How to Calculate MD5 Hash From a String in C#
-
Using the
MD5
Algorithm inC#
-
Use the
System.Security.Cryptography
Library to Calculate MD5 Hash From a C# String
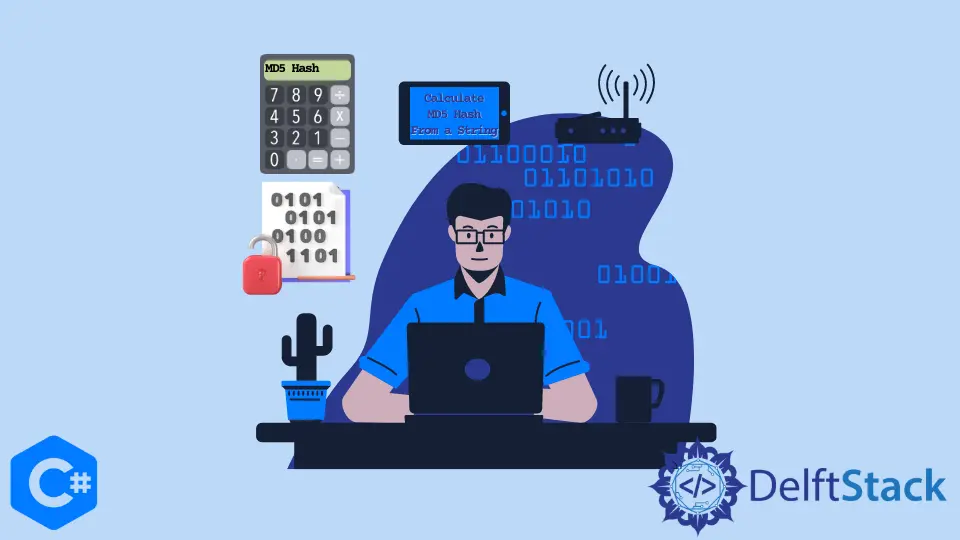
The Hashing algorithms
are mathematical algorithms used in mapping arbitrary input data to a fixed size hash. They are used for multiple purposes like storing data in data structures like hash maps
, password verification
, message digestion
, and cryptocurrency security
.
The MD5
belongs to the message digesting class of hashing algorithms. Rivest developed MD5
and other algorithms like MD2
, MD4
, etc.
The algorithm was originally designed to authenticate digital signatures. But later, many vulnerabilities were discovered that led to its deprecation.
Nevertheless, it is still used for data integrity verification and forms a foundation for understanding advanced algorithms. MD5
algorithm, like all message digestion algorithms, takes as input a message of unknown length and converts it into a fixed-size hash.
A minor change into the input string will result in a completely different hash. This article will learn how to calculate the MD5
hash using C#
.
Using the MD5
Algorithm in C#
The MD5 algorithm consists of the following four main steps:
First, append padding bits.
In this step, we add padding bits so that the number of total bits becomes 64
less than the closest multiple of 512
. It is done so that the algorithm can process data in the size of 512
bits and step 2
, the length of string expressed in 64
bits is added to get an even multiple of 512
.
Second, append length bits.
Calculate the length of the string in 64
bits. Append them to the output formed in step 1
.
Third, initialize MD buffer.
We initialize 4
message digesting buffers of 32
bits named A
, B
, C
, and D
. They are as follows:
A = 01 23 45 67
B = 89 ab cd ef
C = fe dc ba 98
D = 76 54 32 10
Fourth is the Processing of individual 512
-bit blocks.
The whole bits are broken into blocks of size 512
. Each block formed is divided into 16
sub-blocks of 32
bits. All the 16
blocks formed are passed through a series of 4
operations with the buffers A
, B
, C
, and D
to give the final hash.
Use the System.Security.Cryptography
Library to Calculate MD5 Hash From a C# String
using System;
using System.Security.Cryptography;
using System.Text;
public class Test {
public static string ComputeMd5Hash(string message) {
using (MD5 md5 = MD5.Create()) {
byte[] input = Encoding.ASCII.GetBytes(message);
byte[] hash = md5.ComputeHash(input);
StringBuilder sb = new StringBuilder();
for (int i = 0; i < hash.Length; i++) {
sb.Append(hash[i].ToString("X2"));
}
return sb.ToString();
}
}
public static void Main() {
string message = "Welcome to DelfStack";
Console.WriteLine(ComputeMd5Hash(message));
}
}
Output:
53C62733BB54F2B720A32490E6C447FF
In the above program, we create an object of the MD5
class present in C#
that provides a readymade implementation of the md5
algorithm through the method ComputeHash()
.
We convert our message string into a byte stream
to match the function signature of the ComputeHash()
method, which then returns the result in the form of a byte stream
. We then use the string builder class to convert the byte stream into a valid C#
string.
Harshit Jindal has done his Bachelors in Computer Science Engineering(2021) from DTU. He has always been a problem solver and now turned that into his profession. Currently working at M365 Cloud Security team(Torus) on Cloud Security Services and Datacenter Buildout Automation.
LinkedIn