How to Implement a Property in an Interface in C#
- Use a Simple Code Block to Set Properties in an Interface
-
Modifications to Properties in an Interface in
C#
- Implement Property with Multiple Interface Inheritance in an Abstract Class
- Conclusion
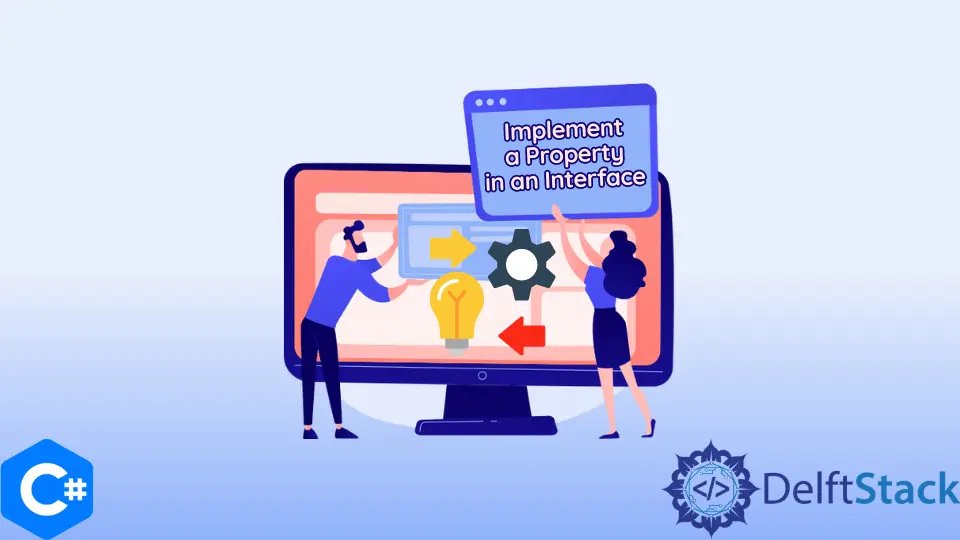
Today, we will be learning how to implement properties in an interface in C#.
Interfaces in C# can have many different properties, along with the access modifiers specifying how we should declare property availability within. The interface often acts as a default implementation of different members and objects.
Let’s begin and see the various ways through which we can implement properties in an interface.
Use a Simple Code Block to Set Properties in an Interface
Let’s suppose that we create an interface in C# called ENGINE
with the TORQUE
property. We’ll set the interface ENGINE
as follows:
public interface ENGINE {
int torque { get; set; }
}
Hence, we have created an interface with a proper getter and setter for its property called TORQUE
. To use the interface, we have to go ahead and define a class that can implement this interface.
Let’s suppose we want to define a CAR
class that contains an ENGINE
. Hence we can write something as follows:
class CAR : ENGINE {
public int torque { get; set; }
}
Do notice that the CAR
class contains an implementation of TORQUE
for public use when creating an object from this class. To use the CAR
object, simply call something as follows:
CAR x = new CAR();
x.torque = 989;
Console.WriteLine(x.torque);
Hence, you can use different properties and include them in the interface to be used later. This provides a better layer of abstraction and allows for modifications without changing the main methods or classes.
So if we wanted to add a new property called LABEL
to ENGINE
, we could set it in the interface ENGINE
, which can be used in the class CAR
.
The whole code would look as follows:
using System;
namespace CA_ONE {
class Program {
public interface ENGINE {
int torque { get; set; }
}
class CAR : ENGINE {
public int torque { get; set; }
}
static void Main(string[] args) {
CAR x = new CAR();
x.torque = 989;
Console.WriteLine(x.torque);
}
}
}
We have named our project CA_ONE
; hence the namespace extends and implements it. One more thing to notice here is that the implementation of TORQUE
in CAR
can have other definitions inside its function, such as a PRINT
method or a torque conversion, etc.
Modifications to Properties in an Interface in C#
In the solution provided above, we mentioned how we could define other methods inside our implementation. Let’s suppose we want to extend the GET
and SET
methods defined as default in the interface ENGINE
.
We will leave ENGINE
untouched but tend to modify the code inside the class CAR
that extends it.
In CAR
, when we set a TORQUE
, we may want also to print "Torque is Set to _value_"
for our user to know if the TORQUE
property has been set properly or not.
We can, hence, go ahead and do something as follows:
class CAR : ENGINE {
private int torque_priv;
public int torque {
get { return this.torque_priv; }
set {
this.torque_priv = value;
Console.WriteLine("This torque has been set to " + torque_priv);
}
}
}
Now here, let’s focus on what’s happening in the code. The first thing that we notice is the use of another variable called TORQUE_PRIV
.
We have instantiated it because we need to store the TORQUE
value passed in the following code:
x.torque = 989;
If rather than this, you do not use the TORQUE_PRIV
variable, the code will get stuck in a loop, moving recursively to assign the value to the TORQUE
property. Also, we have used something called VALUE
here.
Notice that VALUE
is not a variable but rather a contextual keyword inside C#. VALUE
is used in the set indexer in property and access declarations.
It takes the value assigned by the client and assigns it to the private variable of the class. In this manner, you can modify and use your declarations.
Many people will use the following code in the setter of TORQUE
for abstract CAR
.
this.torque_priv = torque;
And for the GETTER:
return torque;
This will present an error as follows due to getting stuck in a recursive loop:
Stack overflow.
The general syntax for implementing any property in an interface goes as follows:
type name { get; set; }
Because multiple classes can inherit and use interfaces, extending and modifying properties in the abstract classes is better. Interfaces can serve as the backbone structure when implementing different methods and provide a basic idea of what and what not to extend.
Remember that interfaces cannot be used straightforwardly. They need to have abstract classes initialized and used while running a code.
Methods in an interface are always public, and there is no need for any implicit access modifier. Also, interfaces can inherit from other interfaces.
If you are trying to implement a property in an interface, and another interface inherits the one defined previously, you can use a class to extend the latter interface.
Let’s suppose that we have an interface ENGINE
that inherits BOLTS
and then is inherited by the class CAR
. We want to install an emergency kit with the wrench size according to the bolt dimensions for each CAR
.
Hence, we can use an implementation as follows:
public interface BOLT {
int size { get; set; }
}
public interface ENGINE : BOLT {
int torque { get; set; }
And then extending the BOLT
in the class CAR
as follows:
public int size {
get { return wrench_size; }
set {
// set the wrench size
wrench_size = value;
Console.WriteLine("The wrench size to use should be: " + wrench_size);
}
}
So you can see that whatever interfaces are inherited. If you set a property in a base interface, you will need to implement that property in all classes that extend either the base interface or the derived interfaces.
Hence it is necessary to extend the definitions.
Implement Property with Multiple Interface Inheritance in an Abstract Class
Let’s say that we have two interfaces: ENGINE
and WHEEL
. And both of them contain a start method, telling us if the ENGINE
or WHEEL
has started or not.
Let’s define a start method.
public interface WHEEL {
void print();
}
public interface ENGINE : BOLT {
int torque { get; set; }
void print();
}
To extend it in the CAR
class, you need to write some functions as follows:
void WHEEL.print() {
Console.WriteLine("Wheel has been started");
}
void ENGINE.print() {
Console.WriteLine("Engine has been started!");
}
And to call them, write something as follows in the main:
((WHEEL)x).print();
This is to specify which interface you want to use the print()
method.
The complete code is below.
using System;
namespace CA_ONE {
class Program {
public interface BOLT {
int size { get; set; }
}
public interface WHEEL {
void print();
}
public interface ENGINE : BOLT {
int torque { get; set; }
void print();
}
class CAR : ENGINE, WHEEL {
private int torque_priv;
private int wrench_size;
public int torque {
get { return torque_priv; }
set {
this.torque_priv = value;
Console.WriteLine("This torque has been set to " + torque_priv);
}
}
public int size {
get { return wrench_size; }
set {
// set the wrench size
wrench_size = value;
Console.WriteLine("The wrench size to use should be: " + wrench_size);
}
}
void WHEEL.print() {
Console.WriteLine("Wheel has been started");
}
void ENGINE.print() {
Console.WriteLine("Engine has been started!");
}
}
static void Main(string[] args) {
CAR x = new CAR();
x.torque = 989;
x.size = 90;
((WHEEL)x).print();
Console.WriteLine(x.torque + " and the wrench is: " + x.size);
}
}
}
Access the complete code used in this tutorial here.
Conclusion
That is all for how to use properties in interfaces. There may be different circumstances in which we need to set and use a property; hence, it is better to study all cases described above.
Hello, I am Bilal, a research enthusiast who tends to break and make code from scratch. I dwell deep into the latest issues faced by the developer community and provide answers and different solutions. Apart from that, I am just another normal developer with a laptop, a mug of coffee, some biscuits and a thick spectacle!
GitHub