Exponent in C#
- Understanding the Math.Pow() Method
- Using Math.Pow() for Different Scenarios
- Handling Edge Cases with Math.Pow()
- Conclusion
- FAQ
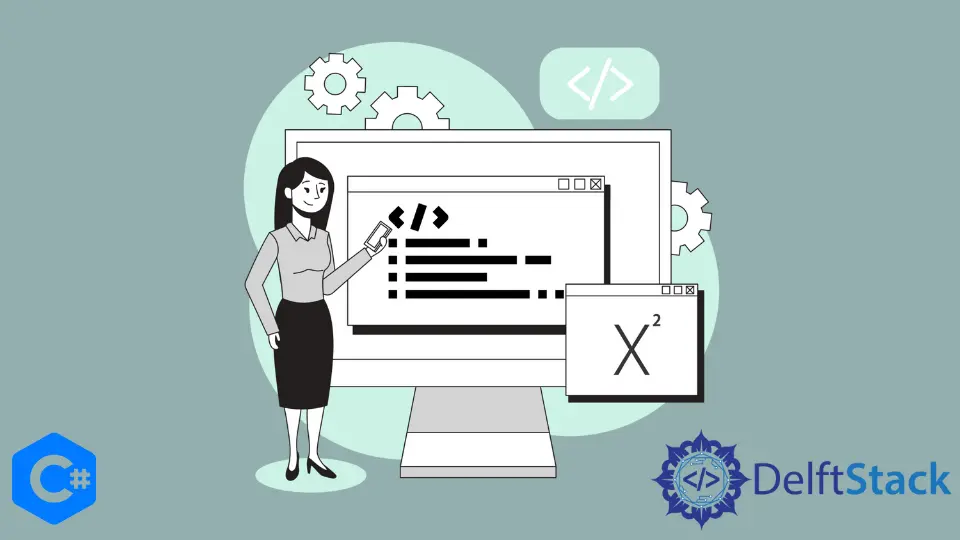
When it comes to mathematical operations in programming, calculating exponents is a fundamental task. In C#, the exponentiation operation can be easily performed using the Math.Pow()
method. This powerful function allows developers to raise a number to a specific power, making it an essential tool for a variety of applications, from simple calculations to complex algorithms.
In this article, we will dive into the exponent operator in C#, explore the Math.Pow()
method in detail, and provide clear code examples to help you understand its usage. Whether you’re a beginner or an experienced developer, you’ll find valuable insights that will enhance your coding skills.
Understanding the Math.Pow() Method
The Math.Pow()
method is a built-in function in C# that takes two arguments: the base and the exponent. It returns the result of raising the base to the power of the exponent. The syntax is straightforward:
double result = Math.Pow(base, exponent);
Here, base
is the number you want to raise, and exponent
is the power to which you want to raise the base. The result is returned as a double
, which allows for fractional values.
Let’s take a look at a simple example:
using System;
class Program
{
static void Main()
{
double baseNumber = 2;
double exponentNumber = 3;
double result = Math.Pow(baseNumber, exponentNumber);
Console.WriteLine(result);
}
}
Output:
8
In this example, we raised 2 to the power of 3, resulting in 8. The Math.Pow()
method simplifies exponentiation, making it easy to perform complex calculations without getting bogged down in the math.
Using Math.Pow() for Different Scenarios
The Math.Pow()
method can be applied in various scenarios, from financial calculations to scientific computations. For instance, you might want to calculate compound interest, where the formula involves exponentiation. Let’s see how we can implement this in C#:
using System;
class Program
{
static void Main()
{
double principal = 1000; // initial amount
double rate = 0.05; // interest rate
int years = 10; // number of years
double amount = principal * Math.Pow(1 + rate, years);
Console.WriteLine(amount);
}
}
Output:
1628.894626777442
In this example, we calculated the future value of an investment using the compound interest formula. The Math.Pow()
method allows us to apply the exponentiation required to compute the total amount after 10 years at an interest rate of 5%. This demonstrates the versatility of the Math.Pow()
method in real-world applications.
Handling Edge Cases with Math.Pow()
While Math.Pow()
is powerful, it’s essential to understand how it handles edge cases. For example, raising a number to the power of zero always results in one, regardless of the base. Additionally, raising zero to any positive power also yields zero. Let’s explore these scenarios:
using System;
class Program
{
static void Main()
{
Console.WriteLine(Math.Pow(0, 5)); // Zero raised to a power
Console.WriteLine(Math.Pow(5, 0)); // Any number raised to zero
Console.WriteLine(Math.Pow(2, -3)); // Negative exponent
}
}
Output:
0
1
0.125
In this code, we demonstrated how Math.Pow()
handles zero and negative exponents. The output shows that zero raised to any positive power is zero, while any non-zero number raised to the power of zero equals one. Finally, a negative exponent returns the reciprocal of the base raised to the absolute value of the exponent, showcasing the method’s flexibility.
Conclusion
The Math.Pow()
method in C# is an invaluable tool for performing exponentiation with ease and precision. Whether you’re calculating compound interest, working with scientific data, or simply performing basic math, this method can simplify your coding experience. By understanding how to use Math.Pow()
, you can enhance your programming skills and tackle a wide range of mathematical challenges effectively. With the examples and explanations provided, you should now feel confident in applying the exponent operator in your own C# projects.
FAQ
-
What is the purpose of the Math.Pow() method in C#?
The Math.Pow() method is used to raise a number (base) to a specified power (exponent) and returns the result as a double. -
Can I use Math.Pow() for negative exponents?
Yes, Math.Pow() can handle negative exponents by returning the reciprocal of the base raised to the absolute value of the exponent. -
What data type does Math.Pow() return?
The Math.Pow() method returns a value of type double. -
How do I calculate square roots in C#?
You can calculate square roots by using Math.Pow() with an exponent of 0.5, or simply use Math.Sqrt() method for simplicity. -
Are there performance considerations when using Math.Pow()?
While Math.Pow() is efficient for most applications, for simple cases like squaring a number, using multiplication may be faster.