How to Get Domain Name in C#
-
Use the
ManagementObjectSearcher
Class to Get the Domain Name inC#
-
Use the
System.Net.NetworkInformation
Namespace to Get the Domain Name inC#
-
Use
System.Environment.UserDomainName
to Get the Domain Name inC#
-
Use
System.DirectoryServices.ActiveDirectory
to Get the Domain Name inC#
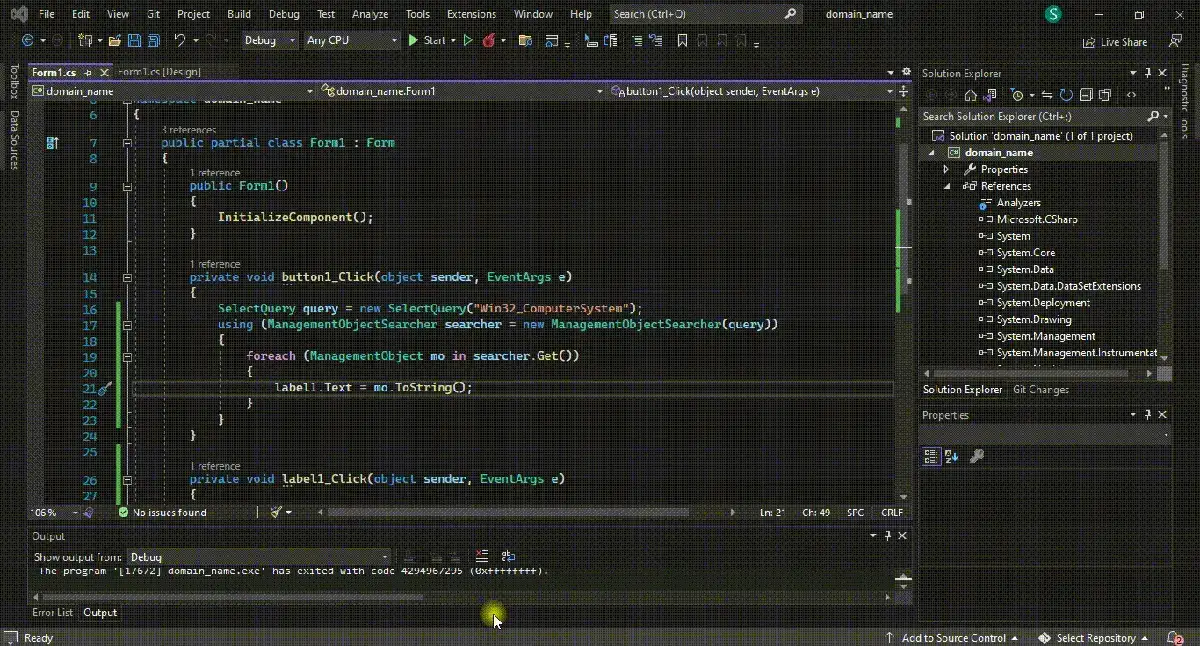
This tutorial explains the different types of domain names and shows you how to get them for a specific user in C#. A domain name can be a user/computer security principal of the Active Directory domain, the network’s stack primary DNS, or a DNS name that resolves a computer/user IP address on a network.
There are four primary ways in C# to get these domain names and choose one that fulfills your requirements and is highly dependent on your tasks. If you are looking for the computer’s Active Directory domain, you want to get (or access) the domain to which the computer’s security principal and user’s principal belong.
In general, you want this information to make a stable connection with the Active Directory; remember, the user’s and computer’s principal are not necessarily in the same domain. On the other hand, the local network stack’s primary DNS suffix can also be a domain name you want to get, and in the majority of cases, accessing or getting it is probably the same as the domain to which both the computer’s security principal and the currently authenticated user’s principal belongs.
You may want the local network’s stack primary DNS suffix as a domain name to connect successfully over LAN, even though devices have to do anything with the Active Directory.
The last option, if you are interested in the user IP address as the domain name, which can be anywhere between zero and infinity DNS records - and it can be HTTP_HOST
to send the request you are currently processing; it also doesn’t have to do anything with Microsoft’s Active Directory.
Use the ManagementObjectSearcher
Class to Get the Domain Name in C#
It belongs to the System.Management
namespace and helps users retrieve management objects (a domain name is a management object) based on a specific query. It’s easy to use and perfect for C# beginners to retrieve management information or domain name.
You can enumerate management information on disk drives, network adapters, processes, system information, network connections, paused services, etc. It uses WMI queries (represented in ObjectQuery
) as an input when instantiated and a ManagementScope
representing the WMI namespace to execute the user queries.
using System;
using System.Management; // essential namespace
using System.Windows.Forms;
namespace domain_name {
public partial class Form1 : Form {
public Form1() {
InitializeComponent();
}
private void button1_Click(object sender, EventArgs e) {
SelectQuery query = new SelectQuery("Win32_ComputerSystem");
using (ManagementObjectSearcher searcher = new ManagementObjectSearcher(query)) {
foreach (ManagementObject mo in searcher.Get()) {
label1.Text = mo.ToString();
}
}
}
private void label1_Click(object sender, EventArgs e) {}
}
}
Output:
C# veterans and experienced developers can also take advantage of its additional advanced options in an EnumerationOptions
. Understanding the ManagementObjectSearcher
class is easy; it executes the given query in the specified scope and returns the domain name that matches the query in a ManagementObjectCollection
when the Get()
method on the class object is invoked.
It’s a perfect example of getting the domain name dynamically when the computer’s in Active Directory for the administrator. Use SelectQuery
to create a query and assign it to the class object and apply the Get()
method on that object to get the domain name.
Use the System.Net.NetworkInformation
Namespace to Get the Domain Name in C#
You can use System.Net.NetworkInformation.IPGlobalProperties.GetIPGlobalProperties().DomainName
to get the network stack’s primary DNS suffix as the domain name and to get the DNS name that resolves computer/user IP address.
The IPGlobalProperties.DomainName
property belongs to the System.Net.NetworkInformation
namespace and can get you the domain name in which the local computer is registered.
The IPGlobalProperties
object contains the domain information (system-supplied type) about the local computer, and the System.Net.NetworkInformation
namespace gives you access to your network traffic data and network address information.
However, you can use the NetworkInterface
class if you want information about your network interface on the local computer.
using System;
using System.Net.NetworkInformation; // essential namespace
using System.Windows.Forms;
namespace domain_name {
public partial class Form1 : Form {
public Form1() {
InitializeComponent();
}
private void button1_Click(object sender, EventArgs e) {
IPGlobalProperties properties = IPGlobalProperties.GetIPGlobalProperties();
string domain_name = properties.DomainName.ToString();
label1.Text = domain_name;
}
private void label1_Click(object sender, EventArgs e) {}
}
}
Output:
Use System.Environment.UserDomainName
to Get the Domain Name in C#
As a part of the System
namespace, the Environment.UserDomainName
property gets you the network domain name or the domain of the security principal associated with the current user.
You can face issues retrieving your domain name from the server, local network, or your computer if the OS does not support retrieving the domain information or the network domain name is inaccessible.
The user domain name or domain account credentials are formatted as the \
character and a user name. You can obtain the user’s domain name without the user name with the help of the UserDomainName
property and the user name without the domain name with the help of the UserName
property.
using System;
using System.Windows.Forms;
namespace domain_name {
public partial class Form1 : Form {
public Form1() {
InitializeComponent();
}
private void button1_Click(object sender, EventArgs e) {
label1.Text = Environment.UserDomainName;
}
private void label1_Click(object sender, EventArgs e) {}
}
}
Output:
The primary purpose of the UserDomainName
property is to get you the domain name of your Windows account name for the current user (referring to you).
In case the attempt to get the domain name via this property fails, it can automatically try to retrieve/get the domain name associated with the user mentioned in the UserName
property.
Use System.DirectoryServices.ActiveDirectory
to Get the Domain Name in C#
You can use the System.DirectoryServices.ActiveDirectory.Domain.GetComputerDomain()
method to get the domain of the current computer’s security principle, which belongs to the Active Directory.
If your local computer or machine is not a part of the domain you are trying to retrieve information from or the domain controller is out of contact, it throws an ActiveDirectoryObjectNotFoundException
exception.
The GetComputerDomain
method belongs to the Domain
class, which is a part of the System.DirectoryServices.ActiveDirectory
namespace. You can use the Domain.GetComputerDomain
method to get the domain name in C# by providing a reference to its namespace.
It returns a Domain
object representing the domain name and is independent of the domain credentials under which the application is running.
using System;
using System.DirectoryServices;
using System.DirectoryServices.ActiveDirectory;
using System.Windows.Forms;
namespace domain_name {
public partial class Form1 : Form {
public Form1() {
InitializeComponent();
}
private void button1_Click(object sender, EventArgs e) {
label1.Text = Domain.GetComputerDomain().ToString();
}
private void label1_Click(object sender, EventArgs e) {}
}
}
Output:
Regardless of the trusted or authorized account domain credentials, you can use the GetCurrentDomain
method to easily retrieve the computer’s domain name. You can access a high-level abstraction domain’s object model to get the domain information as part of your Microsoft Active Directory services.
This tutorial taught you how to get the domain name in C#. It can be an AD domain name, your network domain name, your local computer’s domain name, or any other that you can get with the mentioned approaches while maintaining the performance of your C# application.
Hassan is a Software Engineer with a well-developed set of programming skills. He uses his knowledge and writing capabilities to produce interesting-to-read technical articles.
GitHub