
// check this number
// you can modify this number or make the user set its value thorugh `Console.Readline();`
n = 7;
// corner cases
if (n == 0 || n == 1) {
return false;
}
// Checking Prime
if (n == i)
return true;
// base cases
if (n % i == 0) {
return false;
}
i++;
return isPrime(n);
}
static void Main() {
if (isPrime(35)) {
Console.WriteLine("It is a prime number.");
} else {
Console.WriteLine("It is not a prime number.");
}
}
}
Output:
It is a prime number.
Hassan is a Software Engineer with a well-developed set of programming skills. He uses his knowledge and writing capabilities to produce interesting-to-read technical articles.
GitHub