Double Question Mark in C#
- What is the Double Question Mark Operator?
- How to Use the Double Question Mark Operator
- Chaining the Double Question Mark Operator
- Practical Applications of the Double Question Mark Operator
- Conclusion
- FAQ
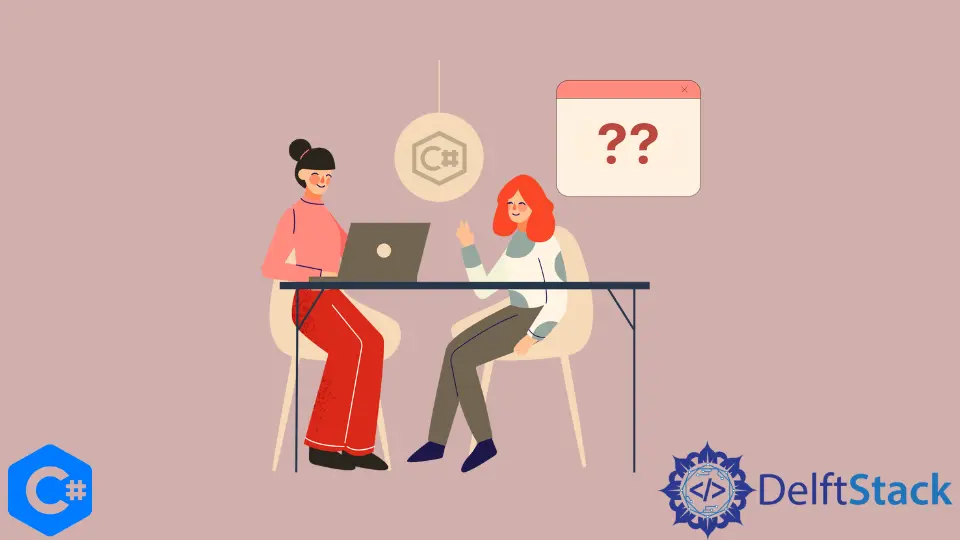
In the world of C#, handling null values is a common challenge that developers face. To streamline this process, C# introduced the double question mark operator, also known as the null coalescing operator. This powerful feature allows developers to provide default values for nullable expressions, enhancing code readability and robustness. If you’ve ever found yourself tangled in null checks and conditional statements, the double question mark operator might just be the solution you need.
In this article, we’ll dive deep into what the double question mark means in C#, how it works, and why it’s a valuable addition to your coding toolkit.
What is the Double Question Mark Operator?
The double question mark operator (??
) is a concise way to handle null values in C#. It allows you to specify a default value that will be used when the left-hand operand is null. This operator simplifies code and reduces the need for lengthy null checks, making your code cleaner and easier to maintain.
Here’s a simple example to illustrate the concept:
string userInput = null;
string defaultInput = "Default Value";
string result = userInput ?? defaultInput;
Console.WriteLine(result);
In this example, userInput
is null, so the result
will be assigned the value of defaultInput
. The output will be:
Default Value
The beauty of the double question mark operator lies in its ability to streamline code. Instead of writing multiple lines to check if userInput
is null, you can achieve the same result in a single, elegant line. This not only saves time but also enhances code readability.
How to Use the Double Question Mark Operator
Using the double question mark operator is straightforward. You simply place it between two expressions: the first expression is evaluated, and if it is not null, its value is returned. If it is null, the second expression is returned. This makes it incredibly useful for providing fallback values.
Here’s another example to demonstrate its utility:
int? nullableInt = null;
int defaultInt = 10;
int resultInt = nullableInt ?? defaultInt;
Console.WriteLine(resultInt);
In this case, nullableInt
is a nullable integer that is null. The resultInt
will take the value of defaultInt
, which is 10. The output will be:
10
This operator is particularly handy when dealing with optional parameters or when fetching data from sources that may return null values. Instead of cluttering your code with if-else statements, you can use the double question mark operator to keep things clean and efficient.
Chaining the Double Question Mark Operator
One of the powerful features of the double question mark operator is that you can chain it to handle multiple potential null values. This allows you to provide a series of default values in a single expression, making your code even more concise.
Consider this example:
string firstValue = null;
string secondValue = null;
string thirdValue = "Final Value";
string result = firstValue ?? secondValue ?? thirdValue;
Console.WriteLine(result);
In this scenario, both firstValue
and secondValue
are null, so the result
will be assigned the value of thirdValue
. The output will be:
Final Value
Chaining the double question mark operator is a powerful technique when you have multiple potential sources for a value. It reduces the need for multiple lines of code and keeps your logic clear and concise.
Practical Applications of the Double Question Mark Operator
The double question mark operator can be particularly useful in various scenarios, such as:
- User Input Handling: When collecting user input, you can easily provide default values for optional fields.
- Database Queries: When fetching data from a database, it’s common to encounter null values. The double question mark operator allows you to handle these gracefully.
- Configuration Settings: If your application relies on configuration settings that may not always be present, you can use the operator to ensure your application has sensible defaults.
Here’s an example that combines these concepts:
string userName = GetUserInput() ?? "Guest";
Console.WriteLine($"Welcome, {userName}!");
In this case, if GetUserInput()
returns null, the user will be welcomed as “Guest.” This small addition can significantly enhance the user experience by ensuring that your application behaves predictably.
Conclusion
The double question mark operator in C# is a powerful tool for handling null values efficiently. By allowing developers to specify default values concisely, it simplifies code and improves readability. Whether you’re managing user input, dealing with optional parameters, or fetching data from a database, the null coalescing operator can save you time and effort. Embracing this feature will not only enhance your coding skills but also lead to cleaner, more maintainable code.
FAQ
-
What does the double question mark operator do in C#?
The double question mark operator (??
) provides a way to return a default value when the left-hand operand is null. -
Can I chain multiple double question mark operators?
Yes, you can chain multiple double question mark operators to handle several potential null values in a single expression. -
Is the double question mark operator the same as the ternary operator?
No, the double question mark operator specifically deals with null values, while the ternary operator is a more general conditional operator. -
When should I use the double question mark operator?
Use it when you want to provide default values for nullable expressions, especially in scenarios involving user input, database queries, or configuration settings. -
Does the double question mark operator work with value types?
Yes, it works with nullable value types, allowing you to provide default values when the nullable type is null.
#, also known as the null coalescing operator. Learn how to handle null values efficiently, improve code readability, and provide default values in your applications. Discover practical applications and examples that demonstrate the power of this feature.