How to Upload a File to FTP in C#
- Understanding FTP and Its Importance
- Setting Up Your C# Environment
- Method 1: Uploading a File Using FtpWebRequest
- Method 2: Using WebClient for Simplicity
- Conclusion
- FAQ
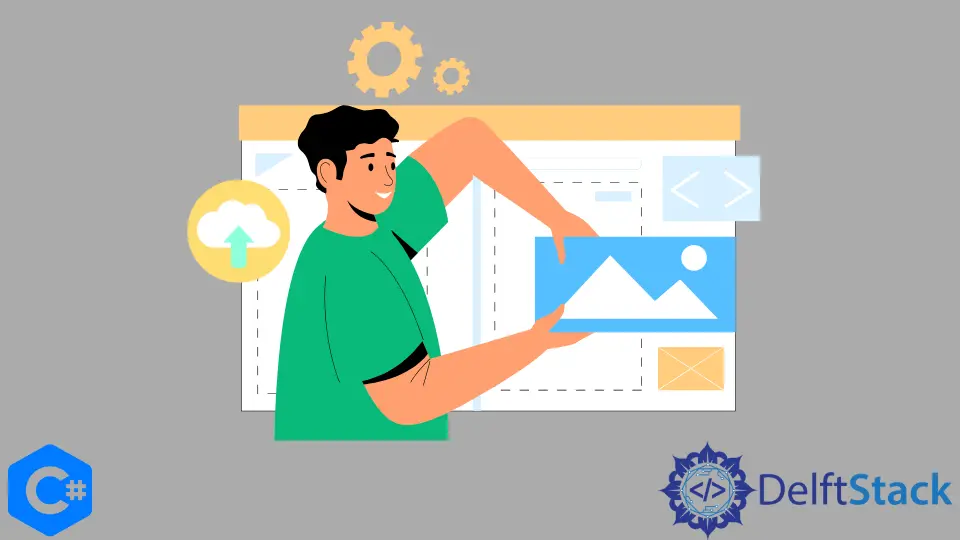
Uploading files to an FTP server using C# can be a straightforward task, especially with the right guidance. Whether you’re developing a web application, automating file transfers, or simply need to manage files on a remote server, understanding how to upload files to FTP in C# is essential. In this guide, we will walk you through the step-by-step process of uploading files using C#. We will cover the necessary libraries, provide clear code examples, and explain each part of the process in a way that is easy to understand. By the end of this article, you will be equipped with the knowledge you need to efficiently upload files to an FTP server using C#.
Understanding FTP and Its Importance
File Transfer Protocol (FTP) is a standard network protocol used for transferring files between a client and a server over a network. It plays a crucial role in web development, data management, and cloud storage solutions. In C#, the System.Net
namespace provides classes like FtpWebRequest
and FtpWebResponse
that facilitate FTP operations. Understanding how to use these classes effectively can help streamline your file management tasks, whether you’re working on personal projects or enterprise-level applications.
Setting Up Your C# Environment
Before we dive into the code, ensure you have a suitable development environment. You will need:
- Visual Studio or any C# IDE.
- Access to an FTP server for testing purposes.
- Basic knowledge of C# programming.
With your environment ready, let’s explore how to upload a file to an FTP server using C#.
Method 1: Uploading a File Using FtpWebRequest
One of the simplest methods to upload a file to an FTP server in C# is by using the FtpWebRequest
class. This method allows you to create a request to the FTP server, specify the file to upload, and handle the response.
Here’s a basic example of how to do this:
using System;
using System.IO;
using System.Net;
class Program
{
static void Main()
{
string ftpUrl = "ftp://example.com/yourfile.txt";
string filePath = @"C:\path\to\yourfile.txt";
string username = "yourUsername";
string password = "yourPassword";
FtpWebRequest request = (FtpWebRequest)WebRequest.Create(ftpUrl);
request.Method = WebRequestMethods.Ftp.UploadFile;
request.Credentials = new NetworkCredential(username, password);
byte[] fileContents;
using (StreamReader sourceStream = new StreamReader(filePath))
{
fileContents = new byte[sourceStream.BaseStream.Length];
sourceStream.BaseStream.Read(fileContents, 0, fileContents.Length);
}
request.ContentLength = fileContents.Length;
using (Stream requestStream = request.GetRequestStream())
{
requestStream.Write(fileContents, 0, fileContents.Length);
}
FtpWebResponse response = (FtpWebResponse)request.GetResponse();
Console.WriteLine($"Upload File Complete, status {response.StatusDescription}");
}
}
Output:
Upload File Complete, status 226
In this example, we first create an FtpWebRequest
object pointing to the desired FTP URL. We specify the method as UploadFile
, set the credentials, and read the contents of the file we want to upload. After writing the file contents to the request stream, we get the response from the server. The response will indicate whether the upload was successful, which we print to the console.
Method 2: Using WebClient for Simplicity
If you’re looking for a more straightforward approach, you can use the WebClient
class, which simplifies the process of uploading files to an FTP server. This method is less verbose and can be easier to implement for smaller applications.
Here’s how you can do it:
using System;
using System.Net;
class Program
{
static void Main()
{
string ftpUrl = "ftp://example.com/yourfile.txt";
string filePath = @"C:\path\to\yourfile.txt";
string username = "yourUsername";
string password = "yourPassword";
using (WebClient client = new WebClient())
{
client.Credentials = new NetworkCredential(username, password);
client.UploadFile(ftpUrl, WebRequestMethods.Ftp.UploadFile, filePath);
Console.WriteLine("Upload Complete");
}
}
}
Output:
Upload Complete
In this example, we create an instance of WebClient
, set the credentials, and use the UploadFile
method to upload our file directly. The WebClient
class abstracts many of the complexities involved in FTP operations, making it a great choice for quick implementations. Once the upload is complete, we simply print a confirmation message to the console.
Conclusion
Uploading files to an FTP server in C# can be accomplished using various methods, with FtpWebRequest
and WebClient
being the most common. Each method has its advantages, allowing you to choose based on your specific needs and complexity of the application. By mastering these techniques, you can effectively manage file transfers in your applications, enhancing their functionality and user experience.
With this guide, you now have the tools and knowledge to upload files to an FTP server using C#. Whether you’re automating processes or developing applications, these skills will serve you well in your programming journey.
FAQ
-
What is FTP and why is it used?
FTP is a protocol used to transfer files between a client and a server over a network, commonly used for managing files on web servers. -
Can I upload multiple files at once using C#?
Yes, you can iterate through a list of files and call the upload method for each file. -
What do I do if my FTP upload fails?
Check your FTP credentials, ensure the FTP server is reachable, and verify the file path and permissions. -
Is there a limit to the file size I can upload?
The file size limit depends on the FTP server configuration; check with your server administrator for specifics. -
Can I use FTP over SSL/TLS in C#?
Yes, you can use FTPS by setting theEnableSsl
property of theFtpWebRequest
totrue
.
with step-by-step instructions and code examples. We cover methods like FtpWebRequest and WebClient, helping you efficiently manage file transfers. Whether you’re a beginner or an experienced developer, this article provides the insights you need to handle FTP uploads seamlessly.
Haider specializes in technical writing. He has a solid background in computer science that allows him to create engaging, original, and compelling technical tutorials. In his free time, he enjoys adding new skills to his repertoire and watching Netflix.
LinkedIn